Understanding the React Redux Provider: Concepts and Best Practices

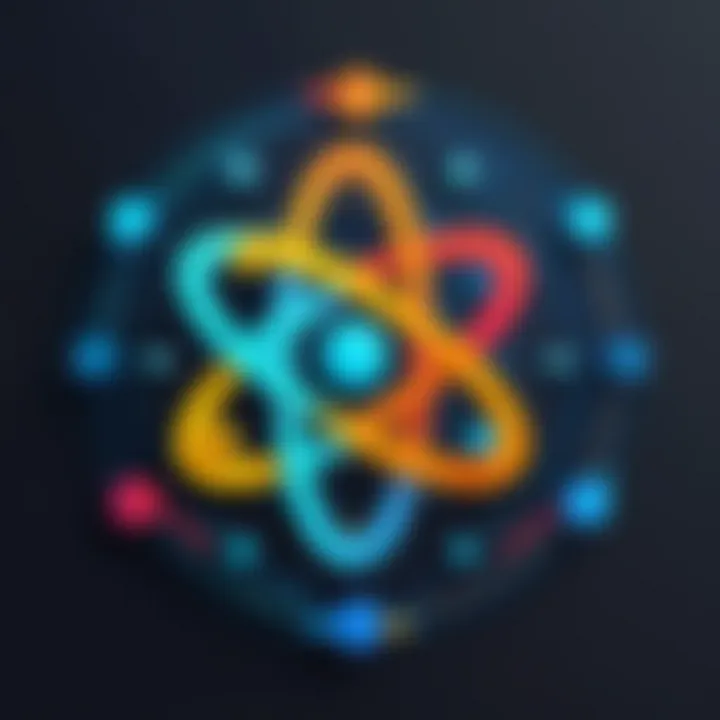
Intro
The integration of Redux with React is vital for managing application state efficiently. At the center of this integration lies the React Redux Provider. It acts as the bridge, connecting the components of a React app with the Redux store. Understanding this component is essential for developers aiming to create scalable and maintainable applications. This section explores its importance, functionality, and how to use it effectively.
The React Redux Provider not only facilitates easy access to the store but also manages component re-renders when the state updates. It encapsulates the store, making it available for any nested components in the application tree. This setup is crucial, especially in complex applications where managing state can quickly become challenging.
Key Points We Will Cover
- The theoretical framework behind the Provider
- Practical application of the Provider in React applications
- Best practices to enhance performance and maintainability
- Common pitfalls and troubleshooting strategies to avoid errors
- Real-world examples illustrating the Provider’s role in state management
Through this exploration, readers will not only grasp how to use the Provider effectively but also appreciate its influence on the overall architecture of a React-Redux application.
Intro to React Redux Provider
The React Redux Provider is a crucial element in the context of modern web development. It serves as a bridge between React, a widely-used JavaScript library for building user interfaces, and Redux, a state management library that allows for predictable state management in applications. Understanding the Provider is essential for developers who seek to create efficient and maintainable React applications that require robust state management.
With the increase in complexity in web applications, effective state management becomes non-negotiable. The Provider component allows React applications to access the Redux store seamlessly, thus centralizing the application’s state management. The benefits of using the Provider include better organization of code, improved performance, and easier debugging. The Provider makes it possible to share data across various components without the tedious necessity of prop drilling, where props need to be passed through multiple layers of components.
Additionally, it's important to consider how the Provider integrates with React’s lifecycle. This understanding can significantly impact the efficiency and reliability of state updates in an application. As we dive deeper into this article, we will explore the foundational concepts surrounding Redux and the role of React in state management, culminating in a comprehensive overview of the Provider component.
Overview of Redux
Redux is an open-source JavaScript library designed for managing application state. Its most notable feature is the centralized store, where all the application’s state is kept. This enables various components to react to state changes efficiently. Redux uses a unidirectional data flow, which simplifies the process of understanding how data moves through an application.
When an action gets dispatched, it describes how the state changes and how it should be updated. The reducer functions take the current state and the dispatched action as arguments to return a new state. This predictable flow makes it easier to track changes and maintain code.
Understanding the structure of Redux is fundamental to effectively working with it. The main parts of Redux include the store, actions, reducers, and middleware. The store holds the state, actions define how the state should change, and reducers specify how this change happens. These concepts provide a clear framework for data management.
The Role of React in State Management
React’s role in state management is pivotal. Within React applications, components often need to display data and respond to user actions. Instead of using local component state exclusively, integrating Redux allows components to share state across any part of the application.
React encourages efficient UI updates with its reconciliation process. When implemented with Redux, components can update when the state in the Redux store changes, ensuring the UI reflects the latest state. This relationship creates a clean separation between UI presentation and state management, making applications more scalable and easier to maintain.
Using hooks such as and , React components can easily interact with the Redux store. This integration enhances performance by minimizing unnecessary re-renders and ensuring that only the components that need to update do so. By mastering the interplay between React and Redux, developers can build highly responsive applications that provide a smooth user experience.
Preface to the Provider Component
The Provider component from the React Redux library is the gateway through which components access the Redux store. By wrapping the entire application in the Provider, components gain access to store data without cumbersome prop passing. The Provider must receive the store as a prop, which sets the context for all nested components.
The Provider plays a crucial role in simplifying state access.
Without the Provider, managing state becomes cumbersome. You would need to manage data flow explicitly through props. The application would soon become tangled and difficult to maintain, especially as nesting deepens. Instead, nesting components within the Provider enables cleaner code and intuitive access to state.
This component is also essential for integrating Redux’s middleware, enabling functionalities such as logging or handling asynchronous requests. Therefore, gaining a deep understanding of the Provider's mechanics is essential for leveraging the full potential of Redux in React applications.
Setting Up React Redux
Setting up React Redux is crucial to ensuring that your application leverages the full potential of React and Redux working in tandem. This section will provide insights into the components needed for such setup, including the installation of dependencies, the creation of the Redux store, and connecting Redux to the React application. Each of these steps will play a significant role in defining how state management occurs within your app. By understanding and implementing these elements, developers can create a robust foundation for their applications, leading to improved maintainability and scalability.
Installing Dependencies
The first step in setting up React Redux involves installing the necessary dependencies. React and Redux are separate libraries, but when combined, they empower developers with an organized way of managing state. To begin, you need to install the Redux library along with , which is the official binding library for React and Redux.
To install these packages, use npm or yarn. Here is the command you can run in your terminal:
This step is essential as it establishes the foundation for your Redux state management system. It’s important to have both libraries synchronized with the latest versions available to avoid compatibility issues.
Creating the Redux Store
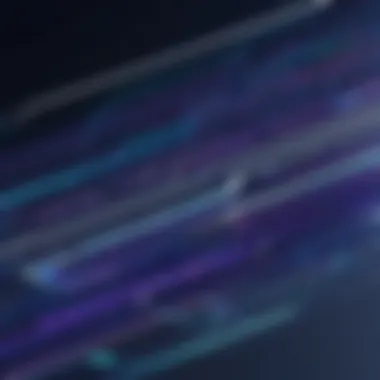
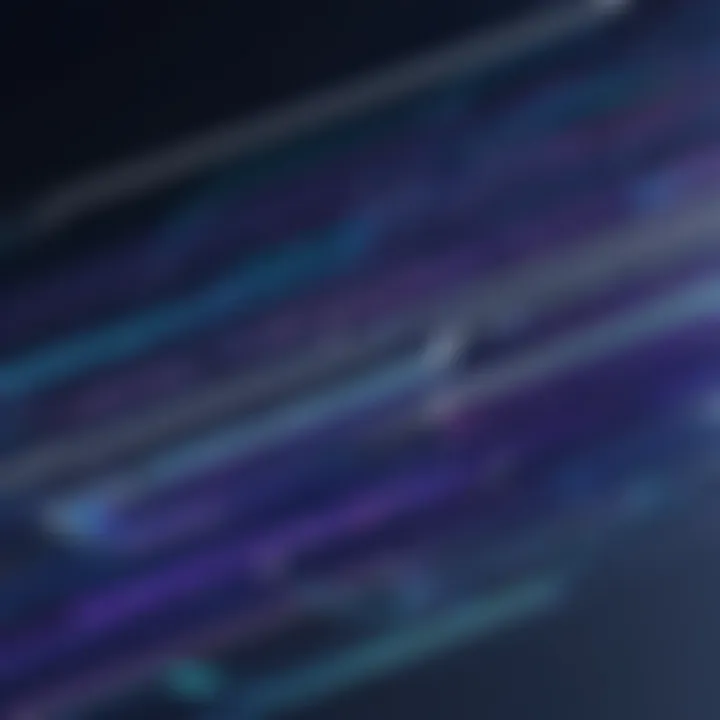
After installing the dependencies, the next step is to create the Redux store. The store holds the entire state of the application and allows access to state via selectors. To create the store, you use the function provided by Redux.
Here is a basic example:
In this snippet, we define an initial state as an empty object and create a which operates based on actions dispatched. This setup is a basic framework that can be expanded with additional reducers and middleware as the application grows.
Connecting Redux to React
The final crucial component of setting up React Redux is connecting Redux to your React components. This process is done using the Provider component from the library, which makes your Redux store available to all nested components. By wrapping your application root component with the Provider, you can seamlessly manage the state throughout the app.
Consider the following example:
In this example, the Provider component wraps the entire App component, allowing access to the Redux store within any nested components. Now, components can subscribe to the Redux store and retrieve the necessary state. With this setup completed, the React application is now ready to utilize Redux effectively.
In-Depth Analysis of the Provider Component
The Provider component is a cornerstone of integrating Redux with React applications. It acts as a bridge that connects React's component tree to the Redux store. This section reveals the deeper functionalities of the Provider, while also addressing essential considerations that developers should keep in mind when implementing it.
Functionality of the Provider
The main purpose of the Provider component is to make the Redux store accessible to all nested components within the React component tree. When you wrap your application with the Provider, you pass the store as a prop. This enables the React components to access the store naturally through the function or the hook. Because of this streamlined access, components can subscribe to the Redux store and automatically re-render when the associated state changes.
The importance of the Provider cannot be overstated. Without it, components would have to prop-drill the store or rely on other methods to access the Redux state, which can lead to complex and inelegant code. By centralizing the state management, the Provider ensures that the application remains organized and maintainable.
Props and the Provider
The Provider requires one key prop: the Redux store. This prop is essential because it informs the Provider how to connect the interface to the underlying state management. This connection is pivotal, as state updates that occur in response to actions get propagated back to the components. In this context, it is also crucial to recognize that the Provider can accept additional optional props, allowing for some level of customization. For example, you can use a custom context if your application demands it, providing flexibility in managing state.
The Provider's props have a direct impact on the efficiency and responsiveness of your application.
When using the Provider, developers should ensure that their store is well-structured and that the necessary middleware is applied appropriately. Poorly structured state or misconfigured middleware can hinder the performance benefits that Redux offers. Therefore, when defining props within the Provider, focus on structuring the store logically and using middleware wisely. This foresight steers developers away from issues that crop up later in the development cycle.
Common Patterns Using Provider
In the context of React and Redux, employing the Provider component is foundational for effective state management. Understanding common patterns of using this component can dramatically enhance application architecture, facilitate debugging, and improve maintainability. Certain patterns have emerged as best practices that align with both the functionality of the Provider and the React ecosystem, and recognizing these patterns is crucial for developers aiming to build robust applications.
Wrapping Your Application
One of the most fundamental uses of the Provider component is wrapping the top-level component of your React application. This is how you integrate the Redux store into your entire component tree. The Provider takes in a prop, which makes the Redux store accessible to any nested components that connect to it.
When you wrap your application with the Provider, the following benefits arise:
- Global Access to State: All components beneath the Provider can access the Redux store. This eliminates the need for passing props through every nested component.
- Simplified State Management: By establishing a clear hierarchy, managing state becomes intuitive. Developers can focus on building components without constantly worrying about their data flow.
- Encapsulation of Logic: Wrapping your app allows you to house your store and related configurations in one place, making your codebase cleaner and easier to maintain.
To implement this pattern, your code could look something like this:
In this example, the entire App component resides within the Provider, allowing it to access the Redux store seamlessly. Such wrapping is a common practice that developers should adopt early in their Redux journey.
Using Multiple Providers
As applications scale, the need for segmenting state management can arise. It is not uncommon to see Redux stores used for disparate components or features within the same application. In situations like this, developers can leverage multiple Provider components to manage distinct stores for various parts of their application.
Using multiple providers can also lead to:
- Decoupled State Management: By segregating state into different stores, you can have dedicated redux logic for different features. This can reduce clutter and improve clarity when debugging.
- Enhanced Performance: With isolated state slices, re-renders can be optimized since components will not subscribe to unrelated data updates. This can ultimately lead to performance enhancements.
- Easier Testing: Testing components can become more straightforward when their dependencies are limited to relevant store aspects.
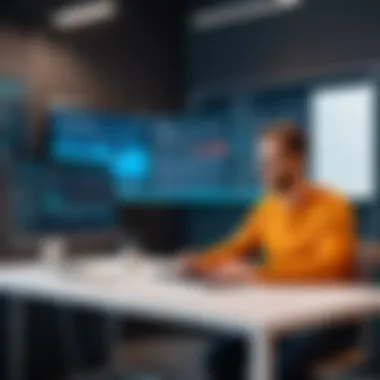
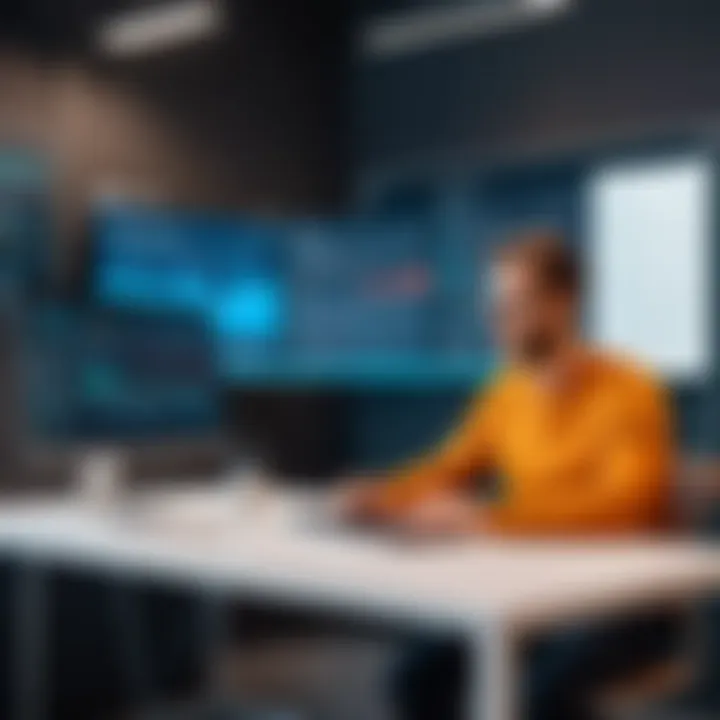
Here’s an example to illustrate wrapping multiple parts of an application with different Providers:
In this example, each Provider corresponds to different features or parts of the application. This pattern allows you to effectively manage state while reducing the complexity that comes with a single monolithic store.
Being mindful of these patterns when utilizing the Provider component will motivate better practices and improved overall architecture for your applications. By thoughtfully structuring how you use the Provider, you can create React applications that not only function well, but are also easier to understand and maintain.
Best Practices for Implementing the Provider
Implementing the Provider in a React application can significantly enhance performance and maintainability when done correctly. Understanding best practices is essential for leveraging the full capabilities of the Redux state management library. These practices not only improve the efficiency of data flow within your application but also simplify the debugging and scaling processes.
Performance Optimization Techniques
Optimization plays a vital role in ensuring applications run smoothly. Here are several techniques to optimize the performance of the Provider:
- Memoization of Components: Utilize React's for functional components. This prevents unnecessary re-renders when props remain the same, thus improving speed.
- Selective Rendering: Instead of connecting every component to the Redux store, focus on connecting only those components that truly need access to the state. This reduces the number of times components re-render.
- Use of Library: By creating memoized selectors, the performance of state updates can improve significantly. This library helps in deriving data from the state while avoiding expensive recalculations.
- Batching Updates: Group multiple actions together. Redux allows for batching of actions, which can reduce the frequency of updates to the components. Ensure to take advantage of this feature where possible.
Understanding how these techniques impact component lifecycle will lead to a smoother user experience and a more reactive application design.
State Structure Considerations
A well-structured state is key to effective state management. Complicated state trees can lead to confusion and performance issues. Hence, it is crucial to create a sensible structure:
- Normalization: Normalize your state shape by keeping related data together. This practice minimizes data redundancy and makes updates more manageable.
- Granular State Slices: Divide state into smaller, manageable chunks. This way, only affected parts of the state will cause a re-render in connected components, optimizing the flow.
- Readability and Maintainability: Ensure your state structure is intuitive. This helps not only in development but also in onboarding new team members and maintaining the code.
A clear structure in state management can greatly enhance both developer experience and application performance.
In summary, applying thoughtful practices when using the Provider will not only elevate the efficiency of your application but also result in easier management of state and performance in the long run.
Troubleshooting Common Issues
In the context of React and Redux, troubleshooting common issues is paramount to maintain the integrity and performance of an application. Being able to quickly identify, analyze, and resolve these issues can save significant development time and improve the end-user experience. This section focuses on two critical areas: debugging state management problems and handling provider errors. Addressing these elements brings clarity to complex interactions, ensuring the seamless functioning of the Redux Provider within your application.
Debugging State Management Problems
State management issues can arise due to various factors, including incorrect state updates, improper action dispatching, or even connectivity problems. Understanding how to debug these problems is essential for any developer using React Redux.
Here are some common strategies for diagnosing state management problems:
- Redux DevTools: Utilize Redux DevTools for a comprehensive overview of your application's state. This tool helps visualize state changes and track actions in real-time.
- Logging: Implement console logging within your action creators and reducers to trace the flow of actions through your state management workflow.
- Component Re-renders: Monitor component re-renders to ensure that they occur as expected. Unwanted re-renders can signify deeper issues within the state or component connections.
- Isolation: Temporarily comment out components or features to isolate the problem area. This method can help identify whether the issue is local to a specific part of your application.
Example of Debugging with Redux DevTools
One effective way to catch issues is by using the time-travel feature in Redux DevTools. By stepping through actions, you can visually inspect how each action modifies your state, allowing for a quick identification of anomalies. Here's a simplified view of how you might set it up:
The key to effective debugging lies in maintaining a clear and consistent approach to your state management workflow.
Handling Provider Errors
Errors related to the Provider component primarily stem from issues with props or incorrect setup. It is crucial to implement error handling to gracefully manage these concerns.
The following guidelines will aid in handling common provider errors:
- Prop Validation: Ensure that all required props are passed to the Provider. Missing or incorrectly typed props can cause unexpected behavior.
- Error Boundaries: Use React's error boundaries in areas where the Provider is utilized. This practice can prevent entire parts of your application from crashing due to provider issues.
- Redux Store Issues: Verify that the Redux store is correctly configured and that middleware, if used, is applied appropriately. Issues in middleware can affect the global state.
- Integration Checks: Regularly check the integration between your React components and Redux. Mismatches in expected state or actions can lead to provider misbehavior.
By taking these steps, you can enhance your ability to address issues that may disrupt the functionality of the Redux Provider, creating a more robust development environment.
Real-World Application of Provider
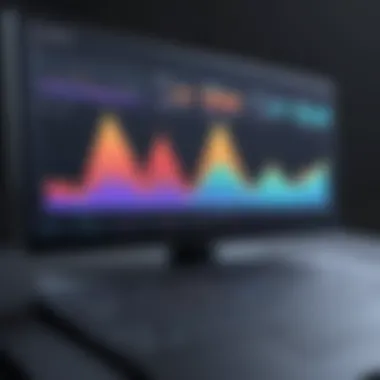
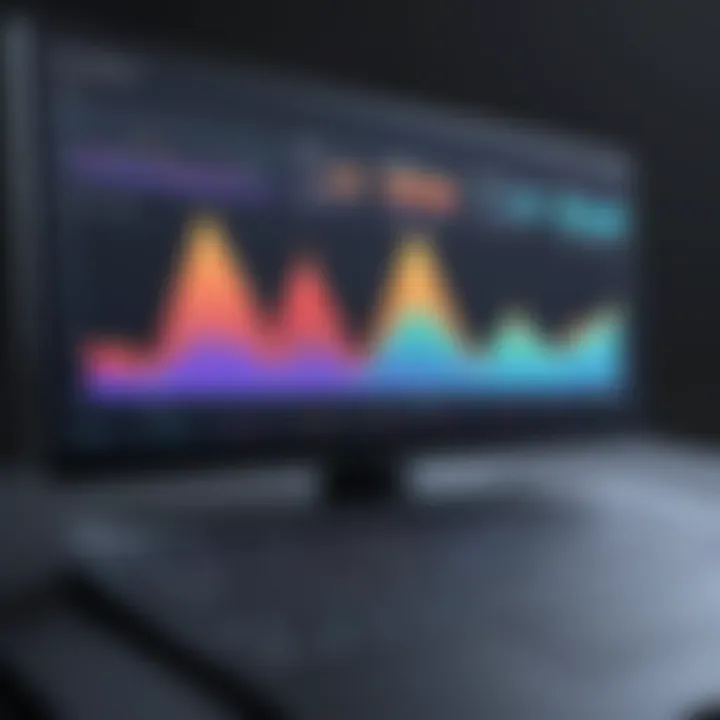
Understanding the real-world application of the React Redux Provider is vital for developers aiming to create efficient and scalable applications. The Provider is instrumental in connecting Redux with React, effectively allowing React components to access the Redux store and its state. By utilizing the Provider, developers can unravel the complexities of state management in React applications, simplifying the process of sharing state across various components. This is particularly significant in larger applications where managing state manually would become cumbersome and error-prone.
The Provider component enables a top-down flow of data which aligns with React’s unidirectional data flow principle. This setup minimizes potential issues that arise from state synchronization in components, as each component can subscribe to the state it needs directly from the Redux store.
Benefits of Using the Provider
- Centralized State Management: It consolidates the application state in one place, making it easier to manage and debug.
- Performance Optimization: By allowing only the components that rely on specific state pieces to re-render, the Provider enhances performance.
- Simplified Code Organization: Abstracting state management through Redux and the Provider reduces boilerplate code within components, leading to cleaner implementations.
Considerations When Implementing Provider
- Nested Providers: While it’s possible and common to have nested Providers for different parts of an application, it adds complexity. Proper planning is essential to avoid redundant states and unexpected behaviors.
- State Shape: The structure of the state in the Redux store plays a significant role in how efficiently the Provider functions. Developers need to think critically about how to organize their state to reflect the needs of their application.
"Using Redux Provider effectively can drastically improve your application's maintainability and scalability."
Key Elements to Focus On
When implementing the Provider in real-world applications, pay attention to:
- Consumer Components: Ensure that only the necessary components are subscribing to the state, which prevents unnecessary renders.
- Middleware: Incorporate middleware, such as redux-thunk or redux-saga, for handling complex asynchronous logic, enhancing the overall state management strategy.
- Testing: Write unit tests to check if the components are interacting with the Redux store as expected. This is crucial for ensuring reliability.
The application of the Provider is not merely a technical implementation; it can bridge the gap between theoretical knowledge and practical execution, allowing developers to build sophisticated applications with ease.
Comparative Analysis
The Comparative Analysis section of this article serves an essential function in clarifying the unique characteristics of the React Redux Provider in relation to other state management solutions. Understanding these comparisons allows developers to make informed decisions about which tool to implement for state management in their applications. When addressing state management, it is crucial to weigh the advantages and drawbacks of various frameworks.
Provider vs. Other State Management Solutions
Redux is often compared with other state management libraries, such as MobX, Context API, and Zustand. Each has unique strengths and use cases. For instance, MobX provides a more reactive programming model, where state changes automatically trigger updates to components. This can simplify code structures in certain applications but may lead to less predictable flows in larger projects.
In contrast, Redux enforces a unidirectional data flow, which can enhance predictability and debuggability. The Redux DevTools are one such advantage, providing robust time-travel debugging capabilities. This makes Redux very appealing to larger applications or enterprises that demand a consistent and manageable state.
When comparing Redux's Provider with Context API, it's essential to note that the latter is suitable for simpler use cases. Context API can handle global state in a more lightweight manner but lacks the performance optimizations found in Redux, especially for deeply nested components. Context might suffer from unnecessary re-renders, which can be managed effectively using Redux.
Ultimately, the choice between Provider and other state management solutions boils down to specific application needs, team experience, and the complexity of the state structure involved. This analysis reveals underlying benefits in structured implementations while identifying potential pitfalls to avoid, thereby guiding developers more effectively in their choices.
When to Use Provider
The decision to leverage the Redux Provider should align with the complexity and requirements of your application. If your application is smaller and involves minimal state interactions, a simpler solution like Context API may suffice. However, as your app grows, incorporating more features often necessitates a robust framework like Redux.
Here are some instances where using the Provider is beneficial:
- Large State Trees: If your application requires managing a large and complex state tree, Redux's Provider excels in maintaining a clear structure.
- Predictability and Debugging: Projects that require systematic state transitions and powerful debugging features benefit from Redux's predictable state management.
- Middleware Support: Redux supports middleware like Redux Thunk and Redux Saga, which can enhance asynchronous logic and side-effect management seamlessly.
- Shared State Across Components: In applications where multiple components need access to the same state, the Provider facilitates this access efficiently.
It is essential to evaluate the unique requirements of your application when deciding to use Provider in Redux.
In summary, the comparative analysis section provides a lens through which we can gain clarity on the Provider's role within the broader ecosystem of state management solutions. Understanding this context, along with identifying when to employ the Provider, empowers developers to craft applications that are not only functional but also resilient and scalable.
End and Future Directions
The conclusion and future directions section serves to encapsulate the core insights gathered throughout this exploration of the React Redux Provider. Understanding the important role that the Provider plays in state management is critical for any developer. It connects Redux's powerful state container to the React component tree efficiently, enhancing app performance and maintainability.
Summarizing Key Learnings
In this article, several key points have emerged:
- Integration Role: The Provider is essential for integrating Redux with React applications, allowing components to access shared state.
- Configuration Practices: Proper setup of the Redux store and the usage of the Provider ensures that state updates are propagated correctly.
- Performance Awareness: Utilizing techniques for performance optimization can significantly reduce re-renders and enhance user experience.
- Troubleshooting: Recognizing and addressing common issues with the Provider can save considerable debug time, simplifying the development process.
"A sound understanding of the Provider boosts development speed and improves application quality."
Potential Developments in State Management
As technology evolves, so do the methodologies for state management. Here are some areas to consider:
- Improved Libraries: New libraries and frameworks are being developed that might offer more efficient patterns than those available today. Therefore, keeping an eye on advancements in state management libraries is advisable.
- React Features: Future React releases may introduce optimizations that affect how the Provider works, enhancing its capabilities.
- Integrating Hooks: With the rise of React hooks, there is potential for new patterns that could simplify how state is managed without relying purely on the Provider.
In summary, the journey into understanding the React Redux Provider illustrates its significance in modern state management. By remaining informed on future developments, developers can continue to leverage this powerful tool effectively.