Comprehensive Ruby on Rails Guide for Developers
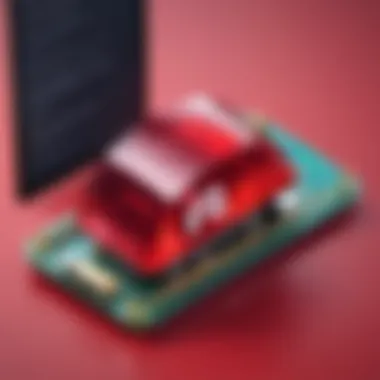
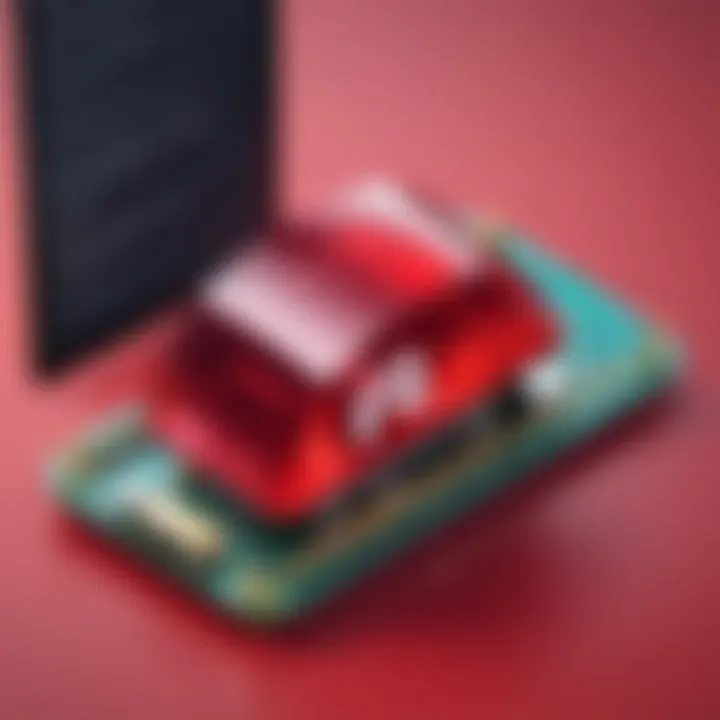
Intro
Ruby on Rails, often simply referred to as Rails, stands tall as a favored framework among developers crafting web applications today. Its prominence remains anchored in the simplicity and elegance of Ruby, which makes building efficient applications a walk in the parkâonce you get the hang of it. In this guide, from the beginner coder fumbling with their first application to the experienced developer polishing up a seasoned project, weâll explore the many facets of Rails.
This thorough dive will cover everything from the nuts and bolts of Ruby to the more advanced methodologies in Rails, helping to illuminate paths for both the curious and the seasoned. The insights shared here are meant to not just teach, but to ignite enthusiasm for what Rails can accomplish. By the end, youâll find yourself not just familiar, but equipped to walk the Rails with finesse.
Whether youâre looking to build a personal project or tackle a compelling business need, Ruby on Rails provides a powerful toolkit packed with functionality and flexibility. Buckle up, and letâs navigate the twists and turns of building robust applications together.
Understanding Ruby on Rails
The realm of web application development has undergone significant transformations over the years, and at the heart of many modern applications lies Ruby on Rails. This section explores why understanding Ruby on Rails is crucial for creating scalable, maintainable, and robust web applications. A solid grasp of Ruby on Rails enables developers to leverage its high-level abstractions and conventions, easing the development process while adhering to best practices. It's not just about writing code; it's about writing better code efficiently.
This framework's versatility means that it can be employed in various contexts, whether for creating a personal blog, an e-commerce platform, or a complex enterprise application. Given its widespread adoption, becoming proficient in Rails also enhances a developer's marketability in a competitive job landscape. This knowledge becomes an invaluable asset, as potential employers place high value on those who can build applications swiftly and reliably.
Prelude to Ruby
History of Ruby
Ruby is not just another programming language; it has a rich history that shapes its present state. Developed in the mid-1990s by Yukihiro Matsumoto in Japan, Ruby was designed to be an enjoyable language for programmers. From its inception, it has emphasized simplicity and productivity. This approach is key when considering the types of applications you can build with Ruby on Rails.
What stands out about Ruby's history is its philosophyâan emphasis on developer happiness. This, in turn, contributes to the language being both powerful and approachable. Furthermore, Rubyâs aesthetics are notably refined, which lets programmers focus more on solving problems than wrestling with syntax.
While thereâs always discussion around performanceâcertain critics say it's slower than competitorsâits appeal lies in many developersâ preferences for clarity over raw speed, especially in early-stage projects.
Key Features
Ruby boasts a few key features that make it attractive for developers. One standout characteristic is its object-oriented nature. Everything in Ruby is an object, which means you can apply methods and properties seamlessly, leading to intuitive designs. Another appealing aspect is Ruby's flexibility with its syntax, allowing developers to express concepts in a myriad of ways without strict rules.
Additionally, Ruby supports dynamic typing, enabling programmers to write less boilerplate code, creating for more agile development processes. However, this dynamic nature does come with trade-offs, as the lack of static typing can lead to bugs that manifest only at runtime, introducing challenges in bigger applications.
Rubyâs Ecosystem
Rubyâs ecosystem is a treasure trove for developers, particularly through its rich set of libraries and frameworks. The community surrounding Ruby is vast and filled with eager contributors. This collaboration has birthed gems like Devise for authentication, ActiveAdmin for admin management, and much more. This open-source environment enhances the development experience by enabling rapid prototyping without needing to create everything from scratch.
What sets Rubyâs ecosystem apart is its commitment to maintaining standards and consistency across libraries, making it a pleasure to work in. However, occasionally, the abundance of choices can overwhelm new developers. Navigating these libraries while understanding which ones align with the current project requirements is an essential skill for effective Ruby development.
Overview of Rails Framework
History of Rails
Ruby on Rails, often just referred to as Rails, emerged in 2004, created by David Heinemeier Hansson. It drew a lot of its strength and philosophy from Ruby but introduced something new: convention over configuration. This means that developers can focus on writing less code while achieving more. It revolutionized the way web applications are built, providing a robust, full-stack solution.
One of the standout features of Rails' history is its rapid adoption. Almost immediately, it garnered a significant following, especially among startups, due to its speed in launching applications. Yet, with growing popularity came the challenge of maintaining quality, particularly as more developers joined the ecosystem. Some early adopters voiced concerns about Rails becoming "too bloated," but updates and community-driven improvements help mitigate these worries.
Core Principles
The core principles of Rails emphasize making development straightforward and efficient. The design philosophy centers around the DRY (Don't Repeat Yourself) and RESTful principles. These not only streamline the development process but also encourage best practices that lead to maintainable code in the long run.
For instance, adhering to DRY helps minimize redundancy, which becomes critical in larger applications. Meanwhile, by following RESTful standards, developers ensure that their applications communicate in a way thatâs organized and predictable. However, mastering these principles requires time and dedication, particularly for newcomers, who must adapt to the nuances of Rails.
Understanding Architecture
Rails is built around the Model-View-Controller (MVC) architecture, a design pattern that separates an application into three interconnected components. This separation simplifies the management of complex applications, allowing developers to keep concerns distinct. The Model handles data and business logic, the View is concerned with the user interface, and the Controller serves as the intermediary between the two.
Understanding MVC is crucial. It promotes organized code, easier troubleshooting, and a clear path for collaboration among team members. However, for those unfamiliar with the concepts, grasping how these components interact within the context of Rails can be daunting, requiring practice and real-world experience to master.
Rails exemplifies the words of Yukihiro Matsumoto himself: "I believe in flexible, productive, and optimized code that allows developers to express their ideas effortlessly."
Setting Up Your Development Environment
Setting up your development environment is like laying the cornerstone of a grand building; without it, you risk structural instability when you start crafting your Ruby on Rails applications. This process is crucial as it determines how efficiently you can write, test, and deploy your code. With the right setup, developers can focus on creating robust applications without the hassle of dealing with software conflicts or versioning issues.
When considering your environment, you'll encounter tools like RVM and rbenv, which help manage Ruby versions. Choosing the right one can ease the installation and maintenance of Ruby, making your journey smoother. Plus, understanding how to verify your installations and handle gems will contribute significantly to your development workflow.
Installing Ruby
Installing Ruby properly is the first step in building your Rails application. Ruby is the language that underpins all Rails apps, and ensuring you have the correct version is vital.
Using RVM
RVM, or Ruby Version Manager, is a widely favored tool among developers. One of the standout aspects of RVM is its ability to manage multiple Ruby environments seamlessly. This feature is particularly advantageous for those who juggle different projects requiring distinct Ruby versions. You can specify the Ruby version for your project right inside your directory, making switching versions no harder than flipping a switch.
However, RVM isnât without its caveats. Some may find it a tad overwhelming at first due to the number of options available. But once you get accustomed, the flexibility it offers is hard to rival.
Using rbenv
On the other end of the spectrum, rbenv offers a more minimalist approach. Its main selling point is simplicity; if you're looking for a lightweight tool that gets the job done without frills, rbenv might be the ticket. It allows you to easily switch Ruby versions within a project, and each project's Ruby version can be defined in a file.
The trade-off, however, is that rbenv doesn't come equipped with as many built-in features as RVM, which could limit some advanced customization options. For developers who prefer straightforward solutions, this can be a blessing or a curse, depending on their needs.
Verifying Your Installation
Once you've got Ruby up and running, itâs a good idea to verify your installation. This step is essential as it ensures everything is functioning as expected. Running a quick command in the terminal can clear up any doubts. Not only does this help in identifying issues right away, but it also serves as a confidence booster.
A unique feature here is that verifying your installation helps confirm that your environment matches your development needs, thus avoiding surprises down the road.
Installing Rails
After setting up Ruby, the next hefty task is installing Rails. This is where all the magic starts happening. Rails is a web application framework written in Ruby, and it's a powerhouse for developing robust applications with less code.
Creating Your First Application
Creating your first Rails application is an exciting leap into web development. This process isnât just about seeing code come to life; it symbolizes the beginning of your journey. The command line provides a simple command to generate a new Rails application, and once executed, it sets up the entire file structure for you with a single line.
This unique feature of Rails is one of its major highlightsâgiving you the scaffolding needed to jump right into development, thereby speeding up your workflow.
Gemfile and Bundler
Once your app is created, letâs pivot to the important topic of dependencies. The Gemfile, in combination with Bundler, manages the libraries your application will use. This arrangement is key for the stability of your application.
When you declare gems in your Gemfile, Bundler ensures that the right versions are installed and loaded. It is a seamless integration that contributes significantly to a well-organized application, shielding you from versioning nightmares.
However, this dependency management requires discipline. Keeping your Gemfile clean and updated can be a chore, but doing so will pay dividends later in your project.
Managing Dependencies
Managing dependencies goes beyond just using Gemfile and Bundler, though they form the backbone. It involves being aware of what libraries your project needs at any given moment and making sure they are properly integrated and updated.
This step is crucial for long-term maintenance and expands your app's capabilities without bogging it down. Regularly reviewing your dependencies leads to a more efficient and secure application. This vigilance allows developers to pivot and adjust as their projects evolve.
Keeping your development environment organized and up to date is not merely a suggestion; it's a necessity for the longevity and success of your application.
With the right installation procedures and dependency management strategies, you'll be set to tackle the exciting world of web application development using Ruby on Rails.
Creating Your First Rails Application
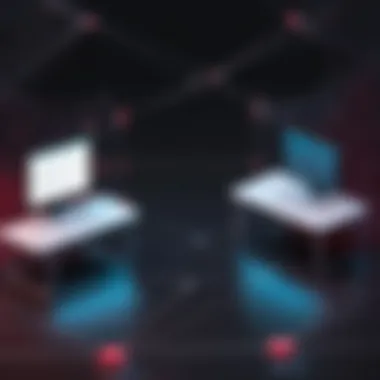
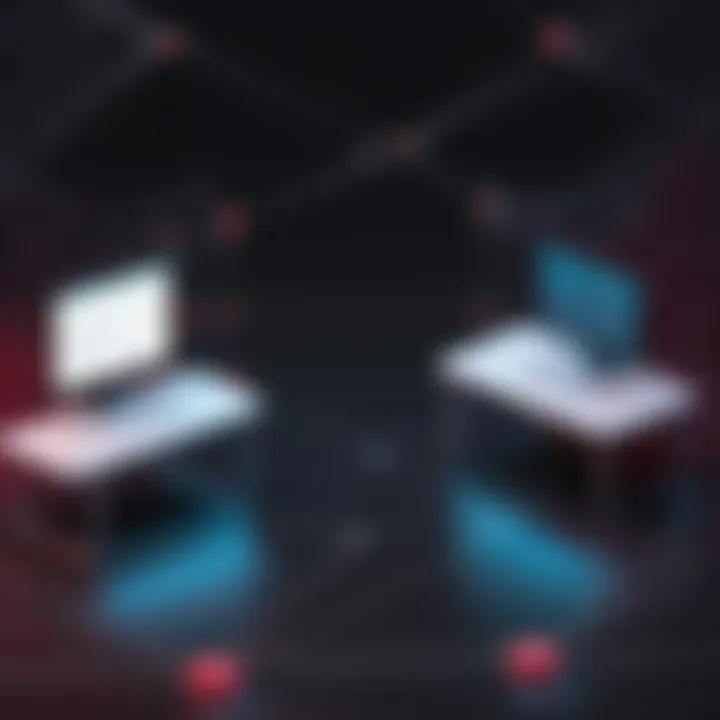
Creating your first Rails application is a significant milestone in your journey as a Ruby on Rails developer. It serves not only as the practical application of the knowledge you've gained thus far but also as a launchpad into more complex ventures. The process of building an application allows you to understand the fundamental components of Rails and their interrelations. From defining routes to creating controllers, every small task you complete adds to your skill set. This section focuses on the importance of various elements involved in the process, the benefits you can gain, and the considerations you should keep in mind as you embark on this venture.
Understanding the Folder Structure
The folder structure of a Rails application is thoughtfully organized, providing clear demarcations of responsibilities. This structure is pivotal for maintainability and scalability.
app Directory
The directory is the heart of your Rails application. It houses models, views, and controllersâcommonly referred to as the MVC architecture. This directory's organized layout allows developers to quickly navigate and modify the application code when needed.
One compelling characteristic of the directory is its division into subdirectories. For instance, youâll find separate folders for models, views, controllers, helpers, and jobs. This modular approach promotes a clean coding environment and is especially beneficial for team projects where multiple developers might be working concurrently.
However, this organization can lead to confusion for novices, especially if they don't follow the Rails conventions. The learning curve can be steep, and newcomers must overcome the initial complexity before harnessing the utility of this structure.
config Directory
Next is the directory, which is often overlooked but immensely crucial. This directory contains the configuration files, which dictate how the application behaves, including database settings, routes, and initializers.
A key feature of the directory is its comprehensive nature. By centralizing configuration settings, it provides developers with straightforward management of environment variables and other settings.
On the flip side, modifying these configurations without understanding their implications can lead to frustrating issues. It's essential to document any changes thoroughly for future reference.
db Directory
The directory's role is chiefly related to database interactions. It includes migration files, seeds, and schema data. This directory is where the database structure evolves as your application grows.
A unique feature of the directory is how it allows you to version your database. With migrations, you can easily modify tables or add new ones while keeping a log of changes along the way. This approach is beneficial for collaboration among teams using version control systems, ensuring everyone is on the same page regarding the database's state.
Nonetheless, unnecessary complexity can arise if migrations are not carefully managed. Developers should be cautious not to pile on too many migrations, as they can become unwieldy over time.
Understanding Routes and Controllers
Routes and controllers are fundamental aspects of how Rails handle incoming HTTP requests and how the application responds. Understanding these components is essential for any Rails developer.
Defining Routes
Creating routes is how you tell Rails which controller to use for a particular request. Essentially, routes act as the traffic signal for your application. They map URLs to controller actions, establishing the application's flow.
A striking characteristic of defining routes is the simplicity it provides. You can declare basic routes with just a few lines of code, allowing for rapid application development and easy URL customization.
However, developers should always ensure they maintain clarity as the number of routes grows. Poorly structured routes can lead to difficulties in debugging and understanding application behavior.
Creating Controllers
Controllers are the bridge between the models and views, handling incoming requests, processing data, and preparing it for presentation. Their creation is a straightforward process with the Rails generators, which means they can be created quickly and cleanly.
A notable benefit of controllers is how they encourage maintainable code. Each controller can handle a specific resource's logic, which makes modifying the application easier as it grows. But be careful; having too many actions in a single controller can make it bloated and difficult to manage.
Action Methods
Action methods within controllers are where the actual logic resides for each response. Each action corresponds to a specific route and performs tasks such as fetching data, applying business logic, and rendering views.
The strength of action methods lies in their encapsulation. By separating each method's logic, you enhance readability and maintainability. But relying too heavily on action methods without breaking things down further can result in complex, hard-to-follow code.
By understanding how to create your first Rails application through these structured components, you set a solid foundation for building efficient, robust web applications. Knowing the intricacies of each directory and component adds considerable depth to your skill set, paving the way for more advanced development in Ruby on Rails.
Building Models and Using Active Record
In Ruby on Rails, understanding how to build models and leverage Active Record is crucial for developing robust applications. Models are the backbone of your applicationâs structure; they interact directly with your database. Active Record, a powerful Object Relational Mapper (ORM), facilitates seamless communication between your Rails models and the underlying database. One of the main benefits of using Active Record is that it allows developers to work with database records in terms of Ruby objects, simplifying CRUD (Create, Read, Update, Delete) operations.
Moreover, adopting this pattern encourages the development of clean, maintainable code, significantly speeding up the coding process. However, efficient modeling also requires precise planning and thoughtful design, which we'll delve into.
Defining Models
Model Naming Conventions
Naming conventions in Rails are not just formalities; they play a pivotal role in enhancing code readability and maintainability. In Rails, model names should be singular, and typically, they follow a convention where the model name corresponds to the table name in the database but in a plural form. For example, a model named corresponds to a table called . This consistency is helpful. It allows developers to automatically predict table names based on the model name and vice versa.
A common characteristic is the camel case style for naming models, which helps distinguish them from controllers and views. Proper naming is crucial as it reduces confusion during collaboration and enhances understanding of the architecture.
A unique feature of following these conventions is that it can make your code self-documenting, promoting better practices and avoiding issues that arise from misnaming or misclassifying. However, straying too far from these conventions can lead to a steep learning curve for new developers on your team.
Creating Migrations
Creating migrations in Rails is a fundamental practice that enables developers to manage changes to the database schema over time. Each migration represents a specific change, such as creating a table or adding a column, allowing for version control of the database structure. Migrations are not only beneficial; they are essential for collaborating on teams where multiple developers may need to make changes concurrently.
One notable characteristic of migrations is that they are written in Ruby, making them accessible and easier to read than raw SQL. This flexibility allows for better documentation of changes as each migration can include comments and descriptions explaining the purpose behind it.
The unique feature of using migrations is that they can be rolled back. Mistakes happen; having the ability to reverse changes keeps your development process agile. The downside? Overusing migrations can lead to a convoluted history if not managed properly, ultimately slowing down the deployment process.
Associations
Associations define the relationships between different models in Rails, such as one-to-many and many-to-many relationships. By establishing associations, developers can easily link data across various tables, which is essential in a relational database. The main advantage here lies in simplifying database queries and making navigation of related data more intuitive.
A distinctive aspect of associations is the ability to chain queries effortlessly. For instance, with an association defined, you can retrieve a userâs posts simply by calling , rather than executing a cumbersome SQL join. This not only enhances the speed of data retrieval but also improves code clarity.
However, associations need careful consideration to avoid too many database calls, which can degrade application performance. Developers should be mindful of the trade-offs, ensuring that the application remains efficient while still maintaining the necessary relationships in the data.
Working with Active Record
CRUD Operations
CRUD operations are the bread and butter of any application, representing the full cycle of data handling. Active Record streamlines these operations, allowing developers to perform them directly on model instances without writing verbose SQL statements. For instance, creating a new record is as straightforward as .
The significant strength lies in clarity; the more intuitive syntax reduces cognitive load for developers, encouraging best practices and quicker development times. However, one must be cautious with bulk operations since they can lead to potential data integrity issues if not handled properly.
Validations
Data validation is another cornerstone of effective application design. In Rails, validations ensure that only valid data is saved to the database. Defining validations on models prevents corrupt data from entering the system. For instance, a validation can enforce the presence of a name in a User model, ensuring every record is ready for processing.
The central advantage of using validations is the clear feedback mechanism it provides. When data doesnât meet defined criteria, Active Record can return informative error messages, allowing users to correct their input easily. However, be careful not to over-validate; excessive validation checks can introduce unnecessary overhead and frustrate users.
Query Interface
Active Recordâs query interface is a powerful feature that allows developers to communicate with the database using a clean and chainable method syntax. Instead of crafting complex SQL queries, you can use methods like to fetch records.
The ease of composing queries is a winning feature, promoting rapid development and consistent code patterns. However, itâs essential to optimize these queries to avoid issues such as N+1 queries, which significantly impact performance. Balancing simplicity and complexity in querying is vital for maintaining a responsive user experience.
With an understanding of models, migrations, associations, and Active Record, you're better equipped to handle the complexities of building robust applications. Each of these elements contributes to a coherent framework that supports efficient data management and enhances the overall quality of your applications.
Developing Views with Embedded Ruby
Developing views using Embedded Ruby is a cornerstone of Ruby on Rails applications. This aspect allows developers to seamlessly integrate Ruby code within HTML, facilitating dynamic web pages that can respond to user interactions and change in real-time. These views are not merely static files; rather, they play a significant role in ensuring that the user's experience is as intuitive and interactive as possible. By leveraging Embedded Ruby, developers can create views that pull data from models effortlessly, rendering content based on the current state of the application.
Templates and Layouts
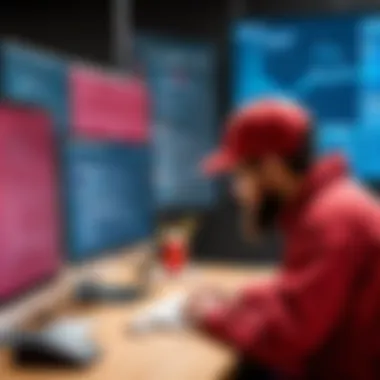
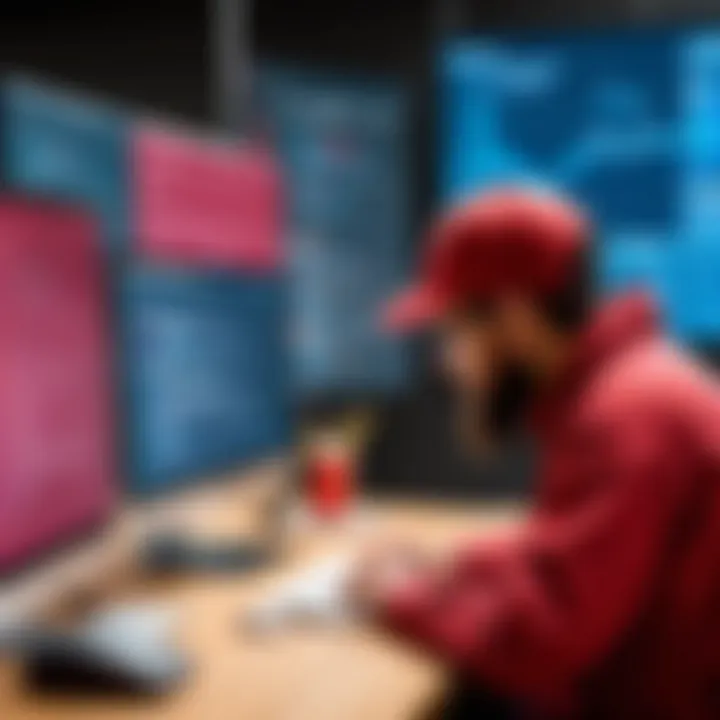
Creating Views
Creating views is essentially about crafting the user interface that is presented to the end-user. It's one of the most direct ways to engage with visitors of a web application. The key characteristic of creating views in Ruby on Rails lies in its simplicity and efficiency. Rails encourages a convention-over-configuration approach, meaning that many decisions are made for you, allowing you to focus more on content and functionality.
One standout feature when creating views is the use of ERB (Embedded Ruby) templating, which allows Ruby code to be embedded within HTML files. This is particularly beneficial because it enables developers to use data pulled from the database directly within the layout. However, one must be cautious about creating overly complex views, as too much embedded logic can make maintenance a chore.
Using Partials
Utilizing partials can greatly enhance the development of views. Partials are smaller reusable templates which can be included in various views. This minimizes repetition and immensely simplifies maintenance, as changes can be made in one place and reflected throughout the entire application. A major benefit of using partials is the streamlining of design consistency across different sections of your application.
The unique feature of partials is their ability to break down complex views into manageable pieces, making code cleaner and easier to maintain. However, developers should be mindful of overusing partials, as it can lead to performance issues if not managed properly.
Layouts and Yield
The concept of layouts combined with the method offers an elegant way to manage the structure of your application's views. Layouts serve as a shell for your views and define the overall structure that your content fits into. The keyword is where the actual view content will be inserted within the layout, allowing for a clean and effective separation of design and functionality.
This approach is beneficial for creating consistent design across various pages, as it prevents the need to repeatedly write the same markup in different views. A disadvantage might come into play if the layout becomes too cluttered, resulting in a less organized structure that could hinder future development efforts.
Form Handling
Creating Forms
Creating forms is a critical aspect of any web application. Forms serve as the primary means for users to submit data, whether it be for creating new records, signing up, or altering existing data. The key feature of creating forms in Rails is the built-in facilities that make handling form data straightforward and systematic. Developers appreciate that Rails helps build forms without excessive boilerplate code.
A unique aspect of Rails forms is their tight integration with models, making form submission a seamless process. However, one must ensure that forms are designed with validation in mind to prevent incorrect data submission.
Form Helpers
Form helpers in Ruby on Rails serve to simplify the process of working with forms. These helpers provide a suite of methods for generating form elements and ensure that they are encapsulated correctly within the application's flow. The key characteristic of form helpers is their ability to automatically handle many attributes and details, saving valuable development time.
While they are a common and preferred choice for many Rails developers, developers should be cautious of excessive reliance on these helpers, as it can sometimes obscure what's happening under the hood, which could lead to debugging difficulties later on.
Handling Form Submissions
Handling form submissions is vital, allowing the application to process and respond to user input effectively. The key here is to manage the data obtained from forms in a way that is both secure and efficient. Rails makes this easy by providing strong parameters and handling submissions with controlled action methods.
The unique feature of Rails in this regard is its emphasis on security through features like CSRF protection, ensuring that form submissions are not susceptible to cross-site request forgery attacks. While this makes forms secure, developers still need to conduct proper validation checks to guarantee data integrity.
Implementing RESTful Services
Implementing RESTful services is a pivotal element in modern web application development, especially within the Ruby on Rails framework. By adhering to REST principles, developers can create applications that are more scalable, maintainable, and user-friendly. In this section, we will dissect various components of RESTful services, including the underlying principles, mapping REST actions, and constructing RESTful routesâeach piece contributing distinct advantages to the overall functionality and efficiency of applications.
Understanding REST
REST Principles
Representational State Transfer, commonly known as REST, emphasizes stateless communication and resource-based architecture. This approach enables different components of a web application to talk to each other effectively, maintaining a separation between client and server. One key characteristic of REST principles is its adherence to HTTP methods like GET, POST, PUT, and DELETE, aligning closely with CRUD operations. This congruity makes REST a popular choice for building APIs in Ruby on Rails applications.
The unique feature of REST principles is statelessness; each request from the client contains all the information needed to process it. This translates into quicker and more reliable interactions between services. Notably, while statelessness can simplify scaling, it may also impose limitations on data that could otherwise be cached for performance.
Mapping REST Actions
Mapping REST actions refers to the process of aligning endpoints with specific HTTP methods to perform operations on resources. This feature of RESTful architecture allows clear and predictable behavior for API interaction. For instance, defining a resources route in Rails using the method provides built-in routing for standard actions, eliminating the need to create multiple routes manually.
A defining aspect of mapping REST actions is the use of resource names in URLs, which contributes to both intuitiveness and SEO-friendly structure. By clearly representing actions through well-defined paths, developers can create more understandable APIs. However, the rigidity of REST actions may pose challenges when custom actions are required, thus requiring additional work to circumvent these limitations.
Building RESTful Routes
Constructing RESTful routes is crucial for linking HTTP requests to the right actions within controllers. This exemplary feature fortifies the principles of REST by ensuring that each route has a clear and direct purpose. Developers can simply generate routes using Railsâ routing DSL (domain-specific language), which reduces boilerplate code and enhances maintainability.
One distinctive advantage of RESTful routes is convention over configuration. Rails encourages best practices through its routing mechanisms, allowing developers to focus on logic rather than configurations. However, developers must ensure that routes remain manageable; overly complex routing structures can lead to confusion and maintenance headaches in the future.
Responding with JSON
As data interchange formats evolve, JSON has emerged as the de facto standard for RESTful APIs. Understanding how to effectively respond with JSON can greatly enhance the client-side experience of Rails applications.
Controlling Responses
Controlling responses involves shaping how data is sent back to the client upon a request. With Rails, this control can be finely tuned, allowing for different formats based on the request or client needs. This flexibility enables developers to provide tailored responses, enhancing the user experience.
One significant advantage of controlling responses is the ability to return data in formats other than JSON when necessary, such as XML or plain text. However, too much variability can complicate an API, making it essential to find a balance between flexibility and clarity.
Serializers
Serializers play a pivotal role in structuring JSON responses from Rails applications. Utilizing serializers allows developers to dictate what data is included in the JSON response, minimizing payload size and allowing for optimized data transfer. This feature is particularly advantageous for mobile applications, where bandwidth may be limited.
The primary strength of serializers is customization, granting developers the freedom to include or exclude attributes as necessary. However, poorly structured serializers may lead to inconsistent data representation across endpoints, so careful design is essential.
Testing APIs
Testing APIs is a critical task that ensures the reliability and correctness of implemented features. Ruby on Rails offers various tools such as RSpec and Minitest to facilitate this testing process. Writing comprehensive tests can reveal potential issues before they become problematic in a production environment.
An essential characteristic of testing APIs is the ability to functionalize checks against various endpoints, verifying that they return the expected status codes and data formats. This process adds another layer of security to applications, helping to prevent regressions and bugs. But create enough tests to maintain robustness without creating an exaggerated test suite that slows down development.
As you deepen your understanding of these concepts, the potential for crafting versatile and powerful applications grows exponentially.
Testing in Rails
Testing in Rails is not just a comfort blanket for developers; itâs more like a compass guiding them through the dense forest of application development. When you dive into testing practices, there are several layers to explore: reliability, maintainability, and efficiency emerge as key players in the game. By verifying that code behaves as expected, you significantly reduce the chances of introducing pesky bugs that could ruin user experience and tarnish your reputation.
Developers now realize that meticulous testing is integral to crafting an application thatâs not just functional but resilient under pressure. This section shines a light on testing frameworks, unit tests, and test structuring, ensuring that your Ruby on Rails applications remain robust and reliable amidst shifting demands.
Testing Frameworks Overview
RSpec vs Minitest
When you throw RSpec and Minitest into the mix, a debate often comes to life among code aficionados. RSpec is celebrated for its expressive syntax, which allows for clear and comprehensive test plans. This characteristic makes RSpec a crowd-favorite, especially amongst those who prefer a behavior-driven approach to testing. How you articulate a feature often feels as crucial as the code itself.
On the flip side, Minitest champions simplicity. It prides itself on being lightweight, making it a good choice for developers who want to keep things straightforward. However, its more concise structure might feel limiting to some, particularly when complex interactions are at play.
In essence, RSpecâs unique feature is its DSL (Domain-Specific Language), which lends itself to readability, breaking down complex tests into more digestible pieces. Minitest, with its no-frills approach, is often favored by those who value speed over verbosity in their test suites.
Installing Testing Gems
Installing testing gems is where the rubber meets the road. It lays the foundation for any testing endeavor in Ruby on Rails. Gems like or streamline the process of writing tests, offering a rich set of tools tailored for various testing needs. This ease of setup is a significant selling point, making sophisticated testing patterns accessible to a wider pool of developers.
Central to this is the act of including gems in the Gemfile, then executing a simple command to bundle them in. Once installed, you can write tests and run them seamlessly. The unique feature here is the community support surrounding these gems, ensuring that help is always a click away. Yet, developers must be careful about dependency bloat; too many gems can lead to complications in managing your application's environment.
Structuring Tests
Structuring tests is an art form in its own right. A well-structured test suite leads to faster feedback and makes debugging less of a headache. An organized directory for tests, separating unit tests from integration tests, showcases a disciplined development approach.
A common characteristic of structured tests is the use of naming conventions and clear organization, which enables a developer to locate and understand tests quickly. This not only enhances clarity but also allows for easier collaboration among team members. One unique aspect of this is the arrangement of context blocks in RSpec, which lets you group related tests logically. However, an over-complicated structure can become a double-edged sword, potentially disorienting rather than aiding developers.
Writing Unit Tests
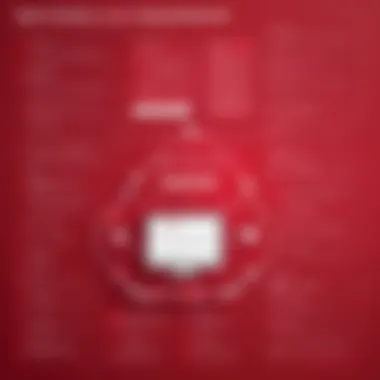
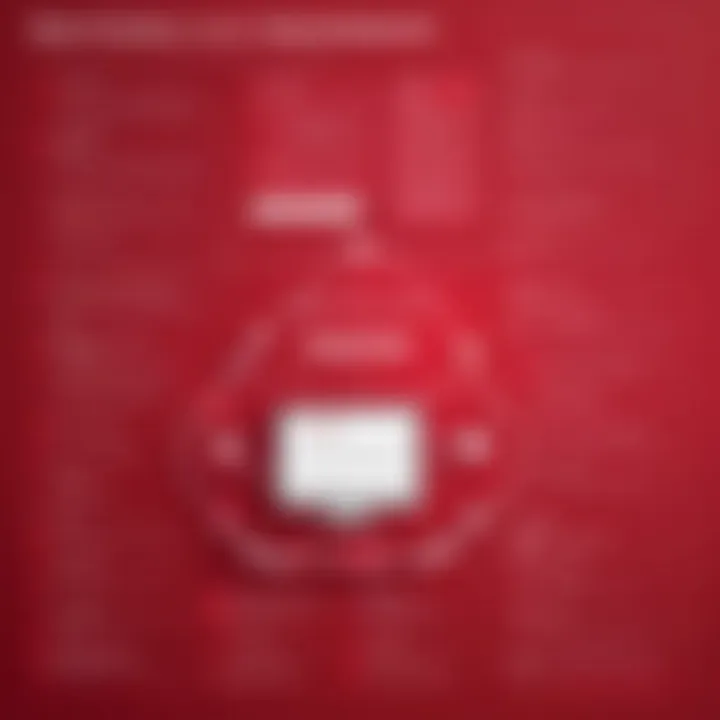
Model Tests
Model tests are the backbone of an effective testing strategy. They ensure that the business logic embedded within the model behaves as intended. Important for maintaining the overall integrity of the application, model tests check validations, relationships, and any custom methods you write.
The key characteristic of model tests is their ability to pinpoint issues at the core of the application. By focusing on the data logic, developers can confidently tweak other aspects of the app knowing their data stays solid. However, one disadvantage is if they become too rigid, they can stifle flexibility, making it harder to adapt the model as your application evolves.
Controller Tests
Controller tests fare well by examining the glue that binds requests to responses. They check whether the controllers correctly handle events and data passing. Due to their emphasis on HTTP interactions, itâs vital for ensuring that users receive the correct pages seamlessly.
A standout feature is the ability to simulate user actions, going beyond just the code to test how the application responds to actual users. The downside? Sometimes excessive focus on controller tests can distract from ensuring the broader application flow works cohesively.
Integration Tests
Integration tests tie everything together, scrutinizing how various components of your application interact. They assess whether different parts communicate effectively, ensuring not just functionality but also a positive user experience.
The main characteristic here is their holistic approach, allowing you to see the bigger picture. However, while they cover extensive ground, these tests can be slower to run, which might test a developer's patience at times.
Deployment Considerations
When youâve crafted your Ruby on Rails application, the next logical step is to enter the world of deployment. This part of the process is not just about getting your app up and running; itâs about ensuring it performs well in a real-world setting. You want it to be accessible to users without a hitch. Understanding deployment is essential, as it encompasses various decisions that directly affect the performance, security, and usability of what you've built. Let's dive into the elements that can significantly impact your deployment journey.
Choosing a Hosting Platform
Picking the right hosting platform is like choosing the right boat for your ocean voyage. Here, the right choice can make your app's performance soar or sink without a trace. Below are some notable platforms:
Heroku
Heroku is often the go-to choice for many developers, especially those just starting out. It provides a platform as a service (PaaS) solution that streamlines deploying applications. One of the primary advantages of Heroku is its user-friendly interface. You can push your code directly using Git, making deployment straightforward.
"Heroku abstracts away infrastructure concerns, enabling developers to focus on writing code rather than managing servers."
This capability allows faster iterations and less downtime during rollouts. However, it may come with a cost; while Heroku offers a free tier to get your feet wet, the premiums for scaling can add up as your app grows.
DigitalOcean
DigitalOcean caters to those looking for more control over their infrastructure without diving too deep into the complexities. It offers cloud computing services that let you scale your resources as needed. DigitalOcean is known for its simplicity and developer-friendliness. Its droplets (virtual servers) are easy to manage, and you can choose from various configurations to suit your needs.
On the downside, the learning curve can be steeper compared to Heroku for those unfamiliar with server management. However, the trade-off is potentially lower costs for higher performance at scale.
AWS
Amazon Web Services, or AWS, is a titan in the cloud hosting arena. It offers extensive services and features that can cater to both small applications and sprawling enterprise solutions. The scalability of AWS is noteworthy; you can start small and grow your infrastructure effortlessly as your application demands increase.
That said, AWS can be overwhelming for newcomers, as the plethora of options requires a significant understanding of cloud computing. Moreover, costs can spiral if not monitored carefully, potentially leaving users with hefty bills if they arenât vigilant about resource usage.
Preparing Your Application
Once you've selected a hosting platform, the next step is to prepare your application for deployment. This aspect can be daunting, but itâs crucial for ensuring that everything runs smoothly in production.
Environment Variables
Environment variables are a foundational block of securing your application. They store configuration settings and sensitive data like API keys without hardcoding them into your source code. This practice is not just a good habit; it helps protect your keys from being exposed to the public.
Using environment variables makes it easy to switch between different configurations for development, testing, and production without altering your codebase, which is quite handy. One downside, however, is that managing environment variables can sometimes become cumbersome, particularly as your project scales.
Database Configuration
Database configuration is another critical element in deployment. In a Rails app, you must specify how your application connects to the database in the file. This includes credentials, host, and environment details.
Proper database configuration ensures seamless data access and integrity, which is vital for your applicationâs functionality. On the flip side, a misconfiguration can lead to performance bottlenecks, data accessibility issues, or even security vulnerabilities.
Asset Management
Proper asset management is essential for a smooth user experience. In Rails, you will utilize the asset pipeline to serve stylesheets, JavaScripts, and images. During deployment, assets should be precompiled to optimize speed and performance.
Failing to adequately manage your assets can create slowdown in your applicationâs load time, adversely affecting user experience. On the plus side, implementing efficient asset management can lead to reduced load times and better performance overall.
By paying careful attention to these deployment considerations, you can significantly enhance the performance and reliability of your Ruby on Rails applications. Taking the time now to ensure smooth sailing can save a lot of headaches down the road.
Best Practices and Optimization Tips
When building applications with Ruby on Rails, adhering to best practices and optimization techniques is essential. It ensures that your codebase remains manageable and your application performs efficiently. With proper guidelines, developers can mitigate risks, address potential problems early, and ultimately deliver a more robust product.
A solid understanding of these practices not only fosters a clearer code structure but can enhance collaboration among team members. Referring to best practices helps avoid misunderstandings and establishes a consistent coding style that every developer can follow.
Moreover, optimization techniques can directly impact loading times and user experience. Applying caching strategies, optimizing database queries, and adopting performance enhancements can lead to significant gains in responsiveness.
In this section, we dive into various aspects, emphasizing code organization and performance optimization while outlining their significance.
Code Organization
Effective code organization is the backbone of sustainable development. Using clear methodologies allows your code to escalate seamlessly and allows others to grasp your logic quickly. It all boils down to a few core approaches.
Modular Code
The concept of modular code refers to writing small, independent units of code. This allows developers to isolate functionalities without crossing wires. A major selling point here is reusability; when you write a function for a specific purpose, you can utilize it across multiple parts of your application.
Advantages include easier debugging and enhanced testing capabilities, as isolated modules can be tested independently. However, if taken to extremes, it may lead to overly fragmented code that becomes difficult to follow. It's a balancing actâfinding the sweet spot between too much separation and too little.
Separation of Concerns
Separation of concerns is crucial in avoiding tightly coupled components within your application. It emphasizes the idea that different aspects of an application should be handled independently. For instance, presentation, business logic, and data access should not interfere with one another, which makes maintenance and modifications easier.
This principle promotes clear delineation, allowing changes in one area without unintended ripple effects. On the downside, this could complicate the architecture if not managed correctly. Striking the right balance can help maintain clarity while steering clear of unnecessary complexity.
Naming Conventions
Having consistent naming conventions ensures that everyone on the team understands the role of each piece of the code. Developers can quickly identify and comprehend code, so they don't waste hours figuring out what something does by simply looking at the names.
Using clear and descriptive names is vital, as obscure abbreviations can lead to confusion down the road. More importantly, sticking to a common naming scheme improves collaboration when working in teams. If you veer too far off the path of naming norms, it may introduce misunderstanding and chaos into your code.
Performance Optimization
Optimizing performance can mean the difference between a fast, responsive application and a slow, clunky one. It's essential to consider how to handle bottlenecks right from the start. Focus on strategies that ensure your application can handle increased traffic without breaking a sweat.
Caching Techniques
Caching techniques can do wonders for application speed. By storing frequently accessed data in a temporary location, you skip the need for repetitive calculations or database fetch requests. Using tools like Redis or Memcached can make significant advancements in speed.
One unique feature is that cached data can be invalidated, meaning that you can refresh the cache to ensure it's up-to-date. However, itâs essential to handle cache properly to avoid issues where stale data gets served, leading to potentially harmful user experiences.
Database Indexing
Database indexing is vital for improving query performance. When you index a database column, you prepare it so that searches can be executed in a flash rather than scanning through every record. This can notably reduce response times and improve user satisfaction.
The upside is that indexed queries execute faster, but over-indexing could lead to increased storage requirements and slower write operations, as the database has to maintain these indexes. Thus, a careful approach to determining which fields to index can go a long way.
Asynchronous Jobs
Implementing asynchronous jobs allows you to offload time-consuming tasks, enhancing the overall responsiveness of your application. By using background processing frameworks like Sidekiq or Resque, you can handle tasks such as sending emails or processing uploads away from the main application thread.
This separation means that users are not slowed down while waiting for these jobs to complete. However, using asynchronous processing requires careful design to manage failures and track job progress effectively.
"Good practices in coding make applications easier to maintain while making them perform better."