Building Web Applications with Django: A Comprehensive Guide
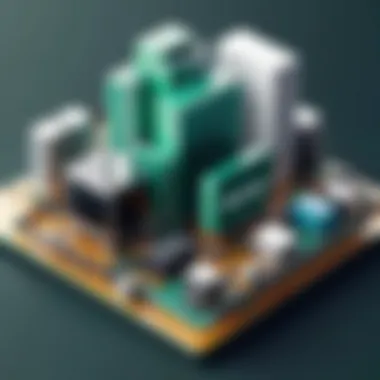
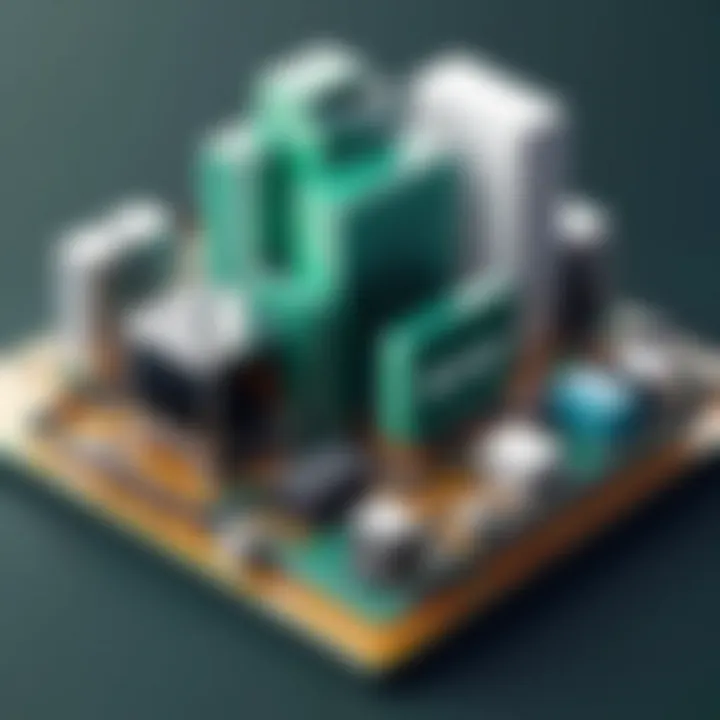
Intro
Django is an open-source web framework built on Python. It provides a high-level structure for building dynamic websites and applications swiftly and efficiently. This guide focuses on the process and best practices necessary for developing scalable web applications using Django.
By following this comprehensive overview, developers, regardless of their experience, can learn the essentials of Django. The framework promotes clean and pragmatic design, which aids in the rapid development of web applications. It is designed with an emphasis on reusability and the principle of DRY (Don't Repeat Yourself). These elements contribute to making Django a popular choice among developers.
In the following sections, we will introduce key concepts around Django, discuss its current relevance in the tech landscape, and provide step-by-step guides to empower developers in their projects.
Intro to Django
Django is a prominent web framework for developing web applications with Python. It plays a crucial role in facilitating efficient and rapid development. As web applications continue to evolve, Django remains a key player, offering a structured approach that enhances productivity. In this guide, understanding Django becomes essential for both beginners and seasoned developers. It enables the creation of reliable, scalable, and secure applications with less effort.
Understanding Django's Role in Web Development
Django streamlines web development through its clear architecture and robust features. This framework tackles common challenges in building web applications, such as database management, user authentication, and security concerns. Its design philosophy emphasizes reducing repetitive tasks, thereby allowing developers to focus on unique aspects of their projects.
Django’s modular structure enables the separation of different components, making it easier to manage and modify. The framework encourages best practices in web development, resulting in clean, maintainable code that can save time and resources.
Key Features of Django
Django is recognized for its numerous features that contribute significantly to web development efficiency. Here are some of the key elements that make Django a preferred choice:
MTV Architecture
The MTV architecture is one of Django's defining traits. It separates the application into three components: Model, Template, and View. This structure ensures a clear division of concerns, which enhances both development and maintenance.
- Model: Represents the data structure. It handles the database and provides an interface for query and manipulation.
- Template: Concerned with the presentation layer. It defines how the data should be displayed.
- View: Acts as a bridge between the model and the template. It processes requests and serves responses to users.
This architecture's main benefit is its modularity, allowing developers to work on different parts of the application independently. This leads to faster development cycles and cleaner code.
Built-in Admin Interface
A standout feature of Django is its built-in admin interface. This tool is automatically generated from the models defined in the application.
- User-friendly: It allows site administrators to interact with the application’s data easily. You can add, edit, and delete content without writing additional code.
- Customizable: While the default interface is powerful, Django also supports custom admin interfaces to fit specific project needs.
This feature simplifies management tasks and reduces the burden of developing a separate interface for administrative purposes.
Robust Security Features
Security is a fundamental component of any web application, and Django excels in this regard. It incorporates several protective measures to mitigate common security threats.
- Cross-Site Scripting (XSS) Prevention: Django automatically escapes sensitive data to protect against XSS attacks.
- SQL Injection Protection: The framework uses parameterized queries, significantly reducing the risk of SQL injection vulnerabilities.
- User Authentication: Django contains a robust authentication system, allowing developers to implement secure user management with minimal effort.
Django’s built-in security features save developers time by relieving them from needing to write custom security logic. However, developers must still adhere to best practices when deploying applications to maintain security integrity.
Django embodies a philosophy of following best practices and adhering to security measures, which accelerates web application development and increases confidence in production environments.
Setting Up Your Development Environment
Setting up a good development environment is crucial for effective Django application development. This stage lays the foundation for all future steps and ensures that everything runs smoothly. A well-configured environment minimizes hurdles, allowing developers to focus on building features rather than troubleshooting. By using the right tools and following secure practices, you can optimize your workflow and foster a more productive coding atmosphere.
Installing Django: Step-by-Step Guide
To get started with Django, installation is the first step. Here's a straightforward guide to help you with this process.
- Install Python: Before installing Django, ensure that Python is installed on your machine. Visit python.org to download the latest version suitable for your operating system.
- Install Django via pip: Open a terminal or command prompt and type the following command:This command downloads and installs the latest Django version from the Python Package Index.
- Verify Installation: To confirm that Django was installed correctly, run:If the installation was successful, this will display the installed version number.
By following these steps, you ensure that Django is available for your projects.
Choosing the Right Tools for Development
Selecting appropriate tools enhances productivity and supports efficient development. Here are three types of essential tools:
Code Editors
Code editors are vital for writing and managing code. A good code editor helps improve your coding experience by providing features like syntax highlighting, code completion, and integrated terminal support. Visual Studio Code is a widely favored choice. Its key characteristic is extensibility, allowing you to add various extensions for Python and Django support. This makes it suitable for current development needs. However, caution should be taken regarding performance issues when too many extensions are active, which can slow down the editor.
Version Control Systems
Version control systems, such as Git, play a fundamental role in managing code changes. They help track modifications, enable collaboration, and safeguard source code. Git has become the go-to solution for developers. A prominent feature of Git is its branching capability, allowing developers to work on features or fixes without affecting the main codebase. Nonetheless, it requires some learning to fully grasp its functionalities, but the long-term benefits of using Git far outweigh the initial learning curve.
Virtual Environments
Virtual environments are essential tools for maintaining project dependencies. They allow developers to create isolated environments for each project, avoiding conflicts between package versions. This is particularly useful when working on multiple projects with differing dependencies. The most popular choice is , which comes pre-installed with Python 3. A significant benefit of using virtual environments is their simplicity and effectiveness. However, managing multiple environments can sometimes become cumbersome if not done systematically.
Consistent organization and proper tool selection can greatly enhance your Django development experience, leading to more successful outcomes.
In summary, a well-set development environment is crucial for effective Django development. Take the time to install and configure the right tools. It will pay off in productivity and smooth workflow, particularly when dealing with Django's robust features.
Creating Your First Django Project
Creating your first Django project is a significant milestone in web development. This process lays the foundation for building robust and functional web applications using Django. Understanding how to initiate a Django project is essential, as it familiarizes you with the framework's structure and configuration. Django's design prioritizes a rapid development environment that allows developers to focus on building functionalities rather than boilerplate code.
When you set up your first project, it is crucial to comprehend the project structure. This understanding ensures you effectively manage your codebase as your application grows. A well-organized structure promotes maintainability and scalability, two key elements of successful web applications. Additionally, the configuration of your Django project impacts how various components interact and work together.
Project Structure and Configuration
Django projects have a specific structure that should be followed for optimal performance and usability. When you create a new Django project, Django generates a default directory layout. This layout includes essential files and directories that facilitate functionality.
Here are the primary components of a newly created Django project:
- manage.py: This script is essential for managing your project. It allows you to run various commands, including starting the server, running migrations, and creating new applications within your project.
- settings.py: This file contains configuration settings for your project. You can define database settings, install applications, and set up middleware, among other configurations.
- urls.py: This module is used to define URL patterns. It maps specific paths to views, allowing you to control what users see when they navigate to different URLs in your application.
- wsgi.py: This file serves as an entry point for WSGI-compatible web servers. It is relevant when deploying your application to a production environment.
Understanding these components helps you make informed decisions as you build your project. It may seem overwhelming initially, but with practice, the structure becomes intuitive and provides a solid foundation for scaling your application.
Understanding Django Apps
Django's modular design encourages the creation of applications within your project. Each application is a self-contained module responsible for a particular function. This modularity allows for better organization, testing, and reusability of code.
In Django, you can create multiple apps within a single project. For instance, if you are building a blog, you might have separate apps for managing posts, user authentication, and commenting systems. This separation of concerns enhances maintainability, making debugging and future development easier.
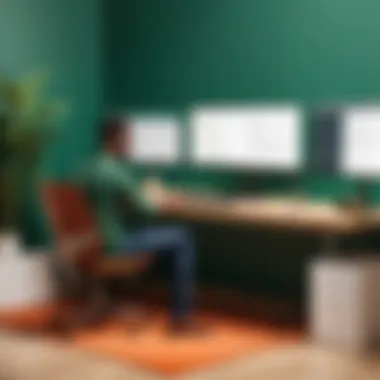
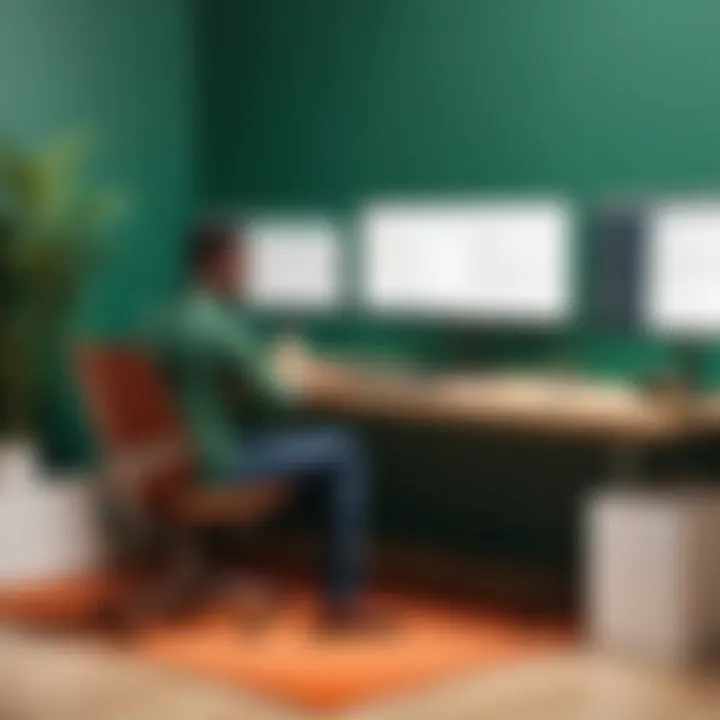
To create an app in Django, you use the command . This command generates a new directory containing several files, including models, views, and tests related to that app. Each app can have its own templates and static files, keeping everything organized.
As you delve deeper into Django development, you'll discover the importance of well-structured apps. They promote collaboration in development teams and behavior can be easily encapsulated.
Modular apps foster an environment where developers can work independently on different features without stepping on each other’s toes.
In summary, creating your first Django project is a critical step in mastering web development with this framework. Grasping its project structure and the concept of applications sets the stage for a fruitful development journey. As you continue to build on these foundations, you will unlock Django's full potential for developing sophisticated web applications.
Database Integration with Django
Database integration is a fundamental aspect of web development, especially when using Django. It allows applications to persist data, essential for most web applications which need to store user information, content, and other dynamic data. The power of Django lies in its ability to seamlessly connect to various databases, enabling developers to leverage robust data management capabilities.
Django uses an Object-Relational Mapping (ORM) system which simplifies the process of interacting with the database. This means that instead of writing raw SQL queries, developers can interact with the database through Python objects. This approach not only improves developer efficiency but also enhances code readability and maintainability.
Models and Migrations
Models in Django serve as the blueprint for your database. Each model corresponds to a table in the database, and the attributes of the model define the columns of the table. By using models, developers can create, read, update, and delete data in a more systematic way. The use of migrations is another significant aspect of Django's database integration. Migrations are essentially version control for your database schema. They allow you to change your database structure without losing data.
Migrations are created automatically when changes are made to models. This validation system is beneficial for development teams, as it helps avoid conflicts and error-prone manual updates. It’s essential to run migrations routinely to ensure the database schema matches the models defined in the code.
Using Django's ORM
Django's ORM is designed to provide developers with powerful tools for managing database records through Python code.
QuerySets
QuerySets represent a collection of database queries. One of the prominent characteristics of QuerySets is their ability to lazy load data, meaning that they only hit the database when necessary. This optimizes performance, particularly in applications where efficient data retrieval is crucial. QuerySets also support filtering and chaining, allowing complex queries to be easily constructed without resorting to raw SQL.
A unique feature of QuerySets is the ability to evaluate them without executing a database query until required. This characteristic can be advantageous, as it helps to reduce server load and enhances the performance of applications. However, caution is necessary; it's easy to inadvertently load more data than intended, which may lead to performance bottlenecks in larger datasets.
Filter and Aggregate Functions
Filter and Aggregate functions extend the capabilities of QuerySets. Filter functions enable the retrieval of specific records that match certain criteria. This method is intuitive and beneficial for isolating data that meets particular conditions, thus saving time and effort in data manipulation.
Aggregate functions, on the other hand, provide a way to perform calculations over a set of values, returning summary data. This aggregate capability is critical for generating reports or visualizing key metrics, further enhancing the decision-making process. The unique aspect of these functions is their ability to operate directly on QuerySets, streamlining the development process. However, they can lead to complexity when dealing with large datasets or when making numerous aggregations at once.
Handling User Authentication
User authentication plays a critical role in web applications. It is the process of verifying the identity of users and managing their access to resources. Proper handling of user authentication is necessary to protect sensitive data and ensure a secure experience for users. A well-designed authentication system can enhance user trust and engagement with the application.
Built-in Authentication System
Django offers a robust built-in authentication system. This system simplifies the process of user registration, login, and session management. The built-in features include:
- User model with fields for username, password, email, and more.
- Support for password hashing, making it secure against common vulnerabilities.
- Functions for user authentication, logout, and user account management.
Implementing Django's built-in authentication system is straightforward. You can utilize built-in views like , , and to create user interfaces. By using these views, you can quickly add authentication features to your application without creating custom solutions. This not only saves time but also reduces the potential for security issues because you leverage well-tested components.
Code Example
Here's a brief example of how to implement a login system in Django:
This snippet demonstrates a simple login function where user credentials are checked against the stored data. If the user is valid, they are logged in and redirected.
Custom User Models
In some cases, you need more than the default User model provided by Django. Custom User Models allow for the creation of a more tailored authentication mechanism. This can be useful in scenarios where you need to store additional user information or change how users identify themselves, such as using an email address instead of a username.
Benefits of Custom User Models:
- Flexibility: Tailor the user model to suit your application's requirements.
- Scalability: Easily accommodate future changes without modifying existing database schema extensively.
- Compatibility: Custom models can integrate with Django's built-in features smoothly.
To create a custom user model, ensure it subclasses or . Configure settings to refer to your user model.
Example of a Custom User Model
Here’s a simple custom user model that utilizes email for authentication:
In this example, the model replaces the username with email as the primary identifier. This kind of customization provides more control over the authentication process and user data security.
Properly handling user authentication in Django is crucial for maintaining a secure, efficient, and user-friendly web application.
Overall, the management of user authentication, whether through the built-in system or custom models, is indispensable to the reliability of Django applications. Nailing down these aspects early can prevent future complications and security risks.
Building User Interfaces with Django
Creating engaging and user-friendly interfaces is crucial in web development. Django provides robust tools to build effective user interfaces that enhance the overall user experience. This section discusses key aspects of building interfaces, particularly through templates and CSS frameworks.
Django Templates
Django templates are integral to rendering HTML pages. They allow developers to separate design from logic. This separation is important for maintaining clean code and facilitating easier updates.
Template Inheritance
Template inheritance is a powerful feature in Django. It lets developers create a base template which can be extended. This method is popular because it promotes reusability and consistency across different pages of a web application.
By utilizing template inheritance, developers can define a standard structure — like headers, footers, and navigation bars — in one location. The key characteristic of template inheritance is that child templates can override blocks defined in the parent template, allowing for specific content without duplicating the entire HTML structure.
Advantages of template inheritance include reduced redundancy and faster updates. When a change is needed, developers modify the parent template, and those changes propagate to all child templates automatically. However, one must be cautious about creating overly complex inheritance chains, as this can lead to confusion in the code.
Static Files Management
Managing static files effectively is another key aspect of Django templates. Static files include images, CSS, and JavaScript. Proper management of these files ensures that they are easily accessible and correctly linked within your web pages.
Django’s static files management feature provides a clear and organized way to serve these files. Its key characteristic is the static files app, which collects all static files into a single location, simplifying deployment. This approach is beneficial, as it reduces the possibility of missing files during the production process.
However, one challenge developers might face is caching. When files are updated, ensuring that users get the latest version can be tricky. Using versioned file names can help mitigate this issue, enabling browsers to fetch the latest files.
Integrating CSS Frameworks
CSS frameworks simplify the styling process for web applications by providing pre-designed components and a consistent grid system. They can significantly speed up development time and ensure a cohesive design.
Bootstrap
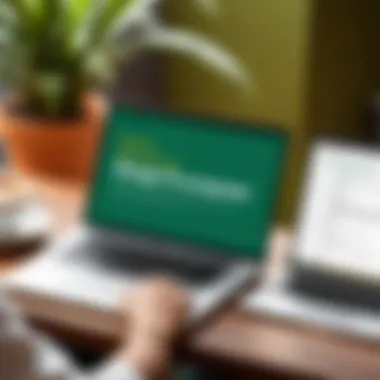
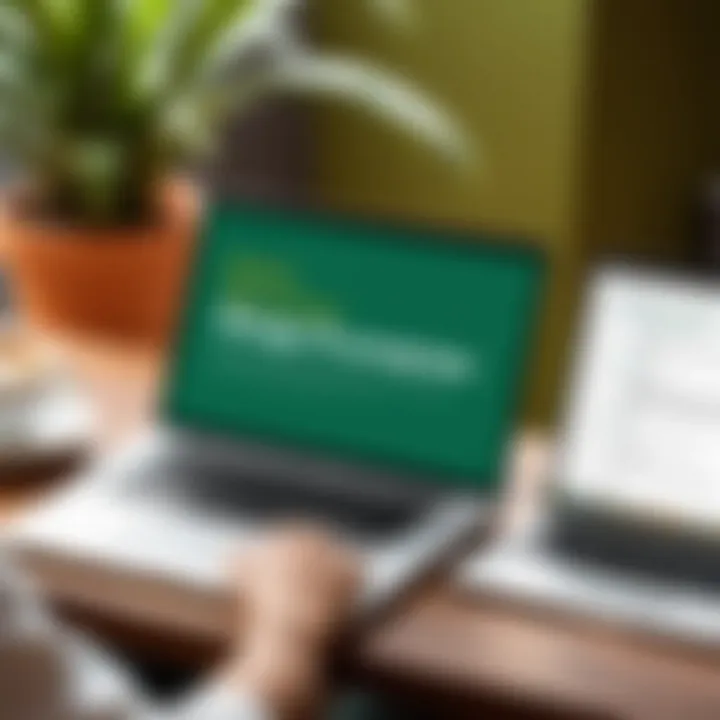
Bootstrap is one of the most popular CSS frameworks. It offers a responsive grid, components, and utilities that are easy to customize. The framework’s key characteristic is its mobile-first design philosophy, allowing developers to design for smaller screens first and then enhance for larger devices.
Its advantages include a comprehensive range of components, from buttons to modals, which can dramatically reduce development time. It also has extensive documentation, making it accessible for both beginners and experts. However, a potential downside is that many websites end up looking similar if the default Bootstrap styles are used without customization.
Tailwind CSS
Tailwind CSS takes a different approach than Bootstrap. It is a utility-first CSS framework, meaning it encourages developers to build designs directly in their HTML by applying utility classes. This style promotes rapid prototyping and helps achieve unique designs without writing custom CSS.
The key feature of Tailwind CSS is its flexibility and support for customization. Developers can easily adapt it to their needs. Moreover, it can lead to fewer context-switching moments since HTML and CSS styles coexist. However, the downside might be an increase in HTML file size due to numerous utility classes. Managing these classes also may become complex as applications grow in size.
In summary, both Bootstrap and Tailwind CSS offer distinct advantages for integrating CSS into Django applications, and the choice largely depends on the specific needs of the project.
Django Rest Framework for APIs
Building applications that communicate effectively with other services is critical in modern web development. Django Rest Framework (DRF) offers a robust framework for building Web APIs in Django. Its significance in this article is twofold. First, it enhances the capabilities of Django to serve as a backend framework that is not just limited to rendering HTML pages but extends to serving data in various formats. Second, it provides a structured and simplified way to expose your business logic and data structures to the web, facilitating mobile applications and third-party integrations. DRF stands out due to its efficiency and flexibility, empowering developers to create RESTful APIs with ease.
Setting Up Django Rest Framework
Setting up Django Rest Framework is a straightforward process that enhances your Django project with powerful tools for creating APIs. To begin, ensure that you have a Django project ready. Then, you can install DRF using pip. Here’s a simple command:
After installation, you need to add to your installed apps in the file of your Django project:
Furthermore, configuring DRF involves setting up permissions and authentication methods that best fit your application requirements. It provides a default permissions system but can be customized extensively. This flexibility allows you to fine-tune how users interact with your API. Additionally, to properly utilize the serializer classes, you can define them in a serializers.py file contained within your app directory.
Creating Endpoints and Serializers
Endpoints are the access points where users or systems can interact with your API. To create an endpoint in DRF, you typically define a viewset and correlate it with a URL in your file. A simplistic example would demonstrate this:
By adding the corresponding routes in , you create a clear mapping for HTTP requests. Here’s how you can register your viewset with a router:
Serializers play a crucial role in transforming complex data types, like Django QuerySets, into native Python data types that can then be easily converted to JSON format. They also validate incoming data. To define a serializer, you can create it similar to this:
Using DRF simplifies the creation of APIs significantly. The built-in features save time while allowing for scalability in function and the ability to manage complex data interactions efficiently. With decision makers trending towards API-based architectures, DRF remains a vital tool in any Django developer's skill set.
"Django Rest Framework is designed to work well with a range of authentication and permissions policies, providing a highly productive way to build web APIs seamlessly."
By mastering these aspects, developers can leverage DRF to turn their Django applications into powerful service-oriented architectures.
Testing Django Applications
Testing is a vital phase in the development cycle of Django applications. It serves several key purposes, ensuring that the application functions as intended, remains stable, and provides users with a reliable experience. Implementing tests helps in identifying bugs early, which can save both time and resources during the development process. It also offers confidence in the application after any updates or changes, guaranteeing that new code does not break existing features.
Benefits of testing are numerous:
- Improved Code Quality: Regular testing promotes a structured approach to coding, resulting in cleaner and more maintainable code.
- Early Bug Detection: By running tests frequently, developers can catch bugs before they become more substantial issues, reducing the overall cost of fixing them.
- Documentation: Well-written tests can serve as a form of documentation, illustrating how the application is supposed to behave and what each component does.
- Facilitate Refactoring: Testing allows developers to make changes with greater assurance. This assurance makes it easier to refactor and improve the codebase without fear of accidentally introducing new bugs.
Testing is not just an afterthought; it should be integrated into the development workflow from the beginning. As applications become more complex, testing ensures that the functionality remains intact across various updates and changes.
Unit Testing in Django
Unit testing focuses on testing individual components or functions in isolation. In Django, unit tests are designed to cover the minimal units of code, ensuring each part works as expected. Django provides built-in support for unit testing, offering tools like the class, which simplifies the setup and execution of tests.
To begin unit testing in a Django application, follow these steps:
- Create a Test Module: In each app, create a file if it does not exist already.
- Write Test Cases: In the , define test classes inheriting from . Each method within these classes should begin with "test_" to denote that it is a test.
- Use Assertions: Django's test framework provides several assertion methods, such as , which can help verify that expected results match actual outcomes.
- Run Tests: Use the command to run all the tests in the application.
Example of a simple unit test for a Django model:
This example demonstrates how to create a model instance and ensure that it holds the expected properties. Unit tests like this enable developers to ensure every component behaves correctly, contributing to the overall stability of the application.
Test Automation and Best Practices
Test automation refers to the practice of running tests using scripts, which helps streamline the testing process. Automating tests leads to more efficient development cycles, as tests can be run quickly and regularly, particularly after each code change. This makes it easier to maintain code quality over time.
Some best practices for automated testing in Django include:
- Write Tests First: Adopting a test-driven development (TDD) approach can help clarify requirements and ensure that functionality is developed according to specifications.
- Keep Tests Isolated: Each test should be independent. Tests should not depend on the success or failure of other tests.
- Use Fixtures: To avoid duplicating data in tests, use fixtures to set up common data for tests. This reduces code duplication and keeps tests clean.
- Run Tests Frequently: Integrate testing into the CI/CD pipeline to ensure that tests are run with every build, enabling quick feedback on code changes.
- Document Test Cases: Include comments and documentation to clarify what each test covers and why it is essential. This aids both existing and future developers in understanding the testing strategies employed.
By adhering to these practices, teams can foster a robust testing culture that enhances the effectiveness of their Django applications, facilitating rapid development while safeguarding against potential issues.
Deploying Django Applications
Deploying Django applications is a crucial phase in the development lifecycle. It encompasses various steps that ensure that the application functions properly in a production environment. A well-executed deployment can greatly enhance user experience, application performance, and security.
When transitioning from development to production, developers must consider several factors. These include choosing a reliable hosting provider, configuring the application correctly, and ensuring security measures are in place. Each of these elements plays an integral role in the success of a deployed Angular application.
"A successful deployment blends technologies, processes, and tools that work together seamlessly to ensure stability and performance."
Choosing a Hosting Provider
Selecting the right hosting provider is a foundational step in deploying Django applications. There are numerous options available, each offering unique features and pricing models. Factors such as scalability, performance, and support should be considered.
Here are some popular providers:
- Heroku: Known for its simplicity and ease of use. Heroku supports scaling and automatic deployment from Git repositories.
- DigitalOcean: Offers flexibility and control through virtual private servers. It is suitable for developers looking for cost-effective solutions.
- AWS: Provides a comprehensive suite of services including Elastic Beanstalk for easy app deployment and management.
- PythonAnywhere: A specialized platform for Python applications, providing a straightforward way to host Django apps.
Each choice has benefits and limitations. It is essential to match your application’s needs with the hosting provider’s strengths. A hosting provider must offer a balance between performance and cost, ensuring that the application can handle user traffic efficiently.
Configuration for Production Environment
Once a hosting provider is selected, configuring the production environment is next. This step is vital for optimal security and performance. Important considerations include:
- Environment Variables: Always set environment variables for sensitive information such as database passwords and API keys. Avoid hardcoding them into your application's source code.
- Debug Mode: Ensure that the Django application is in production mode, meaning that should be set to . Enabling debugging can expose sensitive information to users.
- Allowed Hosts: Define the setting. This helps prevent HTTP Host header attacks by specifying which domains are valid.
- Static and Media Files: Configure serving static and media files efficiently, often through a Content Delivery Network (CDN) for faster access.
- Database Configuration: Migrate the database to a production-ready solution. Ensure that database connections are secured and optimized for performance.
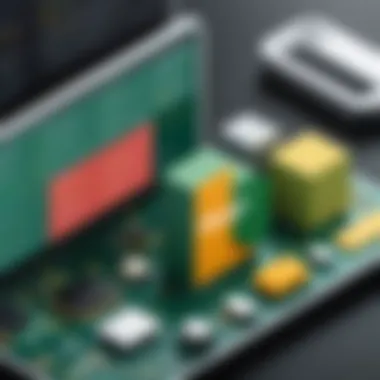
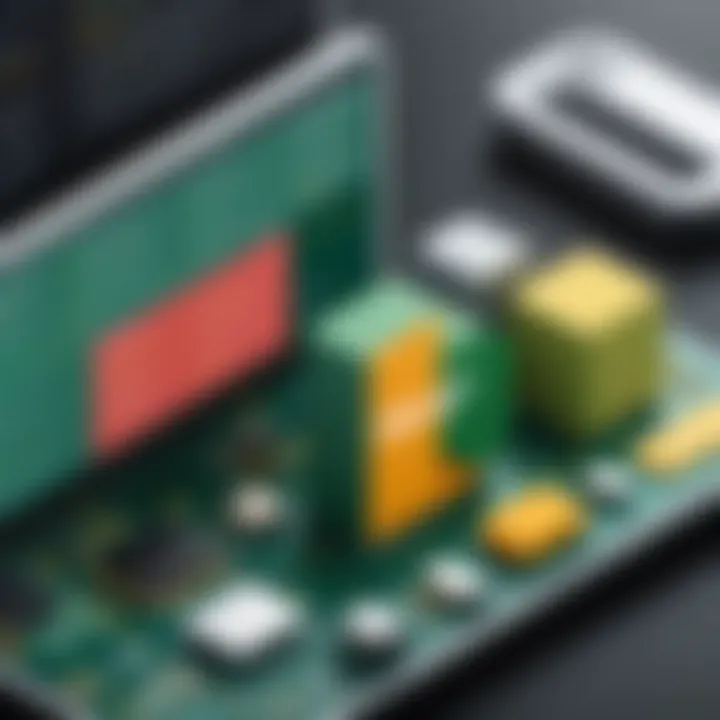
Following these guidelines will lead to a robust and secure deployment. Through careful planning and execution, Django applications can achieve greater reliability and efficiency in the live environment. The deployment stage is more than just moving code; it’s about ensuring that all systems work harmoniously for an optimal user experience.
Maintaining and Scaling Django Applications
Maintaining and scaling Django applications is crucial for their long-term success. As web applications grow, their requirements evolve. This section explores how to effectively manage these applications and ensure they can accommodate increased traffic and complexity. The benefits of this topic can directly influence user experience and operational efficiency.
Monitoring Performance and Logs
Effective monitoring of performance and logs is essential for any Django application. Regularly checking logs helps detect issues early, minimizing potential downtime. Performance monitoring tools can track response times, request rates, and other vital metrics. By keeping an eye on these statistics, developers can identify bottlenecks in their applications. This step is vital for maintaining the health of the application.
Consider these key benefits of performance monitoring:
- Proactive Issue Resolution: By spotting potential problems, developers can address them before they escalate.
- User Experience Optimization: Ensuring the application runs smoothly impacts overall user satisfaction.
- Resource Management: Monitoring resources used by the application can lead to better allocation and cost efficiency.
To effectively maintain your Django application, continuous performance monitoring is non-negotiable.
Scaling Strategies
Scaling strategies determine how well an application can handle increased loads. Effective scaling involves utilizing various techniques to accommodate growth without sacrificing performance or user experience.
Load Balancing
Load balancing distributes incoming traffic across multiple servers. This practice enhances application availability and reliability. By preventing any single server from becoming overwhelmed, load balancing ensures that the backend systems perform efficiently.
Key characteristics of load balancing include:
- Traffic Distribution: This creates redundancy. If one server fails, the load balancer redirects traffic to other servers.
- Enhanced Performance: Balancing workloads can lead to faster response times and higher throughput.
Load balancing emerges as a popular choice for managing growing applications. Its unique feature is its ability to maintain service availability, even under heavy loads. However, it may introduce additional complexity in configuration and management.
Caching Solutions
Caching solutions significantly improve application efficiency by storing frequently accessed data. When users request this data, the application retrieves it quickly from the cache rather than querying the database every time. This speeds up performance considerably.
Key characteristics of caching solutions:
- Faster Data Retrieval: Reducing database queries leads to quicker load times for users.
- Scalability: By alleviating pressure on the database, it allows for larger user bases without degrading performance.
Caching solutions are a beneficial choice as they enhance responsiveness. The unique feature of caching is its capacity to lower server load. However, it may introduce challenges when data needs to be kept current, especially in dynamic applications. Maintaining cache consistency can become a delicate issue.
Common Challenges in Django Development
Building applications with Django comes with its own set of challenges. While Django simplifies many aspects of web development, it is not devoid of hurdles. Understanding these challenges is crucial for any developer aiming to create efficient and effective applications. This section sheds light on common difficulties faced during development, focusing on debugging issues and managing third-party packages.
Debugging Common Issues
Debugging is an inevitable part of the development process. Django provides robust tools to help developers identify and fix problems, yet these tools may still leave some developers feeling overwhelmed. Common issues may include broken links, database connection errors, or template rendering problems. Taking a systematic approach to debugging can be beneficial.
Key points to consider includes:
- Error Logs: Django's built-in logging framework helps capture errors. Familiarity with logging configurations can improve issue resolution efficiency.
- Debug Mode: Enabling the debug mode during development provides detailed error messages. However, it is crucial to disable it before deploying any application.
- Using the Shell: The Django shell allows testing individual components in isolation. This can help quickly identify which part of the codebase is contributing to an issue.
It’s also essential to stay informed with the community forums. Sites like reddit.com can provide insights from peers who have faced similar challenges.
Handling Third-party Packages
Third-party packages can greatly enhance the functionality of a Django application. However, integrating these packages is not always straightforward. They may not be compatible with the latest version of Django or could introduce additional vulnerabilities.
Consider the following when managing third-party packages:
- Compatibility Checks: Ensure any package you plan to use is compatible with your Django version. This will minimize potential issues and save development time.
- Security Concerns: Review the package’s source code if possible. Check for recent updates and user reviews to gauge reliability and security.
- Package Maintenance: Choose packages that are actively maintained. This indicates a better chance for updates, support, and bug fixes.
Using pip to manage dependencies can simplify this process. An example command for installing a package is:
Future Trends in Django Development
Understanding the future trends in Django development is essential for developers aiming to maintain their edge in a competitive landscape. Given the evolution of technology and its impact on web development, being aware of emerging trends can significantly influence the direction of projects and innovations within Django.
These trends not only shape the way applications are built but also enhance the overall efficiency and security of projects. Developers who keep pace with these changes can respond more effectively to user needs and industry demands. This section outlines key considerations for future developments in Django, providing an insight into what lies ahead in this dynamic framework.
Integration with Emerging Technologies
Django’s adaptability plays a central role in its potential integration with emerging technologies such as artificial intelligence, machine learning, and Internet of Things (IoT). The incorporation of AI and ML into web applications can allow developers to create more personalized user experiences.
- Machine Learning Models: Using Django, developers can deploy machine learning models seamlessly, leveraging the framework's capabilities to handle complex data operations. Libraries like TensorFlow or Scikit-learn can be integrated into Django applications, allowing for real-time predictions and insights.
- IoT Applications: As IoT devices proliferate, Django can be utilized to manage data flowing from various sources effectively. It provides the RESTful API capabilities necessary for communication between devices, aiding developers in creating robust connectivity solutions.
- Cloud Technologies: The rise of cloud computing means that Django applications can now be deployed in scalable environments. Integrating services like AWS or Google Cloud Platform enhances data management and offers improved performance.
This integration facilitates a more comprehensive application development experience and meets the growing demands for smart features in web applications.
Community Contributions and Ecosystem Growth
The strength of any framework often lies in its community. Django benefits from a vibrant community that continually contributes to its ecosystem. This is crucial for developers, as community contributions encompass plugins, packages, and active discussions that can enhance development processes.
- Open Source Contributions: The Django community thrives on collaboration. Many developers contribute to the core framework and external libraries. This spirit not only fosters innovation but also allows developers to access a wealth of resources and support.
- Documentation and Tutorials: As more developers engage with Django, the availability of high-quality documentation and tutorials increases. This aids newer programmers in learning best practices and reduces the learning curve associated with the framework.
- Content Creation: Blogs, forums, and platforms like Reddit serve as essential avenues for knowledge sharing. Developers often post their experiences, performance tips, and challenges they face, enriching the collective expertise surrounding Django.
"Django's evolution is not just about code; it's about the community that drives it forward."
By recognizing these key trends, developers can prepare for advancements within Django and position themselves for success in future web application development.
Culmination
The conclusion serves as a pivotal section in any comprehensive guide. In this article, it synthesizes the core topics discussed around building web applications with Django. The focus is not only on summarizing the content but also on reinforcing why each aspect is critical for developers, whether they are beginners or experienced.
The benefits of a thorough conclusion are numerous. It provides clarity, offering the readers a moment to reflect on what they have learned. Moreover, it highlights the importance of understanding key principles in Django. From setting up the environment to deploying applications, each phase adds value to the development process.
Key considerations include how robust the Django framework is for web development. It implies future-readiness as well. As technology advances, understanding how Django integrates with emerging technologies ensures developers remain competitive. It also emphasizes the necessity of keeping up with the community's growth, which continually enhances the capabilities of Django.
"A clear conclusion acts as a bridge to future learning and implementations."
In brief, by emphasizing the importance of each section while concluding, developers can reflect upon their journey through Django development and identify areas for further study.
Summary of Key Concepts
In this section, it is vital to recap the major themes explored throughout the guide. Notably, understanding Django’s core features like the MTV architecture and the built-in admin interface provides a strong foundation. Recognition of Django's ORM capabilities allows developers to manage databases efficiently. Furthermore, handling user authentication and creating user interfaces are paramount aspects. They not only enhance user experience but also reinforce security. Finally, the article covered deploying and maintaining Django applications, highlighting strategies for scalability and performance monitoring. Each key concept builds on the previous ones, culminating in a series of best practices that guide effective development.
Next Steps for Aspiring Developers
For aspiring developers, the next steps should be clearly defined to encourage continuous learning. First, revisiting the concepts outlined in this guide will solidify their understanding. Additionally, experimenting with personal projects can provide practical experience. Leveraging resources such as Django's official documentation and thriving forums like Reddit can expand knowledge. Engaging in community discussions allows developers to learn from experienced peers and explore real-world problems.
Furthermore, contributing to open-source projects enhances not only skill but also recognition within the community. It’s essential to keep abreast of industry trends by following blogs, attending webinars, or joining local tech meetups.
Ultimately, growth in Django development lies in a combination of practice, engagement, and continuous education. Taking deliberate steps now can significantly shape a developer’s future.