Understanding Heap Min: A Deep Dive into Its Concepts
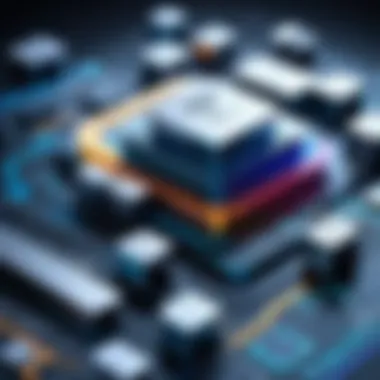
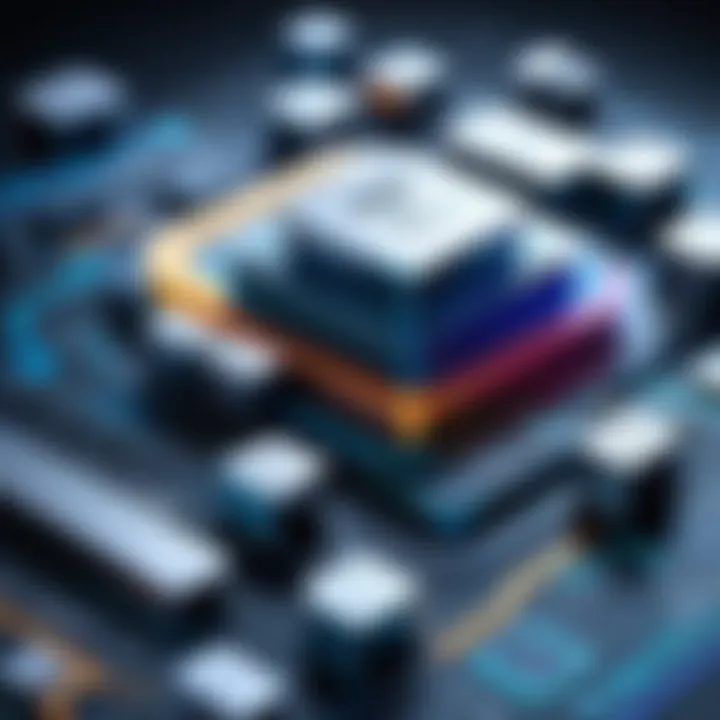
Intro
Heap Min is a crucial concept in computer science, particularly in data structure manipulation and algorithm efficiency. It refers to a specific classification of heaps, known for organizing elements in such a way that the smallest element is easily accessible. This article aims to unpack the intricacies of Heap Min, stressing its significance and applications in modern computation. By understanding its underlying principles and contrasting it with other data structures, one can appreciate its necessity in optimizing algorithms and improving computing efficiency.
Heap structures are foundational in various applications, from priority queues to graph algorithms. A minimum heap is defined by the property that every parent node is less than or equal to its child nodes, which allows for swift access to the smallest element. This function is invaluable in scenarios where quick retrieval of minimum values is essential.
Through this article, we will explore the functionalities, applications, and implications of Heap Min in a targeted manner, addressing the needs of tech enthusiasts and professionals alike.
Foreword to Heap Min
Heap Min plays a pivotal role in the understanding of data structures and algorithms, especially for those engaged in software development and system design. This section serves as the entry point into the intricacies of the minimum heap, elucidating its importance and applications in modern technology.
Definition and Concept
Heap Min, also known as a min-heap, is a specialized tree-based data structure that satisfies the heap property: for any given node, the value of that node is less than or equal to the values of its children. This property ensures that the smallest element is always located at the root of the tree, allowing for efficient access and manipulation of the least item. The conceptual foundation of Heap Min is critical as it underlines numerous algorithms, particularly those involved in priority queues and graph traversal methods.
The basic characteristics of a heap include its complete binary tree structure and the enforcement of the heap property. These qualities facilitate both insertion and deletion operations in logarithmic time complexity, making it a preferred option for scenarios requiring rapid access to the minimum element. This efficiency is significant in numerous applications across computer science, from pathfinding algorithms to real-time data processing.
Historical Context
The concept of Heap Min has been around since the early days of computer science, evolving alongside the development of data structures. The foundational principles of heaps were first articulated in the 1960s and have since been refined. Over the decades, Heap Min has gained traction in algorithmic design, particularly due to its effectiveness in implementing priority queues.
In the context of historical advancements, the introduction of Dijkstra's algorithm—and later the Fibonacci heap—demonstrates the practical applications of Heap Min in solving graph-related problems. Such historical reference illustrates Heap Min’s ongoing relevance; it continues to be integral in algorithm optimization and efficiency improvements. The development of various heap implementations across different programming languages further emphasizes this point. As programming frameworks evolve, so too does the implementation of heaps, solidifying their status as a core concept in algorithmic courses and practical software development.
Characteristics of Heap Min
The characteristics of Heap Min hold significant importance within the context of this article. Understanding these traits helps in grasping why this data structure is favorable in many algorithmic applications. Two major aspects are the structure and properties of Heap Min, and its comparison with the Max Heap.
Structure and Properties
Heap Min is defined as a specialized tree-based data structure that maintains a specific order property. In this structure, each parent node has a value less than or equal to its children’s values. This distinct arrangement allows for efficient retrieval of the minimum value, prominently advantageous when working with priority queues.
A Heap Min is typically implemented as a binary tree. However, it is important to note that it is a complete binary tree, meaning all levels are fully filled except possibly for the last level, which is filled from left to right. This structure is crucial as it ensures balanced trees and operations remain efficient.
The properties of Heap Min can be summarized in specific characteristics:
- Node Value Property: The key property is that for any given node, its value is less than or equal to its child nodes.
- Complete Binary Tree: Ensures all levels are filled, which aids in maintaining efficiency during operations.
- Dynamic Resizing: The Heap Min can adjust in size dynamically, making it suitable for applications where the amount of data is not fixed.
These properties make Heap Min particularly suitable for algorithms that frequently need to access the smallest elements.
Comparison with Max Heap
While Heap Min and Max Heap are both forms of binary heaps, they serve different purposes and offer varied advantages.
In a Max Heap, the parent node is greater than or equal to its children. This arrangement is beneficial when a fast maximum retrieval is necessary. The comparison with Heap Min highlights some important distinctions:
- Operation Complexity: In both structures, insertion and deletion operations can be performed in logarithmic time, but the specific focus on minimum versus maximum creates differences in usage scenarios.
- Use Case Suitability: Heap Min is ideal for implementational strategies requiring minimum value access, while Max Heap shines in scenarios demanding maximum value access.
- Data Type Manipulation: Depending on the problem at hand, choosing between Heap Min and Max Heap is crucial. The choice between them can influence the performance and efficiency of algorithms significantly.
Taking into account these differences allows algorithm designers to make informed decisions based on efficiency and task requirements.
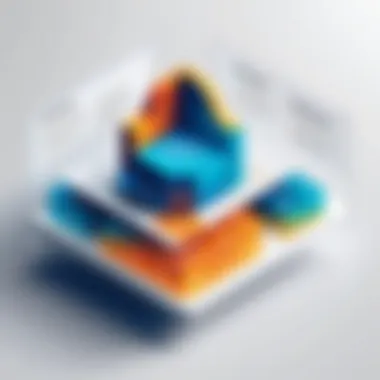
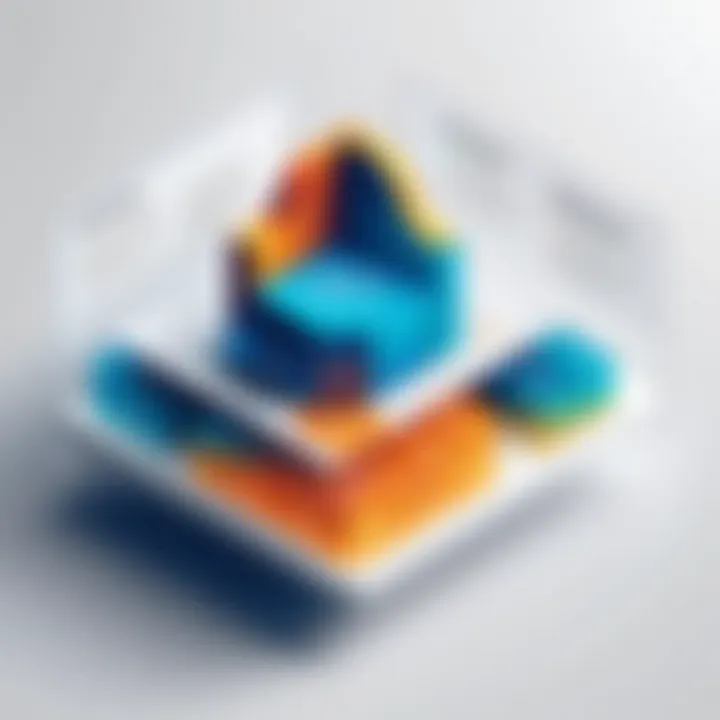
Heap Min Algorithms
Heap Min is not just a data structure; it is the foundation for various algorithms that enhance its functionality. Understanding these algorithms is crucial as they dictate how data interacts within the minimum heap. The efficiency of insertion, deletion, and heapification directly impacts application performance, making this section pivotal for comprehending the broader implications of Heap Min in computational settings.
Insertion Algorithm
The insertion algorithm in Heap Min is a systematic process that ensures order is maintained as new elements are added. The basic steps involve placing the new element at the end of the heap and then comparing it with its parent node. If the newly inserted element is smaller, they swap places. This process continues upward until the heap property is restored.
- Step-by-step process:
- Add the element to the end of the heap array.
- Compare the newly added element with its parent node.
- If the new element is smaller, swap it with the parent.
- Continue this until the heap properties are met.
This algorithm ensures a time complexity of O(log n), which is efficient and keeps the heap balanced. Maintaining this order is critical, particularly in applications where minimum values must be accessed swiftly.
Deletion Algorithm
When it comes to the deletion algorithm, it mainly targets the root element of the heap. To delete the minimum element, which resides at the root, the last element in the heap is moved to the root position. Following this, the heap must be restructured to maintain its properties.
- Deletion steps:
- Replace the root node with the last element in the heap.
- Remove the last element as it is now redundant.
- Perform a sift-down operation to restore heap order.
During the sift-down process, the new root is compared with its child nodes. If it is greater than either child, it swaps with the smallest of the two. This operation continues down the tree until the minimum heap structure is intact. The efficiency of this algorithm also aligns with O(log n) time complexity, making it suitable for performance-critical tasks.
Heapify Process
Heapification can be understood as the process used to transform an unordered array into a structured heap. This process can occur in two main contexts: creating a new heap from scratch and maintaining the heap structure after insertions or deletions.
- In Heap Min, the heapify process can be summarized in the following manner:
- Start from the last non-leaf node, moving upward to the root.
- Check whether each node violates the heap property. If it does, swap it with the smaller of its child nodes until the properties are restored.
The time complexity for the heapify process is O(n), which makes it significantly faster than performing multiple insertions. This efficiency is achieved because fewer comparisons are needed as one moves up the heap.
By understanding these algorithms, one can leverage the full potential of Heap Min in various real-world applications, optimizing both performance and resource management.
Applications of Heap Min
The applications of Heap Min illustrate its versatile utility across various computing domains. Understanding these applications is essential as they showcase how this data structure supports efficient algorithms in many practical scenarios. Rather than simply being a theoretical construct, Heap Min has real-world relevance due to its efficiency in certain operational contexts. Below, we explore three primary areas where Heap Min proves its value: priority queues, graph algorithms, and sorting algorithms.
Priority Queue Implementation
Heap Min serves as a foundational structure for implementing priority queues. A priority queue enables elements to be processed based on their priority, rather than just their order of arrival. In systems such as task scheduling or event simulation, the ability to quickly access the item with the highest priority is crucial.
Heap Min guarantees that the smallest element can always be removed efficiently, which corresponds to the highest priority in many applications. For example, in a task execution system, the task with the shortest execution time can be prioritized using a min-heap. The process works as follows:
- Insertion of new tasks maintains the heap property by placing the new task at the correct position.
- The removal operation fetches the task with the least execution time efficiently.
- Re-heapifying ensures the next smallest task becomes accessible for processing.
This efficiency optimizes the overall task execution time, illustrating the benefits of Heap Min in real-time applications.
Graph Algorithms
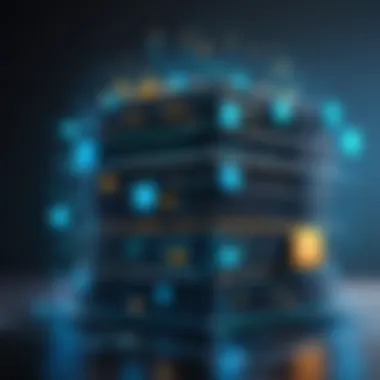
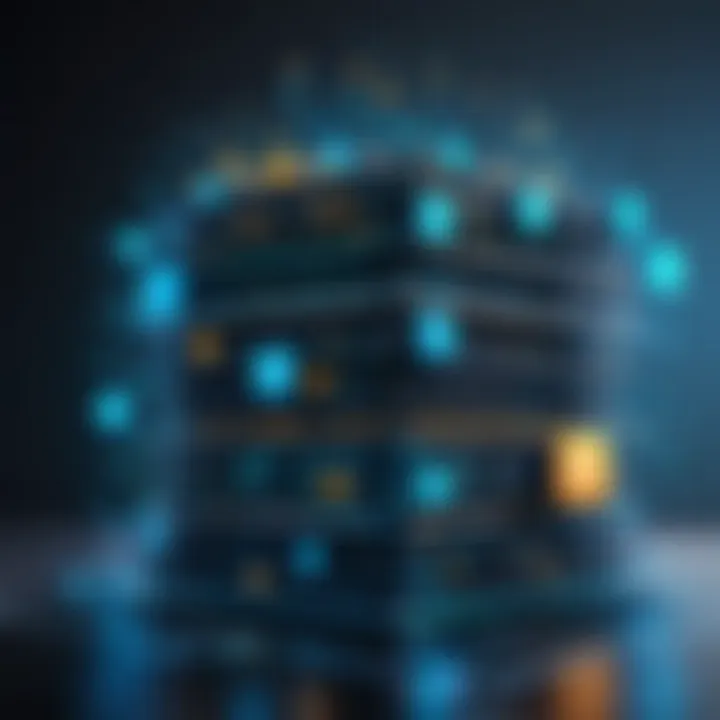
In the realm of graph algorithms, Heap Min finds significant application, particularly in algorithms such as Dijkstra's and Prim's. These algorithms rely on efficiently managing priority in a set of vertices or edges, where the smallest weight often represents the shortest distance or minimum connection weight.
Using a min-heap allows algorithms to efficiently extract the minimum vertex or edge, facilitating steps toward the solution. For instance:
- In Dijkstra's algorithm, the next vertex to explore is always the one with the smallest tentative distance. The min-heap maintains these distances and swiftly retrieves the minimal one for processing.
- Prim's algorithm, which constructs the minimum spanning tree, benefits similarly by allowing quick access to the least weighted edge as the tree grows.
This integration of Heap Min into graph algorithms enhances performance and reduces computation time, critical in large-scale networks or systems.
Sorting Algorithms
Heap Min also plays a role in sorting algorithms, particularly in Heapsort. This comparison-based sorting algorithm uses the heap structure to sort elements efficiently. Here's how it operates:
- The initial array is transformed into a min-heap, allowing the smallest element to be quickly accessible at the root.
- The first step in sorting involves removing the smallest element and placing it into the sorted portion of the array.
- The heap is adjusted, maintaining the min-heap property, allowing the next smallest element to be accessed quickly.
This process continues until all elements are sorted. The elegance of Heapsort lies in its performance, characterized by a time complexity of O(n log n), making it efficient for large datasets.
"Heap Min is not just a theoretical construct; it is a backbone for many practical applications in computing."
Performance Assessment
Performance assessment of Heap Min is a critical element in understanding its efficiency and practicality in various applications. This section focuses on two major areas: time complexity and space complexity. Assessing performance encompasses not only theoretical aspects but also practical implications. Understanding these metrics helps developers and tech professionals decide when and how to utilize Heap Min effectively.
Time Complexity Analysis
Time complexity measures how the execution time of an algorithm grows relative to the input size. In the context of a Heap Min, its operations exhibit predictable time complexities. The operations primarily associated with Heap Min include insertion, deletion, and heapify processes.
- Insertion: The time complexity for inserting an element into a Heap Min is O(log n). This occurs because the newly added element may need to
Comparative Analysis
In the context of data structures, Comparative Analysis serves as a foundational pillar for understanding Heap Min's functionality and its relative advantages. This section aims to dissect how Heap Min stands against other data structures, which is crucial for both theoretical comprehension and practical application. The analysis elucidates the distinct characteristics, strengths, and limitations associated with Heap Min, ultimately enriching the reader's grasp of its role in computing.
Heap Min vs. Other Data Structures
Heap Min is often compared to other data structures like arrays, linked lists, and various tree structures. Each of these alternatives has its own merits and demerits, which influence the decision of when to employ Heap Min.
- Arrays: Arrays provide fast access to elements through indexing but lack the ability to maintain a dynamic data structure effectively. In contrast, Heap Min efficiently manages insertion and deletion while keeping the minimum element accessible at the root.
- Linked Lists: While linked lists facilitate dynamic memory allocation, the time complexity for finding a minimum element remains linear. Heap Min, on the other hand, offers logarithmic time complexity for insertion and deletion, which is a significant advantage for scenarios requiring frequent access to the smallest element.
- Binary Search Trees: Compared to binary search trees which allow for ordered element retrieval, Heap Min excels at maintaining a priority queue. Accessing and managing the least element in a minimum heap is generally faster than navigating through a binary search tree, especially with operations like priority queuing.
- Other Heaps: When contrasted with Max Heaps, Heap Min's main distinction is its structure and primary focus on the minimum value. This characteristic opens up specific applications in scenarios like scheduling and resource management, where the minimum value holds paramount importance.
The depth of this comparative analysis supports the understanding that different use cases arise from varying characteristics of Heap Min. With its strengths notably lying in efficiency, dynamic nature, and priority handling, it establishes itself as a formidable candidate in algorithm design, especially in contexts requiring optimal data manipulation.
Use Cases for Heap Min
Heap Min finds its utility across numerous domains due to its efficient data management capabilities. Some prominent use cases include:
- Priority Queues: In operating systems or process management, priority queues are imperative for task scheduling. Heap Min can be used to ensure that the highest-priority jobs are executed first.
- Graph Algorithms: In algorithms like Dijkstra’s Shortest Path, where quick access to the smallest weights is essential, using Heap Min ensures efficient performance.
- Sorting Algorithms: Heap Sort is a classic sorting algorithm that employs Heap Min to maintain the order of elements efficiently.
- Streaming Algorithms: When tracking minimum values in large, streaming datasets, Heap Min allows for dynamic updates, ensuring timely access to the current minimum without the need for constant data re-sorting.
Understanding these use cases emphasizes how Heap Min integrates with various disciplines in tech, further highlighting its importance as a core data structure.
Challenges and Limitations
Understanding the challenges and limitations of Heap Min is crucial for any tech enthusiast or industry professional. While Heap Min offers certain advantages, such as efficient access to the minimum element, it also presents several challenges that developers must navigate. Addressing these limitations not only aids in optimizing application performance but also enhances one’s ability to make informed decisions regarding data structure selection in various scenarios.
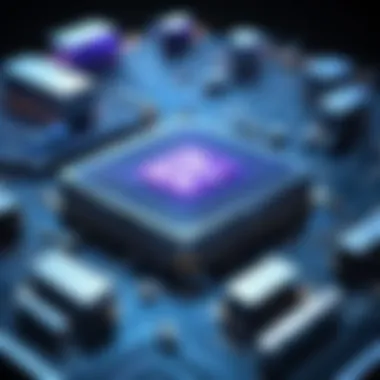
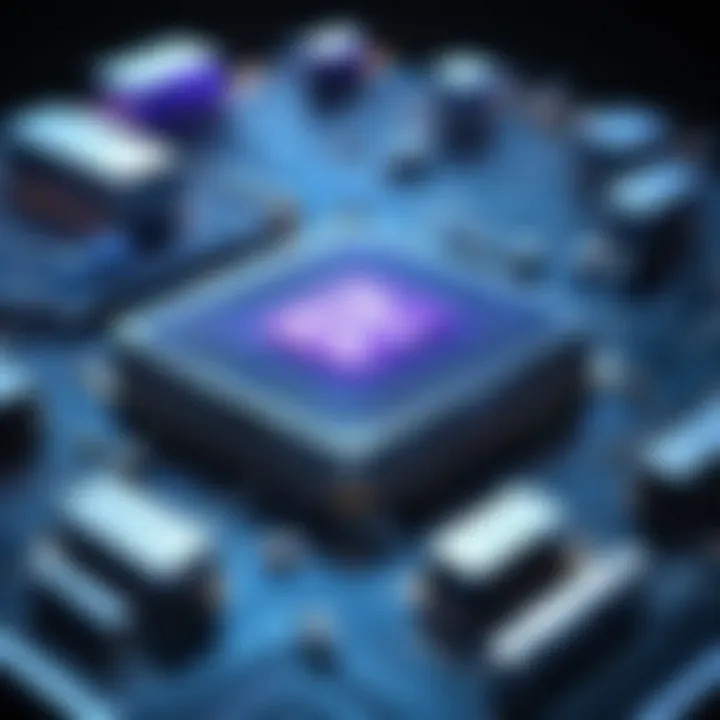
Maintenance of Heap Structure
Maintaining the heap structure is a significant challenge. The heap needs to remain balanced at all times during insertion and deletion operations. With every insertion of a new element, the structure may become unbalanced. Maintaining the minimum condition during these operations is not trivial. The process of re-heapifying (or restoring the heap property) can lead to inefficient operations if not implemented carefully.
Consider the performance impact: for every insert or delete, the operational complexity is typically O(log n). If the heap is not properly maintained, efficiency can drop considerably, which can be detrimental in applications relying on frequent priority updates.
Moreover, the complexity multiplies in a concurrent environment. Different threads trying to manipulate the heap simultaneously can lead to various issues, like race conditions and data inconsistency. This necessitates the implementation of locks or other synchronization mechanisms, which can add overhead and degrade performance.
Scalability Considerations
Scalability is another essential factor when dealing with Heap Min. As data grows in volume, heaps can encounter significant limitations. The traditional array-based implementations may lead to inefficient memory usage. For large datasets, linked-list based implementations can provide an alternative; however, they can introduce their own challenges regarding access speed.
In addition, as the demand for real-time processing increases, the limitations of Heap Min in handling large-scale data become evident. For example, operations on large heaps can be slower due to their height, affecting response times, especially in applications like real-time data analytics or streaming.
"Optimizing the performance of Heap Min is not just about how the data is structured, but also about how it can be scaled efficiently to meet demand."
Lastly, there is also the aspect of adaptability. As technologies evolve, the requirements for data operations can change. The static nature of many heap implementations can pose problems in rapidly changing environments. Organizations must be prepared to weigh these challenges against the benefits when considering Heap Min as a viable solution in their projects.
Future Trends in Heap Min Usage
The examination of future trends in Heap Min usage is crucial for understanding how this data structure will evolve and adapt in a rapidly changing technological landscape. Its relevance in various applications promises to enhance computational efficiency and optimize processing capabilities. As more industries rely on data-driven decision-making, the demand for efficient algorithms and data management techniques will only increase. Consequently, Heap Min offers several benefits that are becoming increasingly significant.
Integration with Modern Technologies
The synergy between Heap Min and modern technologies is noteworthy. As companies explore machine learning, artificial intelligence, and big data analytics, the optimization of algorithms becomes essential. Heap Min can support faster data retrieval, which is invaluable in real-time processing environments. For instance, it is often employed in priority queue management, ensuring that tasks are processed in a timely manner without compromising system performance.
Moreover, the integration of Heap Min with programming languages and frameworks is also on the rise. Libraries in Python, Java, and C++ include Heap Min implementations that make it easier for developers to utilize this structure in their applications. The compatibility of Heap Min with emerging technologies ensures that it remains relevant and widely applicable.
Enhanced Algorithms and Innovations
The landscape of algorithms is continually evolving. Researchers and developers are continuously working towards refining algorithms that utilize Heap Min. One significant trend involves enhancing the efficiency of the insertion and deletion processes, which are crucial for maintaining the heap properties. Improved algorithms are being developed to reduce time complexity, enabling faster operations.
Another aspect is the focus on parallel processing. Leveraging multi-core processors can lead to dramatic reductions in processing time for Heap Min operations. Innovations in this domain open doors for applications that were previously limited due to performance bottlenecks. By adapting algorithms to support concurrency, Heap Min can maintain its edge in speed and efficiency.
Future advancements in algorithms will redefine the way we approach data structures and their applications, ensuring Heap Min remains at the forefront of algorithm optimization.
In summary, the future trends in Heap Min point to a continuous integration with modern technologies and an ongoing commitment to enhanced algorithms. These developments collectively underscore the growing importance of Heap Min in various applications, promising greater efficiency and adaptability as computing demands escalate.
Ending
In this concluding section, we emphasize the significance of Heap Min in computer science and its notable relevance in various practical applications. The exploration of Heap Min throughout this article has unveiled key aspects of its structure, algorithms, and operational efficiency. Understanding the efficiency of Heap Min not only enhances algorithmic optimization but also facilitates effective data management.
Summary of Key Insights
Heap Min is defined by its unique properties that enable it to efficiently retrieve the minimum element in a dataset. The article highlighted the following key insights:
- Fundamental Structure: The heap's binary tree structure underpins its efficiency. Each parent node is less than or equal to its child nodes.
- Algorithm Efficiency: The algorithms for insertion, deletion, and heapification emphasize efficiency in both time and space complexities.
- Wide Applications: From priority queue implementations to critical roles in graph algorithms and various sorting algorithms, Heap Min has proven its versatility.
- Challenges and Limitations: While Heap Min is widely applicable, issues such as maintaining the heap structure and scalability have critical implications for its use in larger datasets.
The thorough analysis of these points demonstrates that Heap Min is more than just a data structure; it is a foundational component that supports various logical constructs within more complex systems.
Final Thoughts on Heap Min
In sum, Heap Min stands out for its robust design and efficiency in various computational tasks. It is vital for anyone in the tech industry to grasp the principles behind it. The integration with modern technologies and the ongoing innovations in algorithms will only enhance its utility in future endeavors. Tech enthusiasts and industry professionals must keep abreast of developments surrounding Heap Min to harness its full potential in their projects and algorithms. This article serves as a comprehensive guide, offering insights essential for a nuanced understanding of Heap Min that underscores its importance in algorithm design and implementation.
"Understanding data structures like Heap Min is crucial for optimal algorithm design, impacting performance across applications."
As we look forward, embracing advancements and integrating such structures into new technologies will likely redefine how data is processed and managed.