Mastering REST API in JavaScript: A Comprehensive Guide
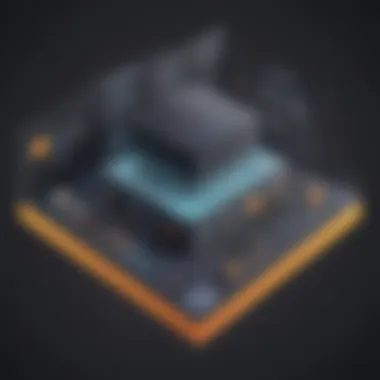
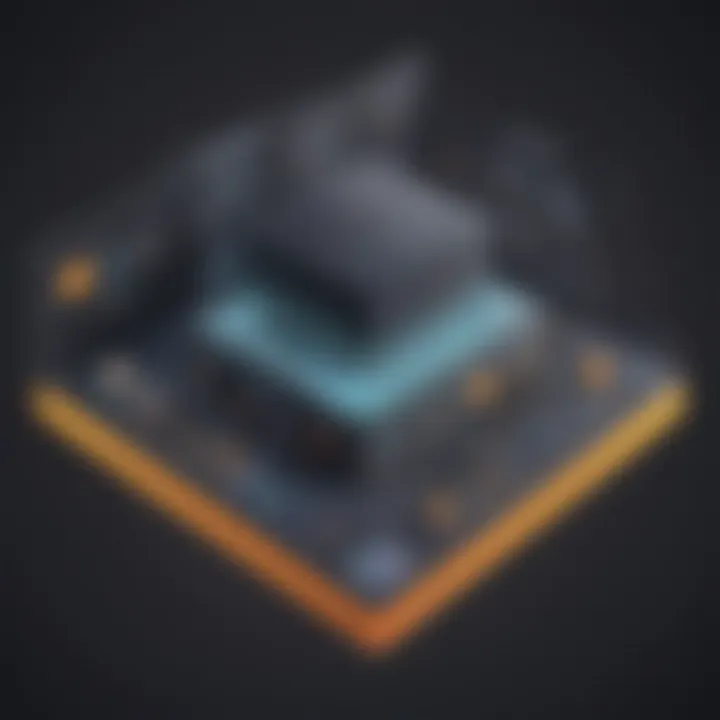
Tech Analysis: Evolution of REST APIs in JavaScript
REST architecture has revolutionized how data is exchanged on the web, particularly in JavaScript development. This section provides an overview of the current trend, highlighting the growing significance of REST APIs in modern web applications. The implications for consumers are vast, as REST APIs enable seamless integration of services and data across platforms. Looking ahead, future predictions suggest a continued rise in the adoption of REST architecture, with possibilities for enhanced scalability and flexibility in web development.
Product Overview and Performance
In mastering REST API in JavaScript, understanding the fundamental features and specifications of the technology is essential. This part offers a detailed look at the characteristics of REST APIs, emphasizing their role in facilitating communication between client and server. A performance analysis delves into the efficiency and reliability of REST API interactions, shedding light on the pros and cons of this approach. Based on performance metrics, recommendations are provided to optimize REST API usage for improved web application development.
Step-by-Step Guide to Implementing REST APIs
For tech enthusiasts eager to delve into REST API development, this guide offers a comprehensive set of instructions. Beginning with an introduction to the topic, readers are guided through the intricacies of building RESTful services in JavaScript. Step-by-step instructions outline the process of consuming and implementing REST APIs, complemented by valuable tips and tricks to enhance development efficiency. Troubleshooting strategies are also included, equipping readers with the tools to overcome common challenges in REST API integration.
Industry Insights: Transformative Impact of REST APIs
Recent developments in the tech industry have underscored the transformative impact of REST APIs on businesses and consumers. An analysis of market trends reveals the growing demand for scalable and interoperable web solutions, positioning REST architecture as a key driver of innovation. The impact on businesses is profound, as REST APIs enable seamless data exchange and integration, leading to enhanced user experiences and operational efficiency. By keeping abreast of industry updates and embracing RESTful principles, companies can stay competitive in a rapidly evolving digital landscape.
Introduction
In the realm of modern web development, mastering REST API in JavaScript is not just a skill but a necessity. REST, representing Representational State Transfer, sets the foundation for interaction between client and server systems, redefining the approach to web services. Its significance lies in its ability to simplify complex architectural constraints through a uniform interface. This introductory section paves the way for a comprehensive exploration of REST API integration using JavaScript by unraveling its core principles and practical implications.
What is REST API?
Understanding REST principles
At the core of REST API lies the adherence to certain principles that govern its design. Understanding REST principles involves grasping the concept of statelessness, where each request from the client to the server must contain all necessary information. This key characteristic ensures scalability and reliability in distributed systems, making REST a popular choice in modern web development. With its unique feature of providing a uniform interface for interaction, REST principles offer advantages in simplifying client-server communication and fostering interoperability across different platforms.
Importance of REST API in modern web development
The importance of REST API in modern web development stems from its ability to cater to the evolving needs of robust web applications. By embracing REST, developers can design scalable and efficient systems that align with industry best practices. REST API's significance lies in promoting a separation of concerns in web services, enabling modular development and enhancing system flexibility. While its advantages include simplicity and ease of integration, challenges such as handling resource representations and navigating state transitions underscore the critical role REST APIs play in shaping the digital landscape.
Fundamentals of REST
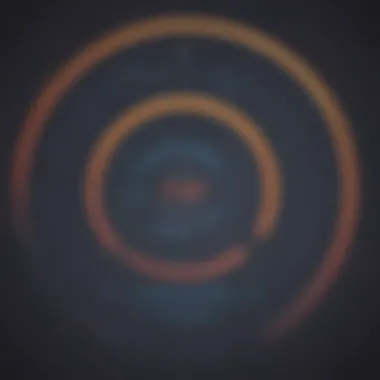
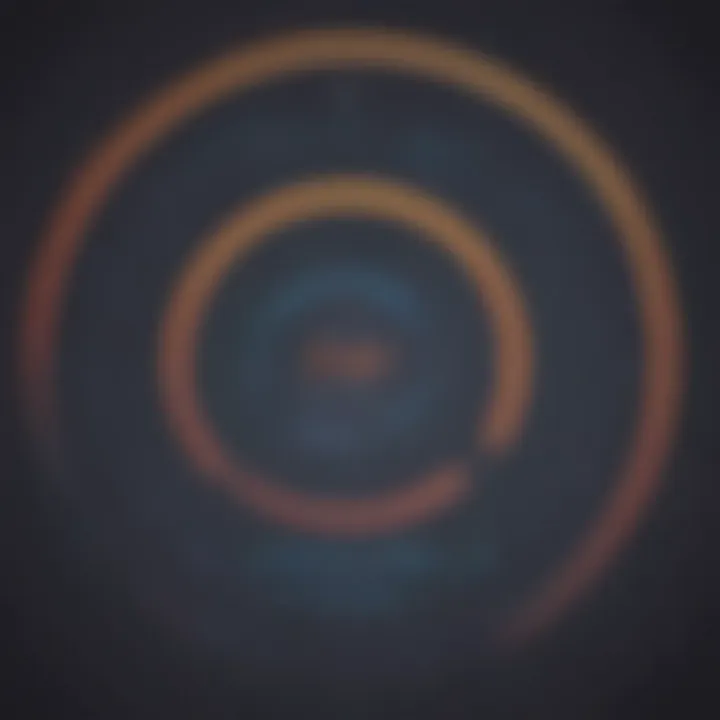
In this part of the guide, we delve into the essential aspects of REST, which form the foundation of integrating APIs using Javascript. Understanding the Fundamentals of REST is crucial for any developer aiming to excel in modern web development. REST architecture, with its emphasis on client-server interaction and statelessness, provides a robust framework for building scalable and efficient applications. By grasping the core concepts of REST, developers can streamline communication between different components of a web application, enhancing performance and user experience.
REST Architecture
Client-server interaction
Client-server interaction is a fundamental concept in REST architecture that defines how communication occurs between the client and the server. This interaction model segregates the concerns between the user interface and the data storage, allowing for better scalability and flexibility in web development. The client-server architecture enables independent evolution of the client-side and server-side components, enabling easier maintenance and updates. Although this approach introduces a certain level of complexity, the benefits of clear separation of concerns outweigh the challenges, making it a popular choice for developers seeking efficiency and modularity in their applications.
Statelessness and caching
Statelessness is a key principle in REST architecture that emphasizes each request from a client to a server should contain all the necessary information, without relying on the server's session state. This design simplifies server implementations, improves reliability, and enhances scalability as servers can handle requests independently without carrying prior context. Caching, on the other hand, boosts performance by storing server responses in the client's cache memory, reducing the need for repeated requests. While statelessness promotes better scalability and reliability, caching optimizes performance, making them invaluable concepts in building high-performing REST APIs.
HTTP Verbs in REST
GET, POST, PUT, DELETE methods
HTTP Verbs, including GET, POST, PUT, and DELETE, play a critical role in REST API operations by defining the action to be performed on a resource. The GET method retrieves data from a server, POST submits new data to be processed, PUT updates existing data, and DELETE removes specific data. These methods form the backbone of communication in RESTful applications, defining how clients interact with resources on the server. By understanding and appropriately utilizing these HTTP verbs, developers can design clear and intuitive APIs, ensuring efficient data manipulation and management.
Resourceful Routing
Defining routes for API endpoints
Resourceful Routing involves mapping HTTP methods and URLs to specific actions or controllers in an API. By defining routes for API endpoints, developers can structure their applications logically, simplifying resource access and management. This approach enhances the readability and maintainability of the codebase, promoting a more organized development process. Implementing well-designed routes streamlines API interactions, making it easier for developers to navigate and understand the functionality of each endpoint. While there may be challenges in complex routing setups, the benefits of clear resource mapping and organization justify the effort in building a cohesive and efficient REST API.
Working with REST APIs in JavaScript
Working with REST APIs in JavaScript is a crucial aspect of this comprehensive guide on mastering REST API development using JavaScript. This section focuses on the practical application of consuming and implementing RESTful services in JavaScript. Understanding how to interact with REST APIs is essential for modern web development, enabling developers to create dynamic and interactive web applications. By working with REST APIs in JavaScript, developers can harness the power of server-client communication to retrieve, update, and manipulate data effectively.
Consuming REST APIs
Making API requests with Fetch API
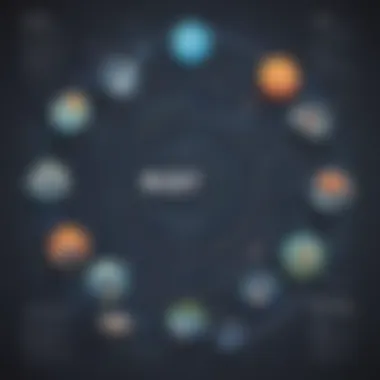
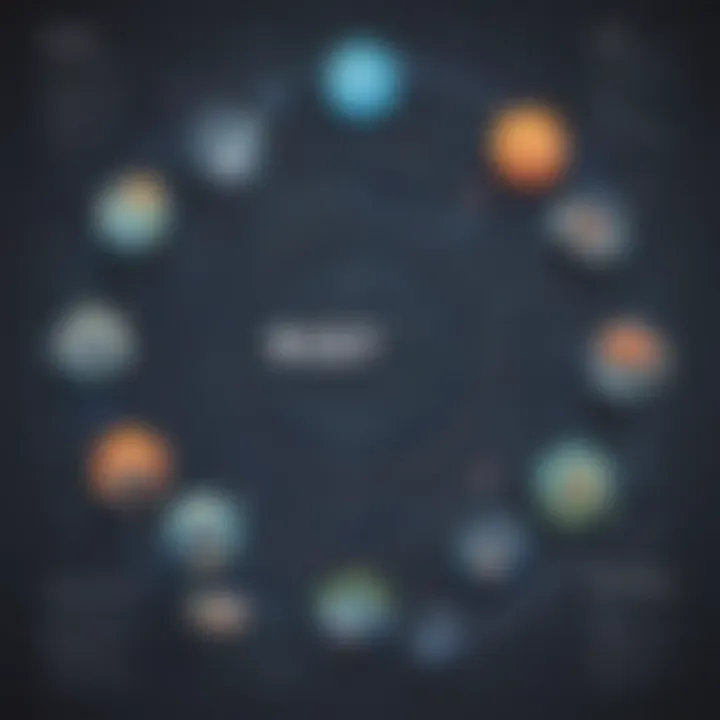
Making API requests with the Fetch API is a cornerstone of interacting with REST APIs in JavaScript. This method allows developers to initiate HTTP requests and fetch data from servers seamlessly. The Fetch API simplifies the process of handling network requests, providing a streamlined way to communicate with RESTful services. Its asynchronous nature enhances performance, making it a preferred choice for fetching data in web applications. While Fetch API offers simplicity and flexibility, it may lack some advanced features like request cancellation or timeout management, which developers should consider when working on projects.
Handling responses and error scenarios
Efficiently managing responses and handling error scenarios is critical when working with REST APIs in JavaScript. This aspect involves interpreting data returned from API calls, handling different HTTP status codes, and implementing error handling mechanisms. By effectively dealing with responses and errors, developers can ensure smooth communication between the client and server, enhancing the overall user experience. Implementing robust error-handling strategies is essential for debugging and troubleshooting issues that may arise during API interactions, leading to more resilient and reliable web applications.
Asynchronous JavaScript
Utilizing asyncawait for API calls
Utilizing the asyncawait syntax in JavaScript is a powerful technique for managing asynchronous operations like API calls. This feature simplifies the handling of asynchronous code, making it easier to write and understand asynchronous functions. By using asyncawait, developers can achieve cleaner and more readable code, improving code maintainability and reducing callback hell. This approach streamlines the process of making API calls, making the code more synchronous in appearance while preserving its asynchronous behavior. However, developers should be cautious about overusing asyncawait, as it may lead to blocking the event loop and impacting performance in certain scenarios.
Implementing RESTful Services
Creating RESTful routes in Node.js
Creating RESTful routes in Node.js is a fundamental aspect of implementing RESTful services in JavaScript. This process involves defining routes that correspond to CRUD (Create, Read, Update, Delete) operations on resources. By structuring routes following RESTful conventions, developers can design APIs that are intuitive, scalable, and easy to maintain. Node.js provides a robust environment for building RESTful services, offering various modules and frameworks that facilitate route creation and request handling. When creating RESTful routes, developers should focus on consistency, clarity, and adherence to REST principles to ensure the efficiency and maintainability of their APIs.
Integrating Express for API development
Integrating Express for API development is a popular choice among developers for building robust and efficient APIs in JavaScript. Express is a minimal and flexible Node.js web application framework that simplifies the process of creating RESTful services. With Express, developers can define routes, handle requests, and implement middleware functions to enhance the functionality of their APIs. Its lightweight nature and strong community support make Express a versatile tool for building RESTful APIs. However, developers should be mindful of security considerations and best practices while using Express to prevent vulnerabilities and ensure the reliability of their API endpoints.
Best Practices and Advanced Concepts
In the realm of REST API development, embracing best practices and advanced concepts is paramount for ensuring robust, secure, and efficient API interactions. By adhering to best practices, developers can streamline their development processes, enhance the overall performance of their APIs, and fortify security measures. Advanced concepts delve deeper into optimizing resource utilization, minimizing latency, and promoting scalability within REST APIs. These practices also encompass strategic approaches to error handling, data validation, and performance optimization, culminating in a cohesive and high-performing API ecosystem.
Security in REST APIs
Authentication and Authorization
Authentication and authorization play a pivotal role in safeguarding REST APIs against unauthorized access and malicious attacks. Authentication verifies the identity of users or applications interacting with the API, preventing unauthorized parties from compromising sensitive data or resources. Authorization, on the other hand, controls the level of access granted to authenticated entities, ensuring that users only have rights to perform specified actions. Implementing robust authentication mechanisms such as JWT tokens or OAut protocols enhances the overall security posture of REST APIs, mitigating risks associated with data breaches and unauthorized activities.
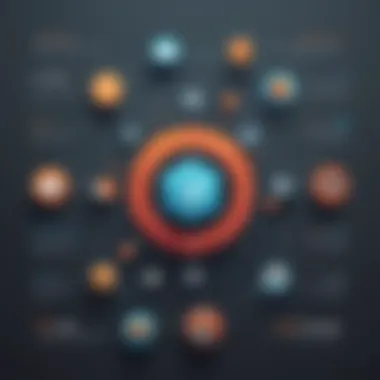
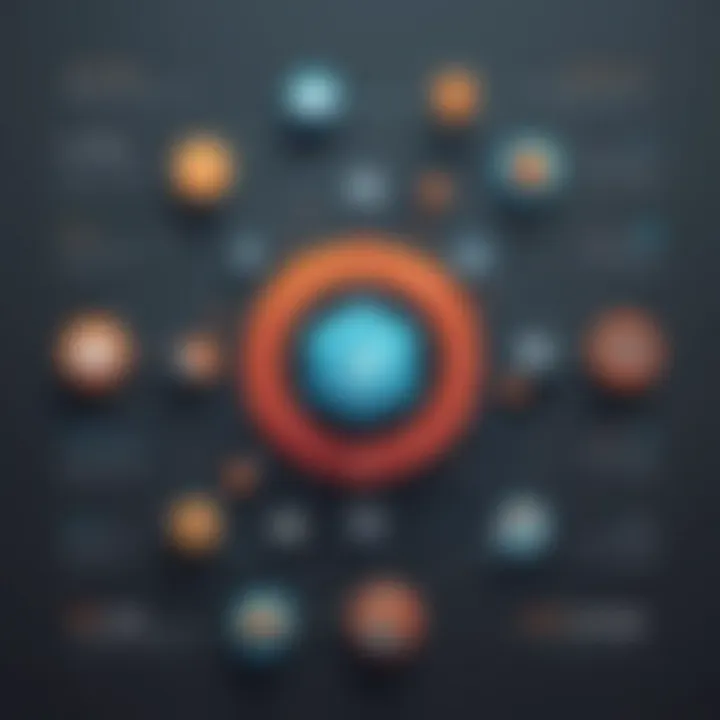
CORS Implementation
Cross-Origin Resource Sharing (CORS) implementation is integral in facilitating secure cross-origin requests within the browser environment. By enabling servers to specify who can access their resources, CORS mitigates the risks of cross-site scripting attacks and data exfiltration. CORS headers dictate the allowable origins, methods, and headers for cross-domain requests, maintaining the integrity and confidentiality of sensitive data. However, improper CORS configuration can expose APIs to security vulnerabilities such as cross-site request forgery (CSRF) or information leakage, underscoring the importance of stringent CORS policies in REST API development.
Error Handling and Validation
Validating User Inputs
Validating user inputs is a fundamental aspect of maintaining data integrity and preventing malicious input manipulation within REST APIs. By enforcing validation rules on incoming data, developers can eliminate common vulnerabilities such as injection attacks, buffer overflows, and data tampering. Input validation verifies the correctness and integrity of user-supplied data, ensuring that only sanitized inputs are processed by the API. Despite adding an initial overhead to request processing, robust input validation mechanisms contribute significantly to enhancing the security and reliability of REST APIs.
Error Responses and Status Codes
Effective error handling in REST APIs involves providing meaningful error responses and utilizing appropriate HTTP status codes to convey the outcome of API requests. Error responses should be informative, concise, and indicative of the encountered issue, aiding developers and users in troubleshooting potential issues. Status codes such as 4xx for client errors and 5xx for server errors delineate the nature of failures accurately, enabling quick identification and resolution of anomalies. Properly structured error responses not only enhance the usability of APIs but also foster transparent communication between clients and servers, bolstering the overall user experience.
Performance Optimization
Caching Strategies
Caching strategies are instrumental in optimizing response times, reducing server load, and enhancing the scalability of REST APIs. By storing frequently accessed data in cache memory, APIs can expedite subsequent requests and mitigate latency issues associated with repeated data retrieval. Strategies like browser caching, CDN caching, and server-side caching enable efficient data delivery and improve the overall responsiveness of APIs. However, implementing caching mechanisms requires careful consideration of cache expiration policies, cache invalidation strategies, and cache coherence mechanisms to ensure data consistency and freshness.
Optimizing Network Requests
Optimizing network requests involves minimizing latency, reducing bandwidth consumption, and enhancing the overall responsiveness of REST APIs. Techniques such as request batching, data compression, and resource minification aid in optimizing network utilization and accelerating data transmission. By efficiently managing network resources, APIs can deliver content swiftly, cater to a larger user base, and adapt to fluctuating network conditions. Nevertheless, optimizing network requests necessitates a balance between performance improvements and resource utilization to uphold the quality of service and responsiveness expected from modern REST APIs.
Conclusion
Mastering REST API with JavaScript is a crucial aspect in modern web development. As the digital landscape continues to evolve, the ability to proficiently work with REST APIs using JavaScript becomes a valuable skill set for developers. This final section of the comprehensive guide encapsulates the essence of the entire article, emphasizing the significance of mastering REST API concepts in JavaScript. Readers will gain insights into the foundational principles of REST architecture, effective API consumption techniques, and practical implementation strategies in JavaScript. By mastering REST API development, tech enthusiasts can elevate their proficiency and excel in designing robust, scalable web applications.
Mastering REST API with JavaScript
Summary of key takeaways
Delving into the summary of key takeaways, this section serves as a centralized reference point for readers to grasp the essential concepts explored throughout the guide. Understanding the importance of REST principles, efficient API consumption methods, and the implementation intricacies in JavaScript is paramount for mastering REST API development. The meticulous breakdown of key takeaways equips developers with a holistic understanding of REST architecture and its practical implications in modern web development. By internalizing these core concepts, developers can streamline their workflow, enhance productivity, and deliver top-notch REST API solutions proficiently.
Future trends in REST API development
Exploring the future trends in REST API development unveils the evolving landscape of web technologies and the trajectory of RESTful services in the digital realm. Anticipating emerging trends such as enhanced security measures, advancements in performance optimization techniques, and the integration of cutting-edge tools and frameworks underline the dynamic nature of REST API development. Embracing these future trends equips developers with the foresight to adapt to changing industry demands, leverage innovative solutions, and stay at the forefront of REST API development. Ultimately, staying informed about future trends empowers developers to deliver exceptionally optimized and secure REST APIs that meet the ever-evolving needs of users and businesses in the digital era.