Mastering Senior Software Engineer Coding Questions
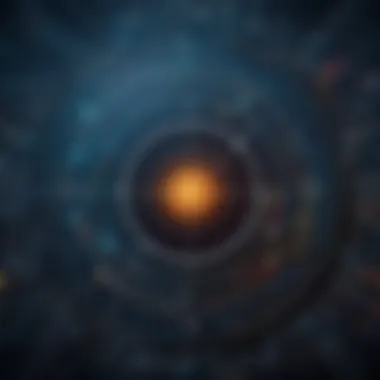
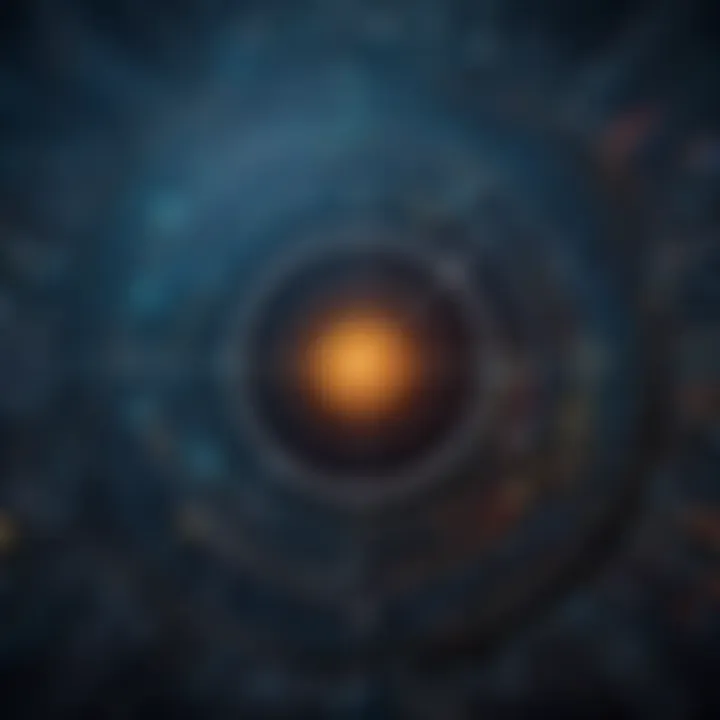
Intro
Navigating the turbulent waters of a career as a senior software engineer involves more than just knowing how to write code. It requires an intricate understanding of various algorithmic concepts, data structures, and the ability to tackle coding challenges with finesse and clarity. If you’re aiming for a seat at the senior table, it’s crucial to prepare yourself by diving into the types of coding questions that frame this challenging interview process. This section will shed light on what lies ahead in the article, highlighting the key points that will be discussed, their relevance, and the necessary knowledge that aspiring engineers should carry with them.
Understanding coding questions at this level means grappling with complexities and nuances that differentiate a junior from a senior. It’s like jumping from a kiddie pool into the ocean—vast and filled with deep mysteries. It’s not only about robust coding skills but also includes architectural decisions and optimal algorithm choices in different contexts. The direct aim here is to arm candidates with tools to dissect problems, drawing from theoretical knowledge while simultaneously honing practical applications.
The essence of this guide revolves around categorizing coding challenges by their nature—ranging from algorithms and data structures to payment systems and software design principles. Our journey will cover:
- Essential Algorithms: How to implement common algorithms and the scenarios where they shine.
- Data Structures: The foundation that software engineering rests upon; understanding when and how to use each structure efficiently.
- Problem-Solving Techniques: Not all problems are created equal. We’ll discuss varied strategies for different types of coding enigmas, ensuring a methodical approach leads to coherent solutions.
- Interview Strategies: Tips on how to present your thought processes effectively while tackling coding questions, merging both technical and behavioral understandings into one seamless narrative.
As we peel back the layers of these intricate topics, you'll gain insight into how theoretical concepts translate into practical knowledge that equips you for interviews and real-world applications. This is not just a checklist; it’s an investment in your future as a senior engineer. So, buckle up and prepare to drill down into the coding questions that could shape your career.
Preface to Coding Questions
In a world where the tech industry evolves at a breakneck speed, coding questions ignite important conversations about skills and knowledge. They're not just a tedious hurdle in the interview process; they are essential checkpoints. Grasping coding questions prepares you for the practical challenges faced daily. The significance of these questions extends beyond mere answers; they dig deep into a candidate's analytical prowess and problem-solving capability.
The Importance of Coding Questions
Coding questions serve as a funnel, guiding interviewers toward identifying the right candidate for their team. They help to uncover whether a candidate can think on their feet and demonstrate proficiency in tackling problems that arise in software development. Additionally, coding challenges align well with various industry demands:
- Real-World Application: These questions mirror day-to-day tasks that a Senior Software Engineer might encounter, ensuring candidates possess practical skills rather than just theoretical knowledge.
- Assessment of Solution Strategy: Interviewers want to see not only if candidates can come up with a solution but also how they go about it. The approach matters just as much as the destination.
- Techniques Testing: From algorithms to data structures, these questions often test the fundamentals vital in software engineering, making candidates rehearse critical concepts.
Moreover, coding problems often vary in difficulty, which helps interviewers gauge depth of knowledge and adaptability. Candidates who can navigate through snags in code or debug under pressure demonstrate a degree of resilience that's highly prized.
Overview of a Senior Software Engineer Role
A Senior Software Engineer is akin to a captain steering a ship through turbulent waters. Their role encompasses a range of competencies both technical and leadership-based. This position demands not just coding chops but a comprehensive understanding of system architecture, database management, and user experience.
Key responsibilities typically include:
- Leading Projects: From inception to delivery, they spearhead initiatives, coordinating between teams to ensure milestones are met.
- Mentoring Team Members: Veterans in the field often mentor juniors, fostering a culture of learning and collaboration.
- Code Reviews and Quality Assurance: Ensuring that the codebase remains robust and maintainable is a priority. This combines development practices with a keen eye for detail.
Furthermore, the right candidate for a Senior Engineer position must be adaptable, handling changes in project scope or technology stack gracefully. This strategic mindset is precisely what coding questions aim to evaluate. Ultimately, armoring oneself with a solid understanding of coding concepts not only bolsters one’s prospects of landing a job but also paves the way for a successful career in an ever-evolving industry.
Categories of Coding Questions
In the world of software engineering, coding questions serve as the cornerstone for evaluating a candidate’s proficiency and problem-solving abilities. These questions are not merely academic; they act as litmus tests for the skills a senior engineer must possess in real-world scenarios. While coding interviews might seem intimidating, understanding the categories can demystify the process and highlight the essential competencies required.
By familiarizing oneself with the various coding question categories, candidates can hone their skills in targeted areas, making their preparation more effective. Each category covers different aspects of programming and systems thinking, enabling interviewers to assess not only coding speed but also depth of knowledge and design thinking.
Algorithmic Challenges
Algorithmic challenges are quite often viewed as the bread and butter of coding interviews. They test a candidate’s ability to solve problems by designing efficient algorithms. This might involve sorting data, searching through structures, or performing complex operations like finding paths in a graph. The key focus here is on efficiency and optimization. Candidates are expected to know the time and space complexity of their solutions.
To provide a clearer picture, here’s what one might typically encounter:
- Sorting Algorithms: Questions may revolve around implementing or optimizing classic algorithms like QuickSort or MergeSort.
- Searching Algorithms: Expect to deal with binary search scenarios or algorithms to locate elements in sorted data.
- Dynamic Programming: Problems that necessitate memorization of past results to optimize future calculations are common, quite the brain-teaser!
For instance, being asked to find the longest increasing subsequence in an array can reveal a candidate’s mastery over classic dynamic programming techniques. These challenges help gauge technical acuity and analytical thinking, where elegance in solution design is just as important as obtaining the correct output.
Data Structure Questions
Data structures form the skeleton of software applications, making it essential for senior software engineers to command various structures. Questions in this category often involve manipulating or optimizing data storage solutions. Knowing how and when to use an appropriate data structure is pivotal for achieving optimal performance.
Commonly tested structures include:
- Arrays and Lists: Expect scenarios requiring you to convert between these structures or manipulate them for optimal performance.
- Trees and Graphs: These structures often present unique traversal challenges. Knowing how to effectively manage and utilize these can earmark candidates as suitable for senior roles.
- Hash Tables: Questions might ask for implementation or optimization of hash-based solutions to achieve rapid lookups.
For example, a question asking to implement a LRU (Least Recently Used) cache taps into both the understanding of hash tables and the algorithmic logic behind cache management. This intersection of knowledge underscores a candidate's readiness for real-world software challenges.
System Design Scenarios
System design interviews are often the crown jewels of senior software engineer evaluations. This category isn't about writing code on the spot, but rather, it’s about showcasing one’s ability to architect solutions from the ground up. Candidates discuss trade-offs, scalability, reliability, and maintainability of systems through various methodologies.
When delving into system design scenarios, candidates might need to explain:
- High-level architecture: Outlining a system’s components and their interactions becomes vital.
- Data flow and storage: Detailing how data is managed through a system gives insight into data integrity and performance.
- Load balancing and failover: Discussing how a system handles high traffic and potential failures is crucial for assessing real-time application effectiveness.
For instance, designing a social media platform may require a candidate to evaluate how to handle user authentication, content delivery, and notifications simultaneously. This comprehensive approach allows candidates to exhibit both their technical prowess and their strategic foresight.
Understanding the categories of coding questions enables candidates to structure their preparation and align their skills with industry expectations. Candidates who are adept in these areas will not only perform well in interviews but also excel in their roles as senior software engineers.
Bold preparation in these question categories can lay a strong foundation for a rewarding career in the tech industry.
Key Algorithms to Master
In the world of software engineering, algorithms can often be the backbone of efficient solutions. Mastering key algorithms not only enhances a candidate’s problem-solving arsenal but also gives a glimpse into their logical thinking and analytical capabilities. When a senior software engineer can demonstrate a solid command of vital algorithms, they often stand out in interviews and on the job.

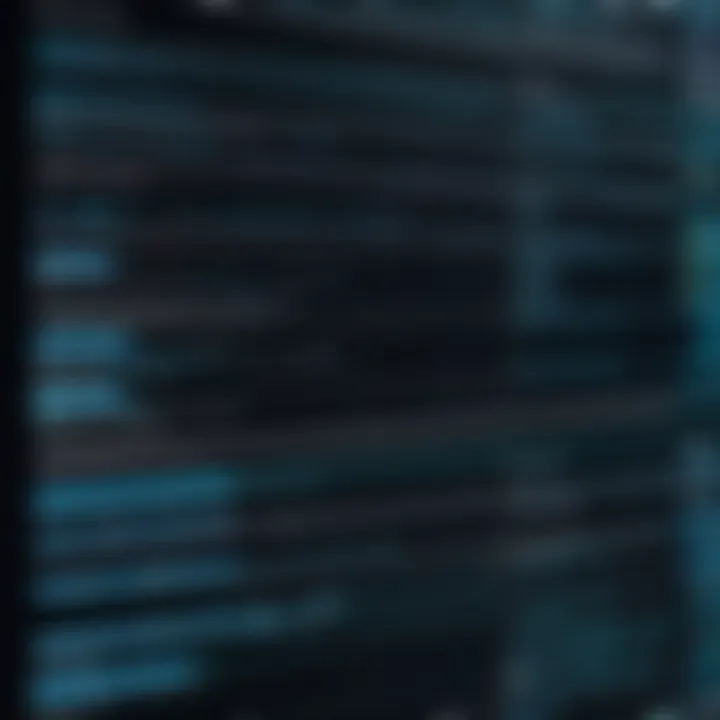
The importance of understanding algorithms lies in their application. Many real-world challenges can be boiled down to algorithmic solutions. Hence, grasping the nuances of various algorithms allows engineers to craft adaptable and optimized code. This section will unpack critical algorithm categories, offering an eye into their mechanisms and relevance.
Sorting Algorithms
Sorting algorithms rank among the most foundational tools in a software engineer’s toolkit. Whether it's organizing data for quicker access or simplifying subsequent operations, sorting plays a crucial role. Common algorithms like Quick Sort, Merge Sort, and Bubble Sort vary in efficiency and use cases.
- Quick Sort is often favored for its average-case efficiency of O(n log n) and its in-place sorting capability, making it ideal for large datasets.
- Merge Sort, on the other hand, shines with its stable sorting approach, especially valuable in linked lists.
In many scenarios, understanding the trade-offs and characteristics of these algorithms can help in selecting the right one for the task at hand.
Search Algorithms
Searching through data is another core function within software development. Algorithms like Binary Search and Breadth-First Search (BFS) can efficiently navigate through vast datasets.
- Binary Search is highly efficient with a time complexity of O(log n), but it requires sorted data beforehand.
- BFS is instrumental in graph-related scenarios, allowing for level-order traversal and is essential when analyzing networks or trees.
Incorporating these techniques into one’s solution repertoire can streamline functionality while improving performance.
Dynamic Programming Techniques
Dynamic programming is a powerful method for solving complex problems by breaking them down into simpler overlapping sub-problems. This approach proves particularly effective for optimization tasks where a straightforward recursion approach may lead to excessive computations.
Key examples include:
- The Fibonacci sequence, where memorization cuts down on redundant calculations.
- The Knapsack Problem, serving as a classic optimization challenge often encountered in coding interviews.
Learning how to employ dynamic programming can provide a significant advantage in competitive coding environments.
Graph Algorithms
Graphs show up more often than expected, especially in modern applications like social networks, maps, and routing systems. Dijkstra’s Algorithm and A Search Algorithm* serve distinct purposes in graph traversal and pathfinding.
- Dijkstra’s Algorithm is fantastic for finding the shortest path in a weighted graph, showcasing how effective algorithm design can lead to swift solutions.
- A* is often preferred in game design and robotics for its clever heuristics that help anticipate the best path based on the explorative function.
Understanding graph algorithms widens the scope for engineers, enabling them to tackle problems relevant to various industries.
"In the realm of software development, algorithms are not a mere set of instructions but the very essence of creative problem-solving."
The mastery of these algorithms contributes significantly to a senior software engineer’s ability to deliver sophisticated solutions in a timely manner. As coding interviews often hinge on these principles, being well-versed in algorithms underpins both theoretical understanding and practical application.
Essential Data Structures
In the realm of software engineering, a firm grasp of data structures is not merely beneficial; it's foundational. Data structures serve as the backbone for efficient data management and manipulation, encapsulating the methods of storing, organizing, and retrieving data effectively. For senior software engineers, being well-versed in essential data structures provides the tools necessary to tackle complex coding questions with ease and finesse.
Importance of Essential Data Structures
Data structures help in optimizing algorithms and ensuring that operations such as insertion, deletion, searching, and traversal occur in a timely manner. A strong understanding of these structures can lead to significant performance enhancements which could be the difference between a program that runs quickly and one that crawls. In interviews, coding tasks often revolve around these structures; knowing them is non-negotiable. They also allow engineers to think critically about how best to store data depending on its usage patterns.
Arrays and Lists
An array is probably the simplest data structure one can encounter. It offers a fixed-size sequence of elements, accessible in a random manner based on indices. Considered a classic choice in programming, arrays allow engineers to perform operations like sorting and searching efficiently.
Lists, on the other hand, are more flexible. Unlike arrays, they can grow or shrink as needed, making them particularly useful when the exact amount of data to hold is not known in advance.
To illustrate, if you have a fixed set of enemy characters in a game, an array might serve well to store their stats. But if new characters must be added often, a list would shine.
Stacks and Queues
Stacks and queues are both abstract data types that comply with specific ordering principles.
- Stack: Functions on a Last In First Out (LIFO) principle, where the last added element is the first one to be removed. Think of it as a pile of plates; the plate added last is the first one removed. Stacks are vital for managing function calls or parsing expressions.
- Queue: Operates on a First In First Out (FIFO) basis. Imagine a line at a grocery store; the first person in line is the first one to be served. Queues are instrumental when handling requests in programming tasks like task scheduling.
To use these structures effectively, consider situations where the nature of data retrieval affects performance. Choosing between using a stack or a queue can change program outcomes significantly.
Trees and Graphs
Trees represent hierarchical data structures with a root value and sub-nodes, while graphs consist of nodes connected by edges.
- Trees: Particularly useful when implementing data that is inherently hierarchical, such as organizational structures or file systems. A binary search tree is of paramount importance, as it allows data to be organized in a way that significantly speeds up search operations.
- Graphs: These can either be directed or undirected and are used to represent complex relationships, such as social networks. Understanding how to traverse graphs using algorithms like depth-first search or breadth-first search can be pivotal when faced with real-world problems.
Hash Tables
A hash table is a unique structure that enables key-value pairs for data retrieval. It offers average case constant time complexity in search operations, making it a fast choice for storing data where rapid access is essential. Picture a library where each book is assigned a unique number; that number allows for speedy retrieval, much like a hash function maps keys to specific slots in the hash table.
However, one must be wary of collisions—instances where two keys hash to the same index. Techniques such as chaining or open addressing are commonly applied to resolve these collisions.
"Understanding these structures is not simply an academic pursuit; it's about empowering your programming to solve tangible problems with elegance."
In summary, mastering these essential data structures equips senior software engineers with the insight and skill to handle complex coding questions effectively, going beyond mere memorization to a deeper understanding of data flow and manipulation.
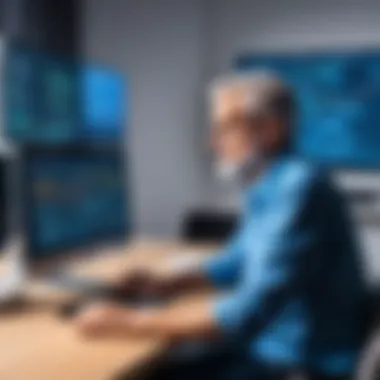
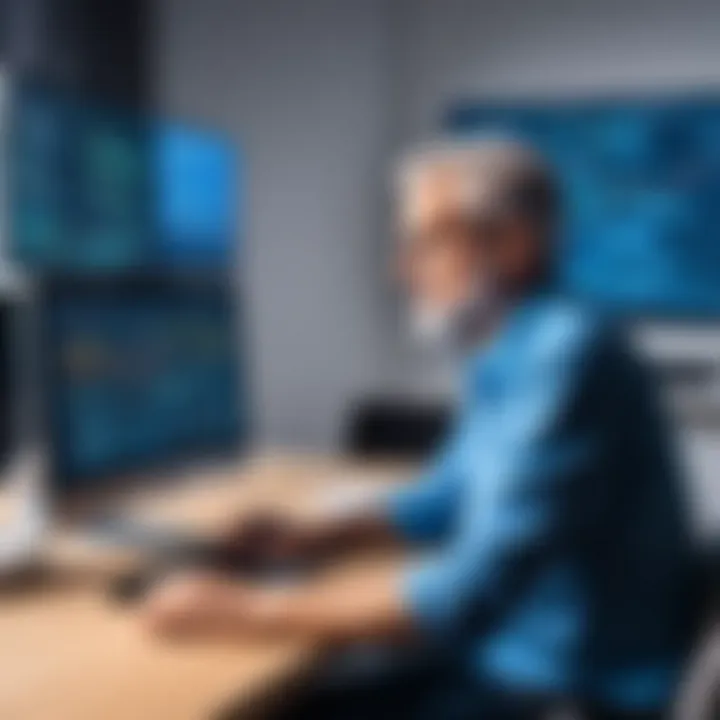
Common Coding Question Types
Understanding the types of coding questions often faced by senior software engineering candidates is pivotal. These questions not only test technical skills but also assess how well one can apply this knowledge to solve practical problems. In many ways, they serve as a bridge between theoretical knowledge and real-world application.
Familiarity with these types can drastically improve confidence. Knowing what to expect allows candidates to dive deeper into their studies rather than just floundering around guessing what might come up. Here, we’ll dissect three common types of questions: string manipulation, matrix and grid challenges, and concurrency problems.
String Manipulation Problems
String manipulation problems are staples in coding interviews. These questions often involve tasks like reversing a string or counting the number of vowels. These manipulations may seem simple, but they reveal a lot more about a candidate's logic and proficiency with programming languages.
The importance here lies not just in finding answers, but in how one approaches these challenges. For instance, consider the problem of determining whether two strings are anagrams. This involves analyzing character counts and utilizing hash tables for efficient lookup.
Why focus on string manipulation?
- It sharpens one’s ability to handle edge cases, such as empty strings or strings with varying character sets.
- It enhances understanding of algorithms and data structures like arrays and hash maps.
- String manipulation forms the backbone of many applications, from data parsing to user input validation.
Here’s a quick example of how one might check for anagrams:
Matrix and Grid Challenges
Matrix challenges require candidates to think beyond linear structures. The ability to navigate through a two-dimensional array can feel a bit like solving a jigsaw puzzle. Whether it’s searching for a specific value or solving a pathfinding problem, understanding these operations is key in many software applications, especially in gaming and data visualization.
These challenges hold significance for several reasons:
- Real-world applications: Features like path navigation in maps or game development require efficient traversal techniques.
- Algorithm depth: Knowing how to implement algorithms such as depth-first search or breadth-first search in a matrix context can demonstrate algorithmic thinking.
Consider a common matrix problem: finding the maximum sums of any subarray. Developing an efficient strategy would require familiarity with dynamic programming and possibly techniques from the algorithmic realm to ensure optimal performance.
Concurrency Problems
Concurrent programming challenges focus on how multiple threads or processes interact. These questions are particularly crucial in today's world of distributed systems and parallel processing. Questions might include how to manage shared resources or prevent race conditions.
There’s a lot on the line here, as concurrency can be a double-edged sword:
- Efficiency versus safety: Poorly managed concurrency can lead to deadlocks or data corruption, while well-structured concurrent programs can vastly improve application performance.
- Industry relevance: Many companies today depend on systems that need to handle simultaneous operations, so demonstrating understanding in this area can be a real leg up.
For example, you might encounter a question about implementing a thread-safe counter. Here's a simple approach using Python:
Utilizing these fundamental question types can bolster not just coding skills, but also overall problem-solving techniques. By mastering string manipulations, matrices, and concurrency, candidates will be better prepared to tackle the complex challenges posed in software engineering.
Problem-Solving Strategies
In the realm of software engineering, particularly for senior roles, the ability to solve problems efficiently and effectively can set a candidate apart from the rest. Coding questions are not merely tests of knowledge but rather a reflection of one's problem-solving mentality. This section steers through essential problem-solving strategies that will equip aspiring engineers to tackle even the trickiest of coding queries.
Understanding Problem Requirements
First and foremost, understanding the problem at hand is a prerequisite for successful coding. One must dissect the requirements, keeping in mind that an ambiguous understanding can lead to a flurry of missteps. Consider these steps:
- Read the problem statement diligently: Take time to absorb every word. One often skims through while thinking they’ve grasped it but frequently misses critical details.
- Identify inputs and outputs: Distinguish what is given and what needs to be returned. This step helps in visualizing the data flow, simplifying the following logic.
- Clarify assumptions: Ensure you are not making any unverifiable assumptions. Asking clarifying questions can illuminate areas of confusion.
By ensuring a solid grasp of the problem, one can strategize effectively rather than wander aimlessly through the code.
Breaking Down Challenges
Once the problem requirements are crystal clear, it’s time to slice the problem into manageable parts. Breaking down challenges serves not just to simplify the complexities but also aids in crafting a robust solution. Here’s how you can approach it:
- Decompose the problem: Break it into smaller sub-problems, addressing each logically. For instance, if you need to sort a dataset before processing, think about the sorting mechanism first.
- Use diagrammatic representations: Flowcharts, graphs, or even simple sketches can help visualize how components interact. This method often clarifies thought and elucidates the path forward.
- Draft pseudocode: Writing pseudocode allows you to outline your thought process without getting lost in syntax. This step is foundational; it’s like drawing a roadmap before hitting the road.
Taking time to dissect a problem can transform a daunting coding challenge into a series of straightforward steps, making it manageable under pressure.
Writing Clean, Efficient Code
At the heart of software engineering lies the mantra of writing clean and efficient code. This isn’t just a practice but a mindset. It showcases professionalism and technical prowess. Several key factors contribute to achieving this goal:
- Choose meaningful variable names: Avoid cryptic abbreviations. Meaningful names give context to the code and ease understanding for others who might read it.
- Keep functions focused: Each function should ideally do one thing, encapsulating logic appropriately. This modular approach enhances clarity and reusability in future projects.
- Optimize for performance: While solving immediate problems, also consider the impact of your choices long-term. Will the solution scale efficiently with larger data sets? Performance matters, especially when handling real-world applications.
Writing clean, efficient code is a skill that goes beyond immediate tasks; it influences future maintainability and collaboration in projects.
"A good programmer is someone who always looks both ways before crossing a one-way street."
Utilizing Practice Environments
The domain of software engineering demands a blend of theoretical knowledge and relentless practice. When gearing up for a senior software engineer position, proficiency in coding goes beyond rote memorization of algorithms and data structures. It necessitates real-life application of those concepts in various scenarios. This is where utilizing practice environments becomes crucial. Such environments simulate real coding challenges while providing a safe space for trial and error. Not only do they familiarize you with a variety of problems, but they also expose you to different coding styles and solutions. Thus, they become an integral part of your preparation strategy.
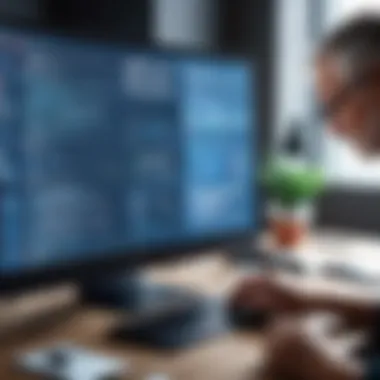
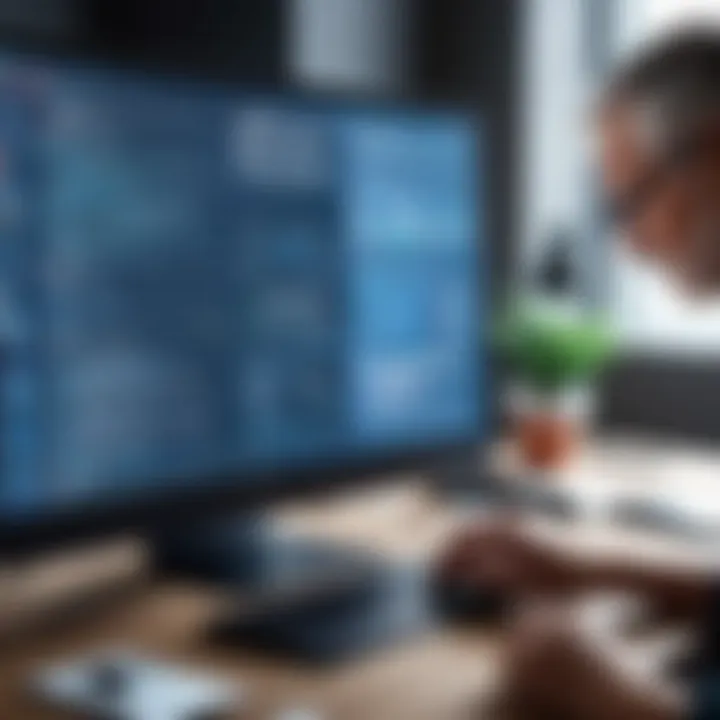
Popular Online Coding Platforms
In today’s digital age, numerous online platforms offer an opportunity to practice coding questions and refine one's skills. These platforms host a plethora of problems ranging in difficulty that can help simulate interview conditions. Here are some noteworthy options:
- LeetCode: Renowned for its extensive database of coding questions, LeetCode also allows users to discuss solutions with peers. The platform routinely updates its problem set to mirror industry trends.
- HackerRank: This platform stands out due to its community-oriented approach. Aside from practice, participants can take part in contests and hackathons which help gauge one’s skills alongside others.
- CodeSignal: CodeSignal offers a tailored experience with a focus on the skills that employers value most. They provide a variety of coding challenges that mimic real-world scenarios.
- Coderbyte: Known for both its quantity and quality, Coderbyte provides challenges along with solutions that can help clarify complex topics.
By engaging regularly with these platforms, you not only sharpen your coding abilities but also build confidence for upcoming interviews.
Participating in Coding Challenges
A key benefit of utilizing practice environments is participation in coding challenges. These contests are often designed to test real-time problem-solving abilities under pressure, mirroring the intensity of actual technical interviews. Here are a few elements to consider when diving into these challenges:
- Time Management: Coding challenges typically present a time constraint that mimics real-life deadlines. Timing yourself can help refine your ability to think quickly and prioritize effectively.
- Diverse Problem Types: Most coding contests feature a medley of challenges, which encourages adaptability. Whether it’s string manipulation, dynamic programming, or system design, the exposure is irreplaceable.
- Networking Opportunities: Engage with your peers during contests. You’ll often find forums and discussion boards where participants share insights, and you can learn from their approaches.
- Feedback Loop: Most platforms provide instant feedback on the solutions you submit, which aids in understanding mistakes and improving your skills.
Keeping a pulse on these challenges not only builds your expertise but also showcases your commitment to continuous improvement. Aligning with the coding community through these challenges can significantly enhance your technical readiness.
"Continuous learning is the key to staying ahead in the ever-evolving landscape of technology."
In summary, utilizing practice environments turns preparation into a systematic and enjoyable process. By leveraging online coding platforms and actively engaging in coding challenges, you can enhance your technical skills to tackle any question that comes your way.
Mock Interviews and Peer Reviews
Mock interviews and peer reviews serve as a backbone for preparing for the rigorous coding environment faced by senior software engineers. They’re not just simulation exercises; they help in honing both technical skills and interpersonal abilities. When approaching a mock interview, candidates often grapple with the pressure and urgency similar to that of a real interview. This practice helps in desensitizing that pressure, allowing candidates to perform at their best when it truly counts.
Importance of Mock Interviews
Mock interviews play an instrumental role in shaping a candidate's readiness. Here's why:
- Practice Under Pressure: The simulated environment mirrors an actual interview. Engaging in these mock sessions allows for a test drive of one’s skills. How well you handle questions can dictate how you’ll fare when the real deal comes around.
- Understanding the Format: Familiarity with the interview structure is crucial. Many engineers focus solely on coding challenges but might overlook behavioral questions or design questions. Mock interviews provide a comprehensive overview of what you'll encounter.
- Identifying Weaknesses: It becomes easier to pinpoint areas needing improvement. Feedback post-interview can highlight gaps in knowledge that need addressing before the actual interview.
Receiving Constructive Feedback
Getting constructive feedback is like gold dust in the preparation process. It’s not merely about being told that your solution was correct or not; it’s about the why behind it. Here’s how constructive feedback can enhance the preparation journey:
- Clarity on Suggestions: Peers or mentors can provide insights on how to approach problems differently. For instance, they may suggest optimizing your code or recommending alternative algorithms that may yield better performance.
- Contextual Understanding: Feedback also fosters a deeper understanding of problem-solving methods. When a candidate hears different perspectives on the same problem, it broadens their repertoire of techniques and strategies.
- Boosting Confidence: Positive feedback in areas well-executed is vital. It builds morale and encourages engineers to face challenges with an affirmative mindset.
Mock interviews and peer reviews are vital steps in the preparation process. They ensure that candidates don’t just learn but also apply and adapt their knowledge in real-time. By embracing this practice, senior software engineer candidates can set themselves apart in a competitive job market.
Preparing for Behavioral Questions
Preparing for behavioral questions is critical when approaching senior software engineer interviews. Unlike coding challenges that focus on technical prowess, behavioral questions dive into your past experiences, soft skills, and how you handle various work situations. Interviewers leverage these questions to gauge how you might fit within the team and the company's culture.
Linking Experience to Technical Skills
When addressing behavioral questions, it often helps to draw links between your past experiences and your technical skills. Interviewers want to see if you can integrate your knowledge effectively in real-world situations. A common framework used here is the STAR method, which stands for Situation, Task, Action, and Result. This structured approach enables you to present your experiences clearly and concisely.
For instance, if you're asked about a time when you faced a major project deadline, you might say:
"In my previous role at XYZ Corp, we had a system upgrade project that was crucial for our operations. Situation: The original timeline had to be compressed due to resource issues. Task: I had to lead the team to ensure we met the new deadline while maintaining quality standards. Action: I organized daily stand-ups, delegated specific tasks according to each developer’s strengths, and closely monitored our progress. Result: We successfully completed the project on time, which led to a 20% increase in system performance and reduced downtime."
This type of structured response not only shows your technical capacity but also showcases problem-solving skills and teamwork, key traits for any senior engineer.
Demonstrating Leadership Qualities
Leadership qualities are another focal point in behavioral interviews. You may be asked to describe your experience leading a project or a team, especially when challenges arise. Good responses can highlight your ability to inspire, motivate, and guide team members through hurdles.
When responding, consider specific instances where you played a pivotal role in leading initiatives. For example:
"During a major product release at ABC Inc., I was tasked with heading a cross-functional team. Situation: We faced differences in opinion regarding technology stack choices that threatened to derail our progress. Task: I needed to mediate discussions and ensure all voices were heard while keeping the team focused on our objectives. Action: I started a series of workshops to align team goals and outline the project vision clearly with everyone’s input. Result: This led to a cohesive plan that the entire team supported, culminating in a successful launch that exceeded expected user engagement."
This not only reflects your ability to lead under pressure, but also your understanding of the importance of collaboration and inclusivity in engineering teams.
Overall, preparing for behavioral questions allows you to present yourself not just as a technical expert, but as someone who can bring leadership, teamwork, and problem-solving abilities to a senior software engineering role.
Final Tips for Success
When embarking on the path to becoming a successful senior software engineer, it’s crucial to focus on the foundational elements that support both technical prowess and effective interview strategies. This section provides invaluable guidance designed to arm engineers with the tools they need to excel in coding challenges and interviews alike.
Through continuous learning and a robust portfolio, candidates can not only prepare for practical coding assessments but also demonstrate their expertise and adaptability to prospective employers.
Continuous Learning and Adaptation
One cannot overstate the significance of continuous learning in the technology field. As tools and languages evolve at breakneck speed, it’s imperative to stay ahead of the curve. The software solutions that are relevant today could be obsolete tomorrow. Here are some compelling reasons why this should be a priority:
- Staying Current: Regularly updating your skillset ensures you are familiar with the latest programming languages, frameworks, and technologies. This is not just about keeping up with trends; it’s about understanding how these changes can impact efficiency and problem-solving.
- Skill Diversification: Broaden your knowledge by exploring new domains such as cloud computing, machine learning, or cybersecurity. This adaptability can be a game-changer in interviews as it reflects your willingness to embrace challenges.
- Engaging with Communities: Joining forums like reddit.com can be extremely advantageous. Interacting with other tech enthusiasts and professionals offers real-time insights into industry practices and common pitfalls to avoid.
Additionally, consider creating a learning schedule. Allocate specific times each week to tackle new concepts or tools. Whether it’s enrolling in online courses or reading articles from britannica.com, staying proactive lays a solid groundwork for your knowledge base.
Building a Personal Portfolio
A portfolio acts as a showcase of your best work. For a senior software engineer, this becomes even more crucial, as it serves to bridge the gap between theoretical knowledge and practical application. Here are key components to consider when crafting a standout portfolio:
- Diverse Projects: Include a mix of projects that reflect your versatility. This can range from web applications built with Node.js to machine learning models using Python. The broader the scope, the more appealing your portfolio will be to potential employers.
- Clear Documentation: Each project should be well-documented. Explain the problem you were solving, the technologies used, and the outcome. This not only highlights your technical skills but also your ability to communicate complex ideas.
- GitHub Presence: An active GitHub repository adds credibility. Regular contributions to open-source projects or personal repos demonstrate continuous engagement with the coding community. Ensure your commits are meaningful and well-commented.
- User-Friendly UI/UX: If your projects involve a user interface, ensure that it’s intuitive. Clean design goes a long way, as it reflects a professional approach to your work.
Remember, a strong portfolio showcases not just your skills but also your passion for technology.
In essence, investing time in continuous learning and crafting a robust portfolio prepares candidates for the rigorous demands of senior software engineering roles. These efforts yield tangible benefits, including confidence, enhanced skills, and a clear demonstration of expertise to potential employers, reflecting both your growth and adaptability in a fast-paced industry.