Unit Test Software: Comprehensive Insights and Practices
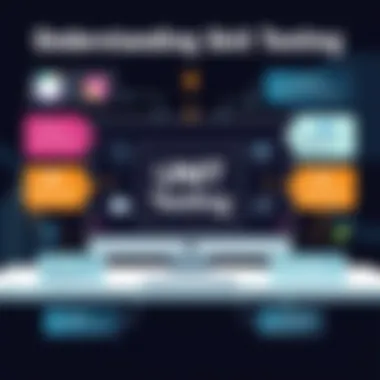
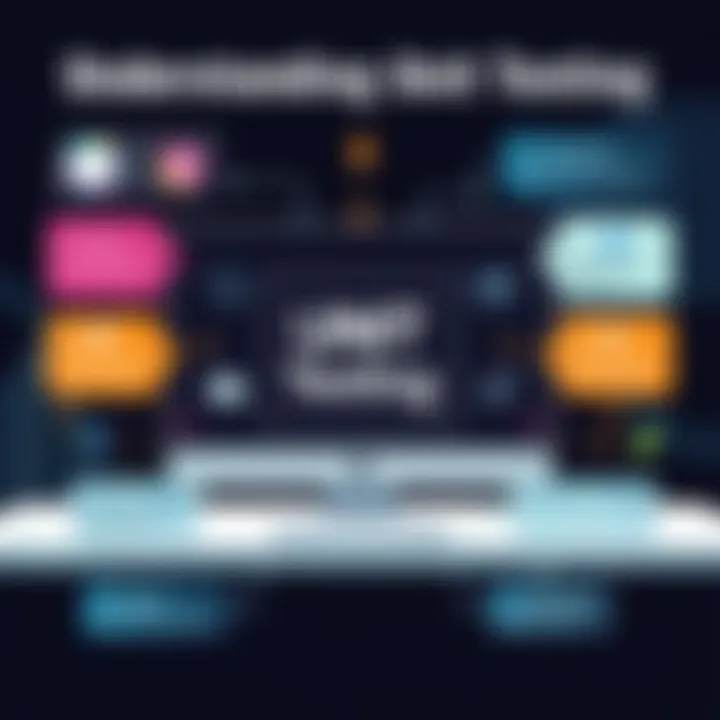
Intro
In the ever-evolving landscape of software development, unit testing stands as a cornerstone practice that ensures the reliability and functionality of code. It may seem small in scale, focusing on the verification of individual components, but its impact on overall software quality is profound. This article offers a deep dive into unit testing, exploring its methodologies, tools, and the critical role it plays in enhancing both the quality of software and the productivity of developers.
By scrutinizing the significance of unit testing, we open the door to a better understanding of how code behaves in isolation and why that matters. Our exploration does not shy away from the tools and best practices that can optimize this essential task, nor does it overlook the challenges faced by developers in an increasingly complex tech environment.
Stay tuned as we unpack everything from the current trends in unit testing to practical guides on tools that can transform your approach to software development.
Foreword to Unit Testing
Unit testing forms the backbone of modern software development methodologies. Without it, the landscape of code validation would be riddled with uncertainty, and the integrity of software products would hang in the balance. This section aims to illuminate the significance of unit testing, shedding light on its definition and its evolution through time, both of which will provide a foundation for further exploration of this essential practice.
Definition of Unit Testing
In the simplest terms, unit testing involves the process of examining individual componentsāor āunitsāāof a software application, ensuring they function correctly. Each unit is tested in isolation from other parts of the application to confirm that it behaves as expected under various scenarios. This practice isnāt merely an academic exercise; itās a vital checkpoint that allows developers to catch bugs at an early stage and validate code reliability.
The crux of unit testing lies in its ability to foster a pragmatic programming environment. By conducting tests regularly, developers can refactor code more confidently. Imagine a scenario where a small piece of code affects the overall system negatively; unit testing can pinpoint the issue before it snowballs into a more significant problem, reducing the time and resources spent on late-stage debugging. Essentially, unit testing ensures that the software's building blocks are solid and ready for assembly into a larger system.
Historical Context
The practice of unit testing has evolved significantly since its inception. Initially, testing was a reactive process, often conducted after code completion, with developers scrambling to fix bugs as they emerged. This method was inefficient and often led to cumbersome code revisions and missed deadlines.
The roots of more structured unit testing can be traced back to the late 1970s and early 1980s, particularly with the advent of methodologies like Extreme Programming (XP). This approach advocated for iterative development and frequent testing, laying the groundwork for unit testing as we know it today. Fast forward to the 1990s, and we see a surge in software testing frameworks that formalized unit testing processes. Tools like JUnit for Java were created, steering developers toward a more disciplined approach to testing their code.
Today, unit testing has become a cornerstone in Agile and Continuous Integration/Continuous Deployment (CI/CD) environments. The emphasis is not just on catching errors but also on creating a culture of quality assurance. In this regard, unit testing is not merely a component of software engineering; it is an integral part of the development lifecycle itself.
"Unit testing is the first line of defense against software bugs. By ensuring each individual component works as intended, we can build more robust applications."
Understanding unit testing's definition and historical context provides us with a vital perspective on its importance. It not only emphasizes a shift from reactive to proactive development but also underscores the necessity of incorporating best practices that evolve with the technological landscape.
The Importance of Unit Testing
Unit testing plays a crucial role in software development, serving as the backbone of quality assurance processes. When you peel back the layers, the benefits of unit testing are evident, influencing not just the code but the overall development workflow.
In this section, we'll delve into the key elements that showcase why unit testing is not just a technical requirement but a strategic advantage in creating robust software solutions.
Quality Assurance
Quality assurance in software transcends mere bug detection; it embodies the discipline of ensuring steadfast performance under varying conditions. Unit testing forms the cornerstone of this process. Each test hones in on an isolated piece of code, guaranteeing that as developers weave complex applications, the fundamental building blocks perform as intended.
By running automated tests frequently, you create a safety net against regressions. A well-tested module can be altered with confidence, knowing that if it breaks, the tests will swiftly point out where the fault lies. Without meaningful unit tests, you gamble with the quality of your product.
"An ounce of prevention is worth a pound of cure."
This timeless proverb captures the essence of unit testing, highlighting the preventive nature of a disciplined testing approach.
Facilitating Refactoring
Refactoring is a natural part of the software lifecycleālike a well-timed haircut, it ensures that the overall shape remains aesthetically pleasing while trimming off the dead weight. However, when faced with tight schedules and evolving requirements, developers often sidestep refactoring out of fear that it may disrupt existing functionalities. Here, unit testing shines.
When robust unit tests exist, developers can refactor code without the nagging fear of breaking something unintentionally. The tests act as a guide, providing essential feedback whenever adjustments are made. By affirming that the desired behavior remains intact, unit tests foster a culture of continuous improvement rather than one of stagnation.
In this way, unit testing encourages developers to embrace change, ultimately enhancing the quality and flexibility of the software.
Reducing Costs in the Long Run
It may seem counterintuitive, but investing time in unit testing early in a project can save hefty amounts down the line. Addressing issues at the unit level is significantly cheaper than discovering them post-release. When bugs are identified during stages of testing rather than after deployment, you dodge costly fixes that typically involve extensive revisions and downtime.
Moreover, the time saved in future debugging efforts mustn't be overlooked. As unit tests become a trusted resource, they streamline the debugging process, letting developers quickly locate sources of failures without sifting through lines of complex code. Less time spent on troubleshooting translates into better resource allocation and ultimately, reduced project costs.
In essence, while the upfront investment in unit testing might seem substantial, the long-term savings and benefits far outweigh any initial concerns, justifying its need in the software development cycle.
Types of Unit Tests
The realm of unit testing isn't one-dimensional; it's a landscape rich with diverse methodologies. Each type of unit test comes with its own set of advantages and challenges. Understanding these types can significantly influence software quality and robustness. Letās delve into the specifics.
White-Box Testing
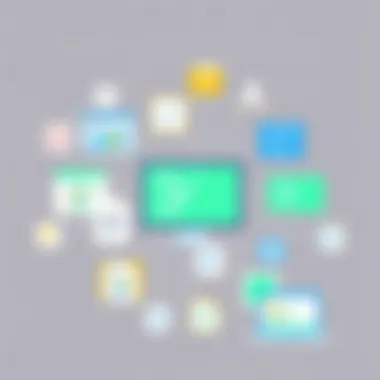
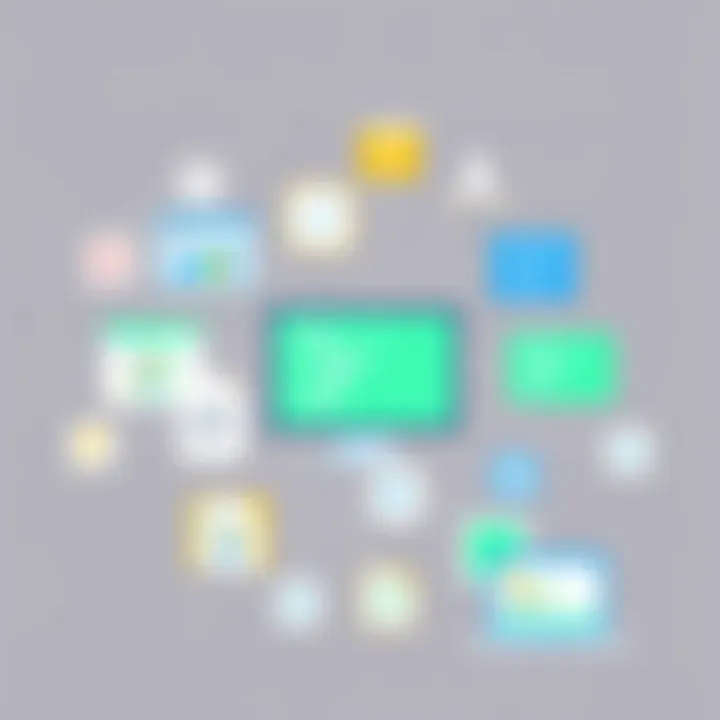
White-box testing, often described as transparent testing, provides a unique perspective on the inner workings of the code. Instead of focusing solely on inputs and outputs, this approach dives deep into the logic, ensuring that all paths through the code are explored.
A substantial advantage of white-box testing is its ability to catch potential errors early in the software development lifecycle. Testers gain insight into how data flows through the code, leading to more thorough tests. Moreover, it often results in better-structured code because developers become more aware of how their actions affect the program's behavior.
However, it does have its hurdles. Crafting effective tests requires in-depth knowledge of the codeās internals, which can be daunting for those unfamiliar with the system. This can potentially lead to maintenance challenges as the codebase evolves. In many cases, white-box testing is paired with automated unit tests to maintain efficiency and accuracy.
Black-Box Testing
Shifting gears, black-box testing takes a radically different approach. Here, the tester is unconcerned with the inner workings of the application. Instead, the focus is placed on inputs and expected outputs. This method simulates real-world interactions, offering a clear picture of how an end-user might engage with the software.
The beauty of black-box testing lies in its accessibility. Testers need not understand how the code is implemented; they simply assess whether the application behaves as expected. This can significantly reduce bias, as the tests arenāt influenced by knowledge of the code. Moreover, since it closely mimics user behavior, it becomes an effective means of validating overall functionality.
Nonetheless, the challenge arises in the form of limited insight into specific code paths. Testers may miss out on identifying certain errors that only reveal themselves via internal logic paths, emphasizing the importance of combining both testing types for comprehensive coverage.
Integration of Unit Tests
Integration of unit tests transcends the individual types of testing. It centers on how various components of the software interact with each other when stitched together, leading to a more holistic quality assurance approach. The integration phase often involves running a suite of unit tests to ensure that independent sections of code not only work well alone but also cooperate without issues.
Successful integration testing identifies conflicts that may not be visible in isolation. For instance, consider a scenario where a new feature is addedāwhile the unit tests for this feature pass on their own, they may introduce unexpected behavior when integrated with existing functions. This underscores the necessity of assessing dependencies in a comprehensive manner.
A well-designed suite of unit tests can dramatically improve the integration process, facilitating smoother implementations and identifying bottlenecks. Furthermore, the practice encourages developers to adopt a mindset that prioritizes modular design, which can simplify future testing and enhancement efforts.
"Unit tests, whether they be white-box or black-box, provide a safety net that can catch issues before they escalate, allowing for smoother workflows and better product quality."
In summary, the types of unit tests play a crucial role in fostering robust software solutions. By employing both white-box and black-box methodologies, alongside effective integration practices, developers can significantly bolster the reliability and efficiency of their code.
Unit Testing Frameworks
Unit testing frameworks are cornerstones in the field of software quality assurance. They serve as structured environments that allow developers to automate the process of executing tests, which can greatly speed up the testing phase and improve overall code quality. These frameworks provide essential functionalities and tools that assist in isolating units of code, verifying their individual behaviors, and ultimately ensuring the software works as intended.
Importance of Unit Testing Frameworks
The implementation of unit testing frameworks is crucial for several reasons:
- Standardization: They create a common standard for writing and running tests, which is particularly valuable in a team setting. This helps maintain consistency across a codebase.
- Automation: Automating tests not only quickens the testing process but also reduces human error, allowing developers to focus on writing better code.
- Feedback: Immediate feedback about the state of the software can guide developers in making informed decisions about their code.
- Documentation: Well-structured tests serve as a form of documentation for the code itself, making it easier to understand its intended functionality.
The awareness and adaptation of these frameworks can help teams stay agile and responsive to the evolving needs of a project.
JUnit for Java
JUnit is arguably the most popular testing framework for Java. Its influence can't be overstated in the ecosystem of Java development as it has initiated a test-driven approach in the community.
Key Features of JUnit:
- Annotations: JUnit uses annotations to simplify the test definition process. For instance, using transforms a method into a test method.
- Assertions: A rich set of assertion methods allows developers to verify the expected results easily, making the documentation of intentions straightforward.
- Test Suites: Using test suites, developers can group related tests, making it easier to manage and execute them as a single entity.
āIncorporating JUnit into your Java projects can significantly enhance your testing efficiency and reliability.ā
The simplicity and effectiveness of JUnit contribute heavily to its widespread adoption, and even new Java developers find it accessible and easy to use.
pytest for Python
When it comes to Python, few testing frameworks have the acclaim of pytest. This framework is known for being easy to learn; yet it is powerful enough to accommodate demands from simple unit tests to complex functional testing.
Noteworthy Aspects of pytest:
- Fixtures: pytest supports fixtures, allowing developers to set up a specific environment for tests, which can keep tests independent and manageable.
- Plugins: The framework has a rich ecosystem of plugins that extend its capabilities, including tools for test coverage, parallel test execution, and more.
- Descriptive Output: It provides detailed error messages which help in pinpointing issues quickly, what can save a lot of time during debugging.
The adaptability and simplicity of pytest makes it a favorite among many Python developers who appreciate its ability to handle both small, focused tests and larger, more comprehensive testing strategies.
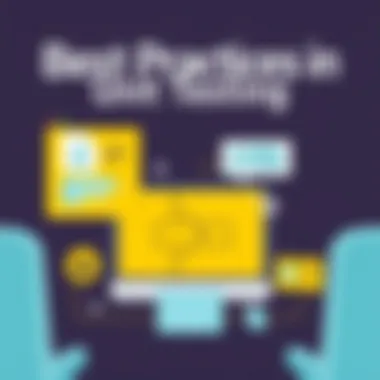
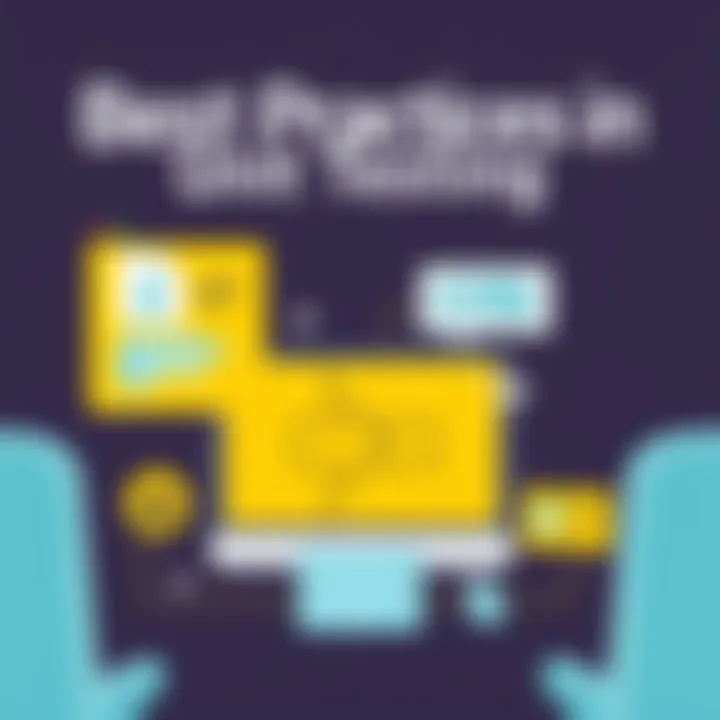
NUnit for .NET
NUnit has become the go-to testing framework for .NET applications. With a robust feature set, it enhances the testing experience and allows developers to write clear and maintainable tests.
Advantages of NUnit:
- Attribute-based Testing: NUnit employs attributes similar to JUnit, allowing for straightforward test method declarations and setups.
- Parallel Execution: Facilitating test cases in parallel can significantly reduce wait time and speed up the feedback loop during the testing process.
- Comprehensive Documentation: NUnit's rich documentation aids developers in understanding the frameworkās features, making the transition smoother.
In summary, each frameworkāJUnit, pytest, and NUnitācarries its unique strengths, aligning closely with their respective programming languagesā ecosystems. As unit testing continues to be pivotal in software development, understanding these frameworks can greatly benefit any programmer aiming to enhance their work quality.
Best Practices in Unit Testing
When it comes to unit testing, certain guidelines stand out as essential for maximizing efficiency and effectiveness. Implementing best practices helps ensure that unit tests do not merely gather dust but actively contribute to the codeās reliability and maintainability. It's not just about writing tests; it's about writing them in a way that uplifts the overall development process.
Test-Driven Development
Test-Driven Development (TDD) is a cornerstone of best practices in unit testing. At its core, TDD flips the traditional programming approach on its head. Instead of writing code and then testing it, you start with the tests. The process is simple:
- Write a Test: Define what you want your code to do.
- Run the Test: Naturally, your test will fail initially since the actual functionality doesnāt exist yet.
- Implement the Code: Now, write the minimal amount of code required to pass the test.
- Refactor: Clean up the code while ensuring the test still passes.
This cycle, often emphasized in agile development practices, can prompt more thoughtful design and a clearer understanding of requirements. It transforms coding into a disciplined activity that proactively guards against regressions. Notably, TDD promotes iterative development, meaning every tiny modification is backed up by tests, allowing for continuous improvements with confidence.
Writing Clear and Concise Tests
Writing tests is not just a ritual; clarity and conciseness should be prioritized. A unit test should clearly assert specific conditions under particular circumstances, making it easy to read and understand. Consider these points:
- Be Descriptive: Use meaningful names for your test cases. Instead of naming a test , opt for , for example.
- Keep Tests Small: Each test should focus on one aspect of the functionality. If the test covers too much ground, it can become difficult to pinpoint what fails.
- Avoid Complex Logic: Tests should reflect straightforward logic. If you find yourself nesting conditions or incorporating loops, itās time to rethink your test design.
Effective tests serve both as checks for the system's functionality and as a form of documentation, guiding the next developer who may traverse your code. Itās common to find that unclear tests can lead to misunderstandings and mismanagement down the line, so avoidance of such scenarios is paramount.
Maintaining Test Suites
Having a collection of unit tests can be a developer's best friend, but maintaining that collection is where the real work lies. Over time, codebases evolve, and your tests must keep pace. Here are some habits to instill:
- Regular Reviews: Make it a point to review tests periodically. Remove those that are redundant or obsolete due to code changes.
- Automate Running Tests: Leverage tools to automate running your test suite regularly. This practice helps surface issues even before they become critical.
- Update with Code Changes: Whenever you alter or add features, ensure corresponding tests are written or updated. Failing to do so often leads to a false sense of security.
"Maintaining your test suite is just as vital as writing the tests themselvesāit ensures your testing efforts continue to yield value."
In a nutshell, the practices of test-driven development, clarity in test writing, and diligent maintenance of test suites not only safeguard software quality but can greatly enhance the productivity of developers. By embedding these principles into the software development lifecycle, the road ahead is not just clearer but also much smoother.
Challenges in Unit Testing
Unit testing is a cornerstone of software development, yet it isn't without its hurdles. Understanding the challenges involved is crucial for developers looking to implement robust unit testing practices. Each challenge presents unique obstacles that can impact code quality and team efficiency. Addressing these challenges not only enhances testing efficacy but also helps in cultivating a more streamlined development process.
Overlapping Tests
One common pitfall in unit testing is the issue of overlapping tests. This phenomenon occurs when multiple tests inadvertently evaluate the same code paths or business logic. Not only does this duplication waste valuable testing resources, but it can also skew test results, making it difficult to pinpoint the origin of an error. When a bug emerges, having overlapping tests can lead to confusion, forcing developers to sift through layers of tests to determine which one actually failed.
To mitigate this, it's essential to take a strategic approach to designing test cases. Developers should carefully outline the purpose of each test and ensure that tests target distinct functionalities or outcomes. This can be managed by:
- Establishing clear naming conventions for tests to indicate their specific goals
- Utilizing test case management tools that highlight dependencies and overlaps
- Regularly reviewing the test suite to identify redundant tests and retire them
By focusing on unique test scenarios, teams can improve the balance between thoroughness and efficiency in their testing efforts, leading to more trustworthy test results.
False Sense of Security
Another significant challenge in unit testing is the false sense of security that comprehensive test coverage can bring. It's tempting to equate a high percentage of covered code with high reliability; however, this assumption can be misleading. Coverage metrics may show extensive testing, but they do not guarantee that all edge cases or failure modes have been adequately addressed.
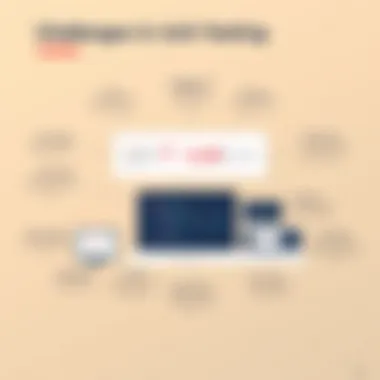
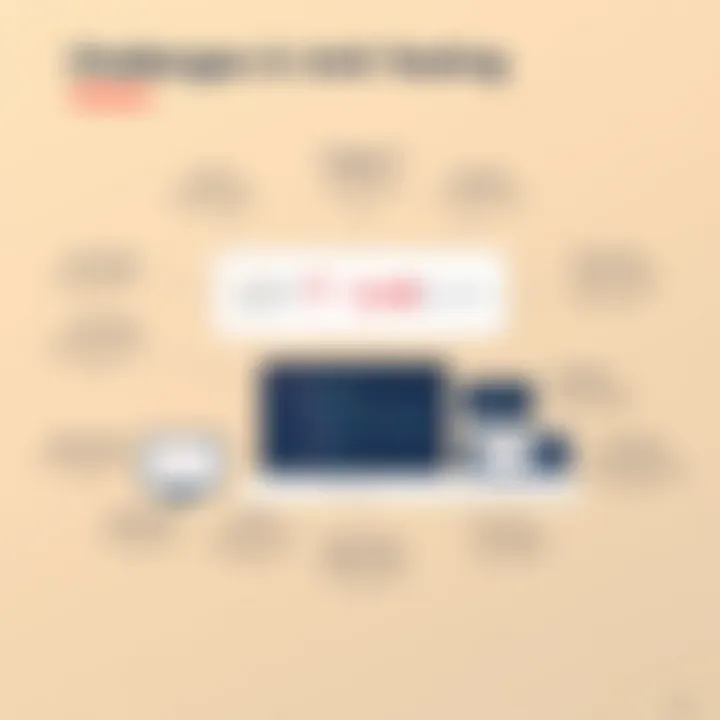
The common adage, "just because something works doesn't mean it's right" rings true in this context. Often, tests can pass while overlooking crucial aspects of functionality, especially when tests are designed with limited scopes. Addressing this issue requires a shift in mentality from solely focusing on coverage numbers to a more quality-driven perspective, emphasizing:
- Designing tests that are not merely thorough but also thoughtful
- Including tests for edge cases that might not be immediately apparent
- Regularly updating tests in tandem with code changes to ensure relevance
The rigor with which tests are conceived can significantly influence the overall reliability and robustness of the software.
Test Maintenance Overhead
Finally, the issue of test maintenance overhead is one that many development teams face. As projects evolve, the corresponding unit tests often require updates, adjustments, or complete rewrites. Maintenance challenges can lead to decreased morale and, if not managed properly, can result in a test suite that becomes a burden rather than an asset.
It's crucial for teams to recognize that maintaining a healthy test suite is as important as writing the tests in the first place. Here are some best practices to ease the burden of test maintenance:
- Invest in training for team members on best practices for writing maintainable tests
- Encourage a culture of code reviews to ensure that unit tests are evaluated for quality and necessity
- Periodically schedule cleaning sessions for your test cases to ensure outdated tests are removed or revised
When test maintenance becomes a priority rather than an afterthought, it can contribute to a more resilient and adaptable testing environment.
"Challenges in unit testing are not just obstacles; they are opportunities for growth and improvement in the development process."
By delving into these challenges with a proactive mindset, developers can refine their unit testing strategies, ultimately leading to higher quality software that stands the test of time.
Future of Unit Testing
The future of unit testing is nothing short of intriguing, as advancements in technology continuously reshape how developers approach their craft. Unit testing, being a vital part of software engineering, must evolve alongside these technological trends. As we discuss its future, elements like automation, artificial intelligence, and integration with other processes become fundamental. Thereās a need to consider not only how we can boost efficiency but also how to maintain quality as projects scale. The benefits of these trends could redefine day-to-day tasks for developers, making testing less of a chore and more of an integral part of the creative process.
Automation and AI in Unit Testing
Automation in unit testing is gaining traction, and itās not just a passing fad. By automating repetitive tests, developers can spend their time on the intricate parts of coding that require human intuition and creativity. Tools that support automation can ensure these tests run quickly and consistently, catching bugs before they snowball into larger issues.
With the advent of AI, we're seeing smarter algorithms that can predict where errors might occur in the code. These systems learn from previous codebases and, as they do so, they can suggest test cases or even automatically generate them based on likely edge cases. In practical terms, consider a scenario where a significant software update introduces new features. An AI system capable of analyzing code changes can highlight areas of concern, minimizing manual review time. This enhances not just efficiency but also the robustness of software deliveries.
The adoption of AI doesnāt mean the end of manual testing; rather, it works in tandem. Teams can leverage AI to identify high-risk areas and focus their manual efforts where theyāll yield the most benefit. Hereās a brief snapshot of the factors driving AI in unit testing:
- Risk Assessment: AI can analyze historical defect data and code complexity to prioritize tests that cover the most critical areas of the software.
- Dynamic Test Creation: Utilizing machine learning, test cases can be generated as new features are added, adapting to the codebase in real-time.
- Continuous Learning: Every time a test runs, results can feed back into the AI system, refining its analysis and suggestions for future tests.
Integration with Continuous Delivery
As development practices pivot towards continuous delivery, unit testing becomes a linchpin in maintaining quality at speed. Continuous delivery emphasizes deploying small, incremental changes regularly, which necessitates that testing be not only thorough but also swift. Here, unit tests serve as the first line of defense, ensuring that every piece of code pushed to production meets quality standards.
Integration requires a seamless transit between unit tests and deployment cycles. Developers can implement frameworks that facilitate automated testing at each stage of the delivery pipeline. Think of this as a conveyor belt: code moves smoothly from development to production, prompted by automated tests checking for potential problems at every stop along the way.
Some critical aspects to consider in this integration include:
- Version Control Integration: Unit tests should be tied closely to version control systems like Git. This ensures that tests reflect the current state of code, minimizing discrepancies.
- Rapid Feedback Loops: Timely feedback during the testing process can catch bugs early on, enabling teams to react quickly.
- Shift-Left Testing: Encouraging developers to write unit tests as they develop features protects against late-stage discoveries of defects, guiding them towards a more quality-focused mindset.
"In the rapid cadence of modern software, unit tests are the safety net that prevent developers from falling into the abyss of unmanagable code."
The road ahead for unit testing will be paved by the commitment to innovation and efficiency. As industry trends align, staying updated with automation tools and strategies for effective integration will be key for developers striving to meet the quality thresholds of tomorrow.
Finale
In the grand scheme of software development, the importance of unit testing casts a long shadow. It's not merely an accessory to coding but a fundamental pillar that upholds the structure of robust software. This article has journeyed through various facets of unit testing, exploring its role in quality assurance, its methodologies, and the tools that facilitate its practice. To wrap things up, letās focus on a few specific elements that encapsulate the essence of unit testing.
Recap of Key Points
Unit testing provides several notable benefits:
- Enhancement of Code Quality: By isolating individual components, developers can address issues early, ensuring a cleaner, more reliable codebase.
- Facilitating Refactoring: As code evolves, unit tests provide a safety net, allowing modifications with confidence, knowing that any potential errors will be flagged.
- Cost Efficiency: Catching bugs during the development phase is often significantly less expensive than addressing them post-deployment. Good unit testing practices contribute to long-term savings.
- Integration in CI/CD Pipelines: Automated unit tests can be seamlessly integrated into continuous delivery pipelines, ensuring that quality is maintained throughout the development lifecycle.
- Boosting Developer Productivity: Unit tests streamline debugging processes, giving developers a quicker turnaround on delivering updates and new features.
In summary, implementing solid unit testing practices lays the groundwork for a more efficient, effective software development process that ultimately produces superior products.
Final Thoughts on Unit Testing
Looking ahead, the future of unit testing seems intertwined with emerging technologies such as AI and automation. These advancements promise to refine testing processes further, making them smarter and more efficient. As industries embrace DevOps and Agile methodologies, the integration of unit tests into every phase of development remains crucial.
Unit testing isn't just a checkbox on the development list; itās the assurance that components function as intended and that every piece of the code puzzle fits snugly in place. While challenges persistāsuch as maintaining a vast suite of tests or managing overlapping test casesāthe benefits significantly outweigh them.