Setting Up a Comprehensive ReactJS Development Space
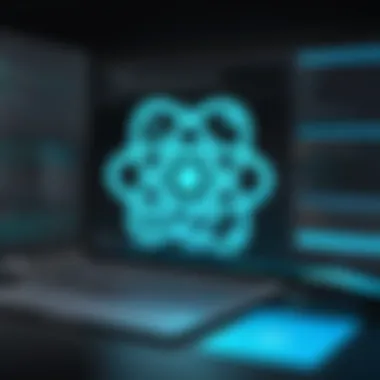
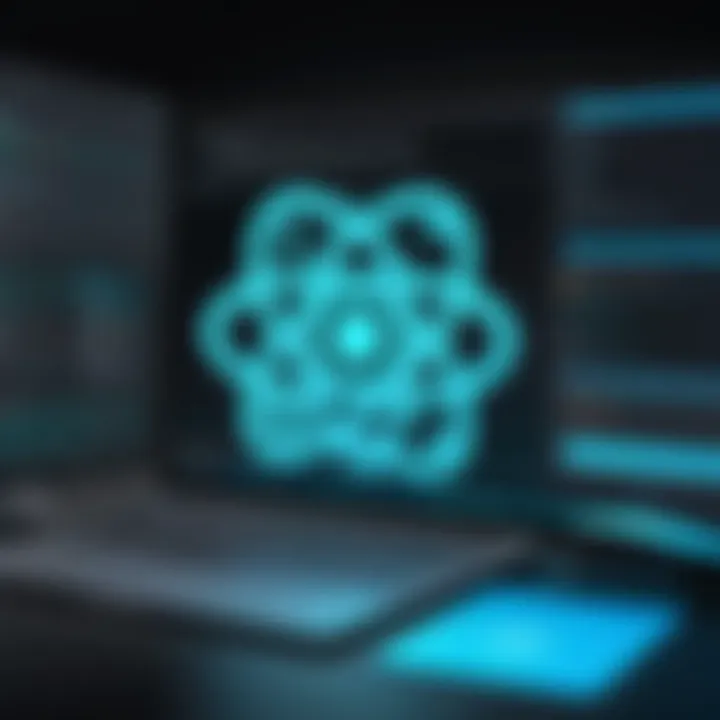
Intro
Creating a strong ReactJS development environment is more than just about coding. It encompasses the entire setup that influences productivity and efficiency. This environment is where developers, both new and seasoned, build applications that can scale and adapt. To set the stage, let’s dive into the current trends within the React ecosystem.
Tech Trend Analysis
Overview of the current trend
ReactJS continues to dominate the front-end landscape, with libraries and tools evolving rapidly. Many developers now adopt a modular approach, integrating diverse solutions such as TypeScript, Redux, and even testing libraries right alongside React. This trend reflects a broader industry movement toward increasing maintainability and enhancing collaboration among development teams. The emphasis has shifted from simply using React to optimizing the development workflow with supportive tools.
Implications for consumers
For the end-users, these advancements mean more robust and user-friendly applications. As developers hone their skills and tools, the applications become faster, have fewer bugs, and ultimately provide a better overall experience. The smoother user interfaces and richer functionalities lead to higher satisfaction rates and increased engagement.
Future predictions and possibilities
Looking down the road, we can expect further integration of artificial intelligence and machine learning within React applications. This will facilitate smarter applications capable of adapting to user behavior. Additionally, as the push for server-side rendering grows, tools like Next.js are likely to gain even more traction, setting the stage for faster load times and improved SEO capabilities.
How-To Guides
Prologue to the topic
Setting up a ReactJS development environment requires a thoughtful approach. It might seem overwhelming at first, but breaking it down into manageable steps makes the process smoother.
Step-by-step instructions
- Choose Your Code Editor: Popular choices include Visual Studio Code and IntelliJ IDEA, thanks to their rich plugins for React.
- Install Node.js: This is crucial; React runs on Node.js. Following that, check for npm (Node Package Manager):
- Create Your React App: Utilizing the Create React App setup can save time. Just use:
- Integrate Version Control: Set up Git for version tracking. This step is vital for collaborative tasks.
- Configure ESLint: Linting helps maintain coding style and prevents errors.
Tips and tricks
- Familiarize yourself with the React documentation; it’s rich in examples and best practices.
- Use debugging tools like React DevTools to streamline the development process.
Troubleshooting
When things don’t go as planned, common issues arise such as:
- Build Failures: Check your Node and npm versions.
- Dependency Conflicts: Use the command to track down conflicts.
Setting up a ReactJS environment isn't just technical; it's about creating the best context for building great applications.
Industry Updates
Recent developments in the tech industry
With significant updates in the React ecosystem, it's vital to stay updated. Tools and libraries surrounding React, like Remix and Recoil, are receiving a lot of attention for their innovative approaches to state management and routing.
Analysis of market trends
The rise of Jamstack architecture is reshaping how React applications are built. It emphasizes pre-rendering and decoupling the frontend from the backend, allowing for better performance and security.
Impact on businesses and consumers
For businesses, these trends translate to quicker time-to-market and lower overall costs. Meanwhile, consumers benefit from more secure and responsive applications, dictating a more engaging digital experience.
By understanding these facets, developers can build environments that are not only productive but also forward-thinking.
Understanding ReactJS
Understanding ReactJS is essential when setting up a robust development environment. It's not just about knowing how to write the code; it's about grasping the framework's fundamental principles, which aids smoother workflows and more effective problem-solving. When developers understand what React is and how it operates, they are better equipped to optimize applications, enhance performance, and maintain code more effectively.
What is ReactJS?
ReactJS is a JavaScript library primarily used for building user interfaces, especially for single-page applications. It lets developers create large web applications that can change data without reloading the page. This capability gives end-users a seamless experience, akin to that of a desktop application. The library was developed by Facebook and has become widely adopted due to its component-based structure that promotes reuse and maintainability.
Using ReactJS means structuring your application around components, which can be thought of as custom elements that encapsulate both logic and presentation. This allows for a more declarative style of programming where the UI is a reflection of the data, making it easier to track changes and debug.
Key Features of ReactJS
A few key features make ReactJS particularly appealing:
- Virtual DOM: React does not update the actual DOM but instead maintains a virtual representation of it. This process reduces the performance cost of DOM manipulation.
- Component-Based Architecture: Each component manages its state and can be reused across various parts of an application, promoting consistency and reducing redundancy.
- JSX Syntax: React uses JSX, which allows developers to write HTML elements in JavaScript. This syntax is highly expressive and simplifies the process of crafting dynamic and interactive UIs.
- Unidirectional Data Flow: Data flows in one direction, making it easier to track changes. You know exactly how the data should flow, reducing complexity in large applications.
- Rich Ecosystem: Coupled with various tools and libraries, React provides a rich ecosystem that allows developers to scale projects efficiently.
The Evolution of ReactJS
ReactJS has seen significant evolution since its initial release in 2013. Initially, it didn’t support many features we see today. The introduction of React Hooks in 2018 was a turning point. It allowed developers to use state and other React features without writing a class, simplifying the codebase considerably.
Additionally, the community surrounding React has grown immensely. Various contributors constantly develop new enhancements, libraries, and tools, leading to a robust ecosystem. The continued focus on performance optimization and developer efficiency demonstrates why React remains a popular choice in both small and large-scale projects.
"A solid understanding of ReactJS is not just beneficial; it's pivotal in establishing strong foundations for all your development efforts."
As you dive deeper into ReactJS, comprehending these facets will enhance not only your interaction with the library but also your overall development experience. Recognizing why and how React functions sets the stage for establishing a solid development environment tailored to your specific requirements.
Prerequisites for Setting Up ReactJS
To effectively dive into the world of ReactJS, it's crucial to have a solid grasp of certain prerequisites. Establishing a robust development environment isn’t just about having the right tools; it’s also about ensuring you possess the foundational knowledge and software required to unleash ReactJS's full potential. This section will highlight essential elements such as programming knowledge and the necessary software. By grasping these prerequisites, users can streamline their learning process and avoid common pitfalls.
Basic Programming Knowledge
Before you can roll up your sleeves and start coding with React, having a firm foundation in basic programming concepts is vital. Understanding principles such as variables, loops, functions, and control structures will provide you with the necessary toolkit to tackle more complex topics down the line.
In particular, familiarity with JavaScript is non-negotiable. React is primarily built upon JavaScript, so being comfortable with its syntax will enable you to read and write React code easily. This is often where many newcomers stumble; they dive head-first into React, only to be bogged down by JavaScript's nuances.
It's not just the syntax of JavaScript you need to be cautious of, though. The asynchronous nature of JavaScript, such as promises and callbacks, plays a significant role in how React works. One must grasp how to manage asynchronous behavior, especially when working with APIs or fetching data. Coding is a craft, and having this skill set helps you create cleaner and more efficient code as you progress.
Required Software
Node.js
One of the cornerstones of any ReactJS development environment is Node.js. This open-source, cross-platform JavaScript runtime allows developers to execute code server-side. Node.js is built on Chrome's V8 JavaScript engine, offering performance and efficiency. This environment is key because it enables the use of JavaScript on the backend, facilitating a more holistic use of the language throughout your application.
The key characteristic of Node.js is its event-driven, non-blocking I/O model, making it particularly suitable for building scalable network applications. In the context of React, integrating Node.js into your setup means you can effortlessly manage features like real-time updates, enhancing user experience.
However, while Node.js is a beneficial choice, it comes with its quirks. For instance, some developers may find its asynchronous patterns challenging to work with initially. Yet, once you wrap your head around it, the advantages far outweigh any drawbacks.
npm
Another integral software component is npm, the default package manager for Node.js. When you set up React projects, npm allows you to easily download and manage libraries, frameworks, and tools that are essential for development.
What makes npm stand out is its large registry of packages. You can think of it as a treasure trove for developers; most of the tools and libraries you might want to utilize are just a command away. By using npm, you avoid the hassle of manually downloading libraries, which can lead to compatibility issues.
Nevertheless, npm is not without its hiccups. Versioning issues sometimes arise when packages are updated, but knowing how to manage those risks can save you a lot of headaches in the long run.
Code Editor
Finally, a good code editor is indispensable in your ReactJS setup. While there are several options available, selecting the right editor can make a world of difference in your productivity and coding experience. The most popular choices include Visual Studio Code, Sublime Text, and Atom.
A significant aspect of any code editor is its customization and extensibility. For instance, Visual Studio Code allows you to install various extensions that enhance syntax highlighting, linting, and debugging, which are critical for React development. This tailored experience leads to increased coding efficiency and helps you catch potential errors early in the process.
As developers will know, not all editors are created equal. Some might provide a more pleasant experience for specific tasks, while others might fall flat. As such, experimenting with a few different options will help you find what works best for your style.
Finding the right tools and knowledge can often be the first stepping stone toward building efficient and maintainable ReactJS applications.
By laying this groundwork, you’re setting yourself up for success in React development. Understanding the prerequisites will not only save you time but also enable you to dive deeper into the functionalities and intricacies of ReactJS itself.
Selecting the Right Code Editor
Choosing the right code editor is a critical decision that can shape your ReactJS development experience. An effective code editor not only enhances productivity but also streamlines the process of writing, testing, and debugging your applications. It serves as the primary workspace where you tackle the intricacies of coding. Factors like performance, user interface, and availability of plugins or extensions play a pivotal role in making the right choice.
Using a suitable code editor can lead to improved code quality, reduced errors, and a more enjoyable coding experience. Equipping yourself with the right tools can save you countless hours in the long run. The competition is stiff, with several editors offering unique features and capabilities that cater to different preferences.
Popular Code Editors for ReactJS
When discussing coding tools for ReactJS, a few options stand out in the crowd. Each editor brings its own flavor to the table, adapted for both novices and seasoned developers alike.
Visual Studio Code
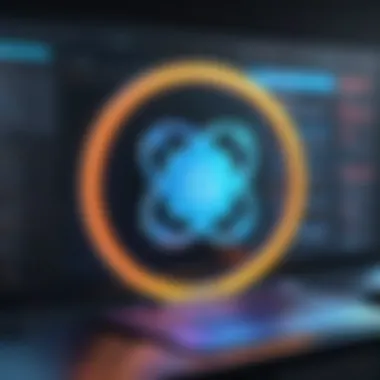
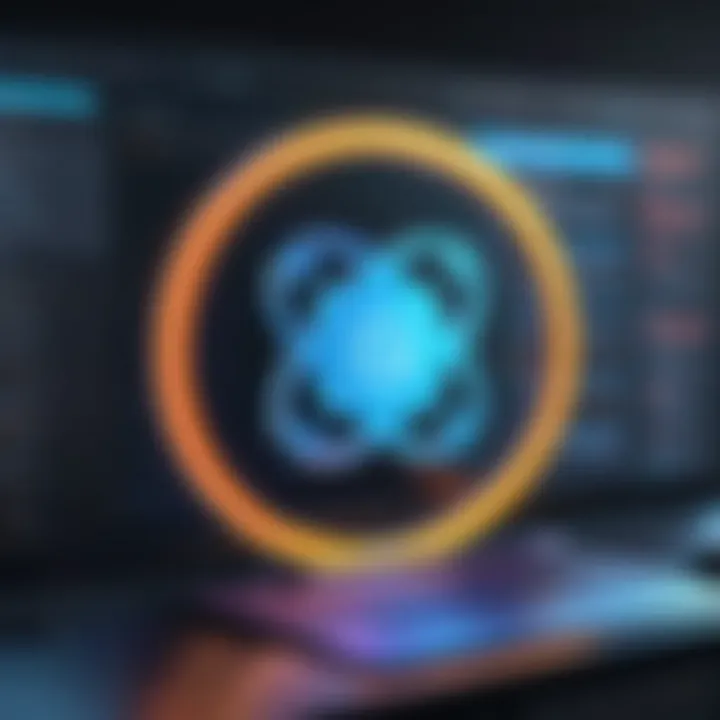
Visual Studio Code is arguably a frontrunner among code editors for ReactJS development. One of its most notable aspects is its lightweight nature combined with powerful features. The flexibility to customize the interface and add functionalities through extensions makes it highly appealing. Among its key characteristics, the integrated terminal and Git integration provide a seamless workflow.
A unique feature of Visual Studio Code is IntelliSense, which offers code completion suggestions that can significantly boost productivity. However, it can be resource-intensive on lower-end machines, which is something to keep in mind if your hardware is on the older side. Overall, its rich ecosystem of extensions and active community help make it a favored choice for many developers.
Sublime Text
Sublime Text presents itself as a sleek and fast text editor. Its key characteristic is the command palette, which takes code navigation to an entirely new level. It allows developers to execute commands quickly without delving through numerous menus. This streamlined interface saves time, especially when working on sizeable projects.
One unique aspect of Sublime is its "Goto Anything" feature, which enables you to quickly jump to a specific file, line, or symbol. While Sublime Text excels in speed and ease of use, it operates on a license model that some may find limiting. In the end, if speed is your top priority, Sublime might just fit the bill.
Atom
Atom, developed by GitHub, has made waves among developers as a customizable open-source editor. It offers a friendly user interface along with a robust system for package management. What sets Atom apart is its easy customization through themes and packages, allowing developers to configure their environment to their liking.
The collaborative editing feature is a unique aspect of Atom, enabling multiple developers to work on the same file simultaneously. It is worth noting, however, that Atom can become sluggish with larger projects, which could hinder productivity over time. Nevertheless, it's a dependable choice, especially if working closely with GitHub and requiring collaboration tools.
Extensions and Plugins to Enhance Productivity
Beyond the choice of editor itself, extensions and plugins can significantly elevate your workflow. Utilizing tools such as Prettier for code formatting and ESLint for linting code can streamline your development process. These tools ease the burden of manual checks and formatting, letting you concentrate more on writing quality code rather than focusing on syntactical details.
Installing Node.js and npm
Setting up a ReactJS development environment hinges significantly on the installation of Node.js and npm. These two tools form the backbone of any JavaScript project, enabling developers to run JavaScript on the server side and manage project dependencies efficiently. Understanding their importance is fundamental as they contribute to a smoother development experience, optimizing performance and workflows.
Node.js is a runtime built on Chrome's V8 JavaScript engine, allowing developers to execute JavaScript code outside the confines of a web browser. This capability lays the groundwork for various server-side applications. npm, which stands for Node Package Manager, accompanies Node.js and provides the necessary tools for managing packages or libraries that developers might need during application development. Essentially, npm gives developers access to a vast ecosystem of pre-built and community-contributed code.
In short, without Node.js and npm, the ReactJS development workflow would be impractical; it's like trying to bake a cake without an oven. Setting these two tools up correctly is vital for any developer aiming to build scalable and efficient web applications.
Installation Process
Installing Node.js and npm is a straightforward process that involves downloading the installer from the official Node.js website. Here’s a brief overview of the steps:
- Visit the Node.js website: Go to the Node.js official site. You’ll notice two primary download options: LTS (Long Term Support) and the Current version. For most applications, downloading the LTS version is advisable as it tends to be more stable.
- Download the installer: Depending on your operating system (Windows, macOS, Linux), select the appropriate version and download the installer file.
- Run the installer: Open the downloaded file and follow the prompts. Ensure to accept the license agreement and select the options for typical installations. It’s often worth checking off the box to install the necessary tools for native modules. This will save headaches down the line.
- Complete the installation: After the installation concludes, close the installer.
- Add to PATH environment variable (if necessary): Typically, the installer adjusts the PATH variable for you, but if you run into issues, you might need to add Node.js to your environment variables manually.
Verifying Installation
Once you've installed Node.js and npm, the next logical step is verification. It’s always best to confirm that everything is working as intended. To do this, you can follow these steps:
- Open your command line interface (CLI): Use Terminal on macOS or Command Prompt/PowerShell on Windows.
- Check Node.js version: Input the following command and hit Enter:This will return the version number of Node.js currently installed. If you encounter an error message, it indicates that Node.js has not been installed correctly.
- Check npm version: Similarly, you can verify npm by typing:Again, this should return a version number. If it doesn’t, there may have been an issue during installation.
- Double-check installation: For added assurance, you can create a simple JavaScript file to test if Node.js is functioning correctly.Run this file from your CLI:
"Verifying installations can save a lot of time debugging later on; ensuring your foundations are solid gives you peace of mind."
Getting comfortable with these initial steps is crucial. The reliability of your entire ReactJS project will be tied to the successful installation and functionality of Node.js and npm.
Creating a New React Application
Creating a new React application is the cornerstone of any successful project using this popular JavaScript library. In this phase, you set the stage for your entire development journey. This is not merely about establishing the skeleton of your app but rather about understanding the various methodologies and tools available to kick start a robust development process. The significance of this topic lies in its ability to streamline your workflow and provide a solid foundation that encourages productivity.
The initial steps in setting up your app will determine how smoothly your project progresses. Establishing a new React application sets the groundwork for efficient code management, scalability, and future feature integrations—elements that are crucial as your application evolves. Utilizing the right tools and processes can significantly reduce troubleshooting times and confusion in the later stages of development.
Using Create React App
When it comes to initiating a new React application, Create React App is often the go-to solution for many developers. This tool abstracts away the complexities involved in configuring a React application, allowing developers to hit the ground running. Without needing to fiddle with intricate build configurations, you can focus on building your application rather than wasting time on setup.
To create an application using Create React App, open your terminal and simply run the following command:
Replace with the name you want for your project. This commands helps you generate a fully functional React application with an organized folder structure and a standard configuration already in place. The tool installs Webpack, Babel, and other essential libraries under the hood, so you don't have to deal with the nitty-gritty details.
Just like that, your app is up and running! This means that you can now direct your energy toward writing code, crafting components, and building a user interface that catches users’ eyes.
Understanding Project Structure
Once your application is set up, understanding its project structure is pivotal. The conventional layout consists of folders and files that separate various functionalities and assets, making the application scalable and maintainable. Typically, you'll find the following structure in your newly created React app:
- node_modules/: This folder holds all your project’s dependencies.
- public/: Here, you can place static files such as HTML, icons, and images. Anything in this folder will be served directly to the web.
- src/: This is the heart of your application.
- index.js: The entry point for your React app, where the rendering begins.
- App.js: This is where your main application logic resides. You’ll be building your components and functionalities around it.
- components/: A common practice is to create a separate folder for reusable components.
Understanding this structure helps in organizing your code effectively. You’ll want to create components that are coherent, reusable, and easily maintainable. If you find yourself lost, it’s useful to refer back to this structure as a guide, ensuring that your files and components align with best practices.
"A well-structured project today can save you headaches down the line."
Finally, knowing where to find and place files encourages better team collaboration. Team members can quickly locate specific parts of the codebase without fumbling around, which serves to keep everyone on the same page.
In summary, creating a new React application is an essential step that requires thoughtful consideration and organization. By leveraging Create React App and understanding the project structure, you're well on your way to developing an efficient, scalable application that can adapt and grow with your project needs.
Folder Structure in React
Establishing a robust folder structure in React is a significant step in ensuring that your development process flows smoothly and stays organized. A well-thought-out structure can save you from headaches down the line. Properly organizing your files makes it easier to locate components, assets, and styles when the project begins to grow. Setting the right foundation at the start can guide you through the inevitable complexities that arise in larger applications. It’s not just about tidiness; it’s about enabling efficiency and scalability.
Organizing Components
When it comes to organizing components, there’s a fine balance between keeping things modular and ensuring they aren’t scattered all over the place. Ideally, creating a dedicated folder is advisable. This folder can house all presentational components, containers, and any reusable parts of your project. It would be beneficial to create subfolders inside the components folder for different features or functionalities.
For example, let’s say your application has a dashboard. You might have:
- components/
- Dashboard/
- DashboardHeader.js
- DashboardSummary.js
- DashboardChart.js
- DashboardFooter.js
Each component can focus on a single job, making them easy to understand and maintain. Following this pattern results in self-contained units that can be tested independently, avoiding any potential confusion when several components are jostling for space in the same file.
Additionally, adhering to a naming convention helps maintain clarity. Use clear, descriptive names that reflect the functionality of the component. So instead of , opt for or , which clearly indicates the purpose.
"A clear structure in your code base is like a well-organized toolbox. You know exactly where to find your tools when you need them."
Managing Assets and Styles
Managing assets and styles properly is equally crucial for a React project. Much like components, keeping your assets together helps streamline the workflow. Create an folder in the root of your project. This folder can be divided into subfolders for images, fonts, and any other static files you might need.
For example:
- assets/
- images/
- fonts/
When it comes to styles, a common practice is to have a folder or to group styles alongside components in their respective folders. If you maintain global styles, it’s wise to have a separate or file in the styles folder. Here's how the structure may look:
- styles/
- GlobalStyles.css
- components/
- Button.css
- Card.css
This segregation makes it easier to maintain and update both assets and styles. It avoids confusion, especially as your application scales. The clarity you achieve here will pay off when you, or someone else, faces the task of modifying or debugging the application later on.
Ultimately, a well-organized folder structure is one of those behind-the-scenes aspects that often goes unrecognized. Yet, it’s the backbone that supports efficient productivity in a React application, giving developers the agility they need to tackle new challenges and deliver seamless user experiences.
Using Development Tools
In the landscape of ReactJS development, the tools you utilize can make or break your productivity. Using the right development tools not only streamlines your workflow but also enhances code quality and facilitates collaboration within a team. Without these essential tools, developers may find themselves trapped in a cycle of inefficiency, spending more time troubleshooting than coding.
React Developer Tools
React Developer Tools is a powerful browser extension that brings significant advantages for both debugging and performance monitoring. This tool provides insights into the component hierarchy of your React application, enabling you to track the current state of components and observe their props.
One key benefit of the React Developer Tools is the ability to inspect the performance of your components. With profiler capabilities, you can identify components that may be taking too long to render, allowing you to make improvements where necessary. Such insights are crucial when scaling applications since some components may lag as your app grows.
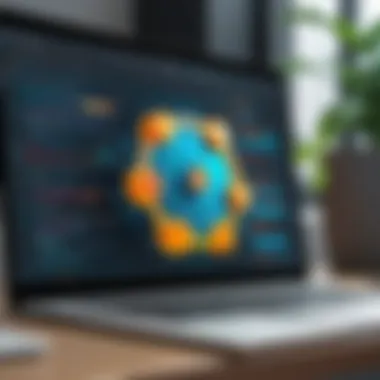
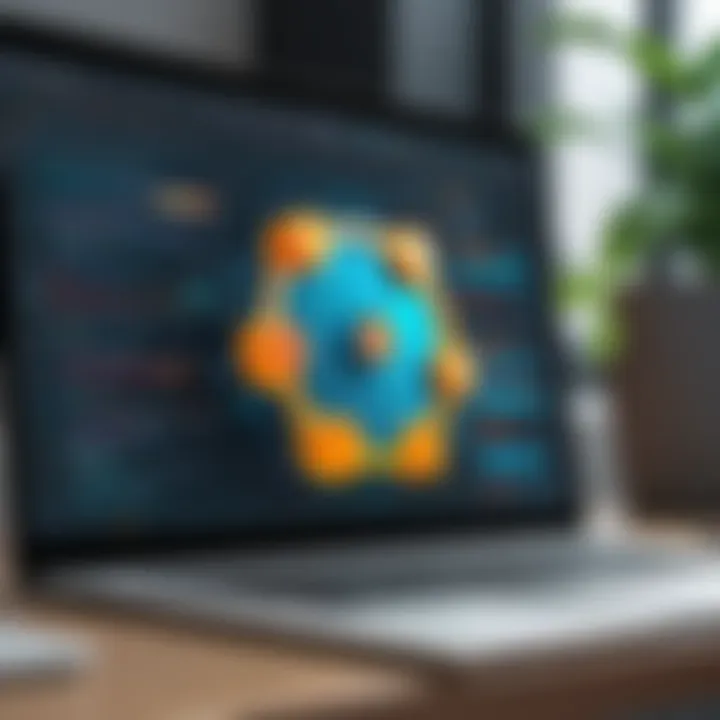
To make the most of React Developer Tools:
- Install the Extension: It’s available for both Chrome and Firefox. Just search for "React Developer Tools" in the extensions store and hit install.
- Familiarize Yourself with the Components Tab: This section shows your React component tree, making it easier to understand how props and state flow throughout your app.
- Utilize the Profiler Tab: After you enable the profiler, it records how long each component takes to render during interaction.
"Having the right tools is like having the right map; they will guide you smoothly through the wilderness of development!"
Linting and Formatting Tools
Linting and formatting tools play a pivotal role in maintaining code quality. Linting checks your code for potential errors and enforces style guidelines, while formatting tools ensure that your code remains neat and readable.
Consider employing ESLint, a popular linting tool for JavaScript. It helps you catch syntax errors and enforces coding standards to ensure your code is consistent. This prevents common issues from slipping through the cracks, especially in a team environment where coding styles may differ significantly.
In addition, tools like Prettier come into play for automating code formatting. By selecting a single formatting style, you ensure that all developers on the team follow the same conventions. This not only improves readability but also reduces the time spent on code reviews.
To effectively utilize linting and formatting tools:
- Set Up ESLint in Your Project: You can do this by initializing ESLint in your project using and following the prompts.
- Install Prettier: Integrate Prettier into your project with . Configure it with a file to define your preferred formatting rules.
- Combine ESLint and Prettier: This ensures that code is linted and formatted according to both tools. Tools like can help eliminate conflicts between the two.
Employing these development tools greatly enhances the coding experience and fosters a culture of quality and efficiency in ReactJS development.
Handling Dependencies
Managing dependencies is a crucial part of developing a ReactJS application. It ensures that all the tools and libraries your project needs are available and functioning correctly. Handling dependencies well not only streamlines development but also prevents potential conflicts that may arise later in the project. Without a clear strategy for managing these dependencies, you might find yourself knee-deep in issues that could slow down your progress or even halt it altogether.
There are specific benefits to mastering dependency management:
- Version Control: Every library can have different versions, and updating one could create compatibility issues. Understanding how to handle this—especially with tools like npm—keeps your project stable.
- Easy Rollbacks: When an update breaks something, having a well-defined process allows reverting to stable versions reliably.
- Cleaner Project Structure: Properly managing dependencies leads to a more organized project, making it easier for collaborators and future you to understand.
It's essential to consider how these dependencies interact with one another. Each package you add can have its own dependencies, leading to potential conflicts. Therefore, a clear understanding of how to read and modify the file in your project will serve you well.
Understanding Package.json
The file is like the DNA of your project. It contains all the vital information about your project and its dependencies. This includes the name, version, scripts to run, and, most importantly, the dependencies your project requires.
Imagine trying to build a car without knowing which parts you need—it would be a disaster. Similarly, without a clear and updated , your project can quickly spiral into chaos. Here’s what you typically find in this file:
- Dependencies: Lists libraries that your project needs during runtime.
- DevDependencies: These are necessary only during development, like testing utilities.
- Scripts: This section allows you to define commands like , , or custom scripts that can run during various stages of development.
When you want to add or update a package, this file makes it all seamless. You simply use npm commands, and the changes are reflected instantly. For most developers, a solid understanding of translates to significant efficiency down the line.
"Every dependency is a double-edged sword; know how to wield them before they cut you."
Using npm to Manage Packages
Using npm (Node Package Manager) is fundamental for managing packages in a ReactJS environment. It simplifies the process of adding, updating, or removing libraries and tools in your project. You can interact with npm using the command line, and here are some common commands that can be instrumental for any developer:
- Installing New Packages:
To install a package, you simply run . This command not only adds the package to your project but also updates the and files to reflect this change.
Example: - Updating Packages:
Keeping your dependencies up to date is critical. You can run to update all packages, or to target a specific one. - Removing Packages:
If a library no longer meets your needs, you can easily remove it with , which will tidy things up—removing the library and updating the as required. - Listing Installed Packages:
Running will show you all the packages currently installed, along with their versions. This is handy for auditing your dependencies and ensuring everything is on the up-and-up. - Checking for Vulnerabilities:
You can use to identify security vulnerabilities among your dependencies, ensuring that you keep your project secure.
In summary, handling dependencies effectively via and npm is not just a nice-to-have; it's an essential skill for ReactJS developers aiming for robust and maintainable applications.
Configuring Environment Variables
A well-structured ReactJS development environment goes beyond just getting the code to run. Configuring environment variables plays a pivotal role in ensuring that your application operates smoothly and securely across various stages of development, testing, and production. Environment variables allow developers to define settings and configurations that can change depending on the environment where the application is running. This is particularly important not only for maintaining security by avoiding hard-coded sensitive data, but also for enhancing flexibility and scalability.
In React applications, environment variables streamline the configuration process by allowing you to differentiate between local development and deployment environments. This is crucial for settings such as API keys, database URLs, or even feature flags that should not be exposed in public repositories. By leveraging these variables, you can make your application more robust and easier to manage.
Creating .env Files
Creating .env files is a straight-forward but vital step in configuring these environment variables. A .env file is essentially a text file that stores key-value pairs of variables.
Here’s a simple starting point:
When you define variables in this format, they become accessible through the object in your React application. When you start your React application using a command like , the React scripts will load the variables defined in the .env file automatically.
It's worth noting that the variable names must start with to be recognized by Create React App, so remember that little detail!
Best Practices for Environment Variables
When working with environment variables in your React application, adhering to best practices ensures that your app remains secure and maintainable. Here are key strategies to consider:
- Keep Sensitive Data Confidential: Never hard-code sensitive information such as API keys directly in your codebase. Use environment variables instead to keep such information out of your source code.
- Separate Environment Files: Consider maintaining separate .env files for different environments, like and . This allows clarity in what each environment should be using and minimizes the risk of deploying development configurations to production.
- Use Defaults with Caution: If you have default values, ensure they are safe for use in production and do not expose sensitive information.
- Document Your Variables: Maintaining a documentation file that explains the purpose of each environment variable can be very helpful for team members or for future reference.
- Avoid Committing .env Files: Ensure that your .env file is included in the file to prevent it from being pushed to a public repository. This is crucial for protecting sensitive information.
"By following these best practices, developers can create secure and maintainable React applications that protect sensitive data while ensuring a smooth deployment process."
These practices will not only help you maintain a secure development environment but also promote a cleaner workflow overall. Remember, a strong foundation leads to greater productivity and fewer headaches down the road.
Setting Up Version Control
In the fast-paced, ever-changing realm of software development, one cannot underestimate the importance of establishing a robust version control system. Setting up version control is like laying a solid foundation before building a house. Without it, you risk chaos lurking just around the corner. It allows developers to track changes, collaborate efficiently, and manage the project over time.
Version control not only preserves your work but also provides a way to revert back to previous states if things go awry. This capability is invaluable, particularly when multiple developers are working on the same codebase. It prevents conflicts and makes merging changes smoother than a hot knife through butter.
Using Git for Version Control
Git is the crown jewel of version control systems.
- Local and Remote Repositories: With Git, developers can have a local copy of their entire project history. This way, you can work offline and push changes when it suits you. The remote repository acts as a shared hub, facilitating collaboration.
- Branching and Merging: One remarkable feature of Git is its branching capability. Developers can create separate branches for new features or bug fixes, allowing for experimentation without disturbing the main codebase. Once the feature is polished and gleaming, it can be merged back into the main branch.
- Tracking Changes: Every change is recorded, and Git keeps an exhaustive history. It helps one to understand who did what and when—a crucial aspect for teams as it fosters accountability and transparency.
Getting your hands dirty with Git is a journey worth taking. Here’s an example of how to initialize a Git repository:
Integrating with GitHub
Once you've tamed Git, it's time to make it social. Integrating your project with GitHub elevates your development game to a new level.
- Collaborative Environment: GitHub provides an easy platform for collaboration. Team members can contribute from various corners of the world without stepping on each other's toes. Issues can be reported and tracked, and discussions tied directly to code changes lead to clarity.
- Pull Requests: When you’re ready to merge changes from a feature branch, GitHub's pull request feature allows for code reviews, discussion, and additional checking before integration into the main project. Think of it as quality control for your code.
- Deployment: Many deployment pipelines integrate seamlessly with GitHub, enabling effortless deployment of the latest stable code. It makes the whole process feel as smooth as silk.
Utilizing Git and GitHub not only enhances your productivity but also helps in maintaining a clean project structure. It’s a necessary skill that every developer should have under their belt.
Rather than being just a safety net, version control is your partner in crime, helping you track progress and maintain quality without the headache of losing your hard work.
Testing and Debugging React Applications
In software development, particularly in environments as dynamic as ReactJS, testing and debugging are not just additional steps but are indeed critical for ensuring the reliability and quality of applications. By incorporating comprehensive testing strategies and efficient debugging techniques, developers can preemptively catch errors and guarantees that applications perform as intended across different scenarios. It’s often said that "an ounce of prevention is worth a pound of cure," and this rings especially true when it comes to managing the complexities of a ReactJS application.
Engaging in unit testing allows developers to validate the functionality of small parts of the code independently. This modularity is essential in ReactJS since components act as the building blocks of any application. If a single component fails, testing can quickly identify the source of the problem—saving precious development time.
Meanwhile, debugging is like having a crisp map in a territory full of twists and turns. It’s not just about finding problems, but also understanding why they occurred in the first place and preventing future occurrences. The art of debugging is one that all developers on their journey should master, especially those navigating the vast landscape of React.
Unit Testing with Jest
Jest has emerged as a staple for unit testing in React applications. It's a delightful testing framework that is not only easy to set up but also shines with its rich feature set. The beauty of Jest lies in its ability to provide a solid platform for testing any JavaScript code, including React components, APIs, and utility functions.
Key Features of Jest
- Snapshot Testing: This allows developers to take a snapshot of a component's rendered output, making it simple to compare against future outputs.
- Mocking Functions: Jest provides natural mocking capabilities that help isolate units for tests, allowing you to test components without dependencies.
- Rich API: The API is quite user-friendly, making it easy to set up tests without getting buried in complex syntax.
Here's a small example of how a simple test could be set up using Jest:
This sample not only demonstrates how to render a component but also checks if certain text is present. Such straightforward tests can significantly improve the robustness of your application, catching errors early in the development phase.
Debugging Techniques
Next up is debugging. While Jest takes care of unit testing, debugging techniques can help you make sense of your code during runtime.
Here are some prevalent strategies to enhance your debugging experience:
- React Developer Tools: This browser extension is a game changer, allowing you to inspect React component hierarchies and observe their current props and states.
- Console Logging: Sometimes, the simplest method is sufficient. By sprinkling statements judiciously throughout your code, you can track variable values and discern where your logic may have veered off course.
- Source maps: When debugging with tools like Chrome DevTools, source maps help translate back to your original code instead of minified versions, making it easier to identify issues.
Additionally, embracing some common practices can elevate the efficiency of your debugging process:
- Always keep your components small and focused. This minimizes complexity.
- Use tools such as Redux DevTools if your app relies on Redux for state management—it provides a fine-grained view into state changes.
- Maintain a consistent logging approach to track issues as they arise, allowing for faster isolation of problematic areas.
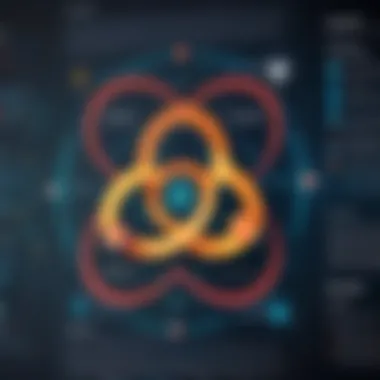
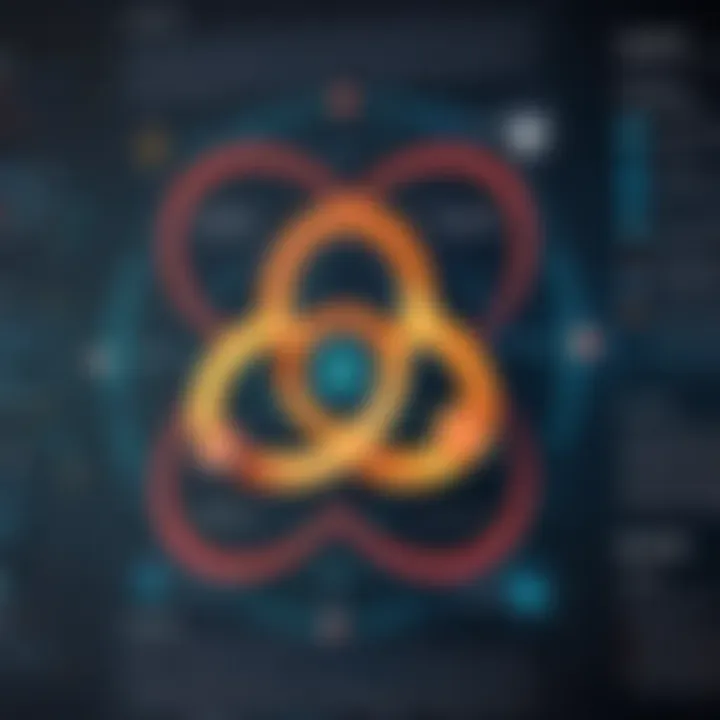
In sum, both testing and debugging are integral parts of the development lifecycle in the React ecosystem. By prioritizing them, developers can produce cleaner, more reliable applications, significantly streamlining the process of building robust user interfaces.
Building and Deploying React Applications
The process of building and deploying React applications is a critical undertaking for any developer. This ensures that the end product is not only functional but also efficient and scalable. Building is about transforming source code into production-ready assets, while deployment is about making these assets available for users. In today’s fast-paced world, quick deployment and efficient performance are essential for user satisfaction. Understanding these two processes allows developers to set the bar high for quality and user experience.
Creating Production Builds
Creating a production build is the stage where your React application transitions from development to a fully optimized version ready for users. This step is vital because it involves various techniques to minimize your code. This can lead to faster load times and improved performance.
To create a production build, you generally use a command like or . Here’s a key point: when you execute this command, React relies on Webpack to compile and bundle your application efficiently.
The production build compresses files, optimizes images, and removes unnecessary code. This not only reduces the overall size of the application but also enhances the loading speed, which is increasingly becoming a critical factor for retaining users.
Deployment Options and Strategies
When it comes to deploying a React application, various options are available, each boasting unique features that cater to different needs. Here are some of the well-known deployment platforms:
Netlify
Netlify is lauded as an easy-to-use platform that boasts a plethora of features tuned for developers. One specific aspect that sets Netlify apart is its continuous deployment from Git. This means that whenever you push updates to your repository, Netlify automatically builds and deploys the latest version of your application – a significant time saver.
Key Characteristic: The deployment pipelines reduce hassle significantly, making it a favored choice among developers procrastinating over server management.
Unique Feature: It provides instant global deployment via a Content Delivery Network (CDN), which significantly accelerates site performance.
Advantages/Disadvantages: Although Netlify is a great choice for static sites, it may incur costs when you exceed certain usage limits. Thus, developers should be mindful of their application’s growth and usage patterns.
Vercel
Another excellent choice is Vercel, which is particularly popular for its seamless integration with Next.js. This platform thrives on its simplicity and ability to handle server-rendered applications with ease. One specific aspect of Vercel that developers appreciate is its automatic scaling.
Key Characteristic: Vercel allows developers to focus more on coding while it manages backups and scaling as per their traffic.
Unique Feature: Preview deployments are another compelling aspect, enabling developers to see changes in real-time before making them live.
Advantages/Disadvantages: It’s also worth noting that while Vercel is user-friendly and great for developers, users should consider the learning curve if they aim to leverage advanced custom configurations.
AWS
Amazon Web Services, or AWS, offers a comprehensive suite of services for deploying almost any application – including React apps. One key aspect of AWS is that it provides a robust backend infrastructure, which is incredibly helpful for large applications with a critical need for stability and flexibility.
Key Characteristic: Its scalability is unmatched, catering to applications of all sizes, with services like Amazon S3 for storage and Elastic Beanstalk for easy deployment.
Unique Feature: Serverless architecture through AWS Lambda allows developers to manage application logic efficiently without concerning themselves with server maintenance.
Advantages/Disadvantages: However, AWS might feel overwhelming for developers just starting, due to its complexity and pricing nuances. Users need to be well-versed in its various offerings to get the best out of it, making it a choice better suited for more experienced developers.
Performance Optimization Techniques
In the world of web development, ensuring that your applications run efficiently and swiftly is paramount. For ReactJS developers, performance optimization is not just an afterthought but a critical aspect of the development process. An application that runs slowly can frustrate users and negatively impact their experience, leading to higher bounce rates and lower engagement. Therefore, understanding and implementing optimization techniques can significantly enhance the usability and speed of your React applications, ultimately translating to user satisfaction and retention.
Code Splitting and Lazy Loading
Code splitting is one of the cornerstones of optimizing performance in React. The main idea? To load only what is necessary when it’s necessary. Imagine you’re on a website that takes ages to load because it's trying to fetch a mountain of JavaScript, even if you’re only interested in a small portion of it. This situation can lead to a poor first impression and could turn away potential users.
By breaking your application into smaller chunks, you can make sure that only the required code is loaded at any given moment. This is especially useful in large applications. There are several ways to implement code splitting in React. One popular method is leveraging the function combined with . Here’s how it can be done:
Through this, you can render a component that is loaded lazily. The user will see a loading indicator if the component isn’t ready yet, which keeps them informed.
Lazy loading complements code splitting by delaying the loading of resources until they are needed. Instead of loading everything on the initial render, you can instruct your React application to load components, images, or other assets only when they're about to appear on the screen. This is particularly effective for images in long lists or galleries.
The benefits of employing code splitting and lazy loading include reducing the initial bundle size, significantly cutting down on load times, and improving perceived performance.
Memoization and UseMemo
Memoization is another vital optimization technique that can dramatically enhance the performance of React applications. The concept revolves around caching the results of function calls, so when the same inputs are provided, the cached result can be returned instantly rather than recalculating it. React provides the hook to facilitate this process.
Using ensures that expensive calculations are recalculated only when necessary. This can prevent unnecessary renders and keep the application running smoothly. For instance, if you have a component that performs heavy calculations based on a few input props, memoization can help by remembering the output based on those inputs. Here’s a simple example:
In this example, will only be called again if the prop changes. This can save a lot of performance overhead, especially when the calculation is intensive.
Utilizing these performance optimization techniques not only refines the responsiveness of your React applications but also elevates the overall user experience. By focusing on strategies like code splitting, lazy loading, and memoization, you can ensure that your applications are agile, efficient, and pleasant to navigate. As the landscape of web applications evolves, optimizing performance will continue to be a crucial aspect of ReactJS development.
Staying Updated with ReactJS
In the ever-evolving tech landscape, particularly when working with ReactJS, staying updated is not just a good practice; it’s essential. ReactJS has a vibrant community and an active development roadmap. Every time you blink, there’s a new update or discussion looking to refine or enhance the framework. Keeping pace with updates can greatly affect the effectiveness and efficiency of your projects.
Staying informed allows for the integration of the latest features, tools, and best practices into your workflow. This can lead to improved performance, easier debugging, and maintaining a modern codebase that adheres to recent security protocols. Essentially, consistent learning becomes the backbone of a successful development environment. Here are a few focused approaches to ensure you're always on the cutting edge:
- Adapt to New APIs: React introduces new features frequently, such as hooks and concurrent mode. Understanding these can help enhance your application’s responsiveness and performance.
- Refine Performance: Updates often come with performance improvements. By keeping up, you can implement these optimizations swiftly.
- Critical Bug Fixes: React’s updates often address known bugs. Failing to update might mean your application is vulnerable or not performing at its best.
Following Official Documentation
React’s official documentation serves as the bedrock of learning the framework effectively. This treasure trove is constantly enhanced to reflect changes in the React ecosystem. By regularly consulting the official React documentation, you can gain insights directly from the developers who design and maintain the library. Here’s why it’s indispensable:
- Accurate Information: It’s kept up-to-date with the latest features and best coding practices, providing reliable insights that are more current than many blogs or forums.
- Examples and Tutorials: The documentation has a wealth of examples which succinctly indicate how to implement features correctly. These examples can be golden and save you from unnecessary trials and errors.
- Problem-Solving Guidance: When you run into issues with your code, the documentation can often provide the breakthrough you need. Search for a specific component or API, and the answer is most likely there.
Engaging with the React Community
The React community is expansive and incredibly supportive—it’s like having a massive think tank right at your fingertips. By engaging with fellow developers through platforms like Reddit, GitHub, and even on Twitter or Facebook, you open yourself up to different perspectives and insights. Here’s why community engagement can be a game changer:
- Networking Opportunities: Connecting with others in the field can lead to collaborations. Who knows? Engaging in discussions might even land you a project or job opportunity.
- Problem-solving Collaborations: Discussing challenges with others can offer immediate solutions. The chances are high that someone else in the community has navigated similar roadblocks.
- Access to Real-world Tips: Often, community members share personal experiences, real-world applications, or non-official hacks that can greatly enhance your understanding or framework usage.
"An engaged community doesn't just learn together; they grow together."
Common Challenges in React Development
Setting up a React development environment comes with its own set of hurdles. Understanding these challenges not only prepares you for the bumpy ride but also provides a path to smoother sailing in the long run.
From debugging multifaceted codebases to optimizing performance metrics, it's vital to recognize common issues early. This section serves as a guide to identify these pitfalls, ensuring a better grasp of the development process. By confronting issues head-on, developers can foster productivity and efficiency, steering their projects toward success.
Identifying Common Issues
When diving into the world of React, developers often stumble upon various obstacles that can stall progress. Some of the most frequently encountered problems include:
- State Management Confusion: Understanding how to manage components' local and global states effectively can leave developers scratching their heads.
- Performance Bottlenecks: A sluggish app can deter users. It's crucial to recognize when and how performance dips occur, especially as complexity increases.
- Versioning and Compatibility: With continual updates to libraries and frameworks, keeping projects compatible with the latest versions can be a high-wire act.
- Debugging Complexity: Errors can be elusive, often buried deep within nested components or unique render cycles, making them difficult to track down.
Developers may experience these issues in different manifestations depending on their project setups and existing knowledge. That's why pinpointing problems early is crucial; it allows for timely interventions rather than chaotic post-mortems.
Best Practices for Troubleshooting
Troubleshooting can often feel like finding a needle in a haystack. Here are some solid practices to make this task less tedious and much more systematic:
- Use Console Logs: Don’t underestimate the power of the console. Logging the state or props of crucial components can help shine a light on troublesome areas.
- Isolate Components: Breaking down complex components into smaller, testable parts can help isolate the issue. It’s easier to manage smaller chunks than a hulking monolith.
- Leverage React Developer Tools: Using the React Developer Tools extension can help monitor component hierarchies and states. It's invaluable for quickly identifying where things start to go awry.
- Check Dependencies: Sometimes, issues could arise due to outdated or improperly installed dependencies. Always ensure that the dependencies align with the version of React you are using.
- Seek Help from the Community: Engaging with forums like Reddit can connect you with experienced developers who might have faced similar challenges. You might find that the solution to your problem is just a post away.
"Problem-solving isn’t about avoiding issues; it’s about tackling them creatively and with persistence."
By committing to these best practices, you're not just firefighting; instead, you’re building a sustainable approach to prolonged development processes. Over time, mastering these techniques will reflect in your code quality, ultimately leading to more robust and maintainable applications.
Finale and Best Practices
Importance of Ending and Best Practices
In the tech world that evolves at breakneck speed, best practices serve as guiding stars for both new and seasoned developers. They help in mitigating the risks associated with coding errors, dependency mishaps, and environment configuration failures. As teams grow or projects expand, a failure to adhere to best practices may lead to chaos.
The conclusion synthesizes the key insights gained from this guide, reinforcing the necessity of configuring a suitable environment. It encourages developers to not only set up their current workstations effectively but also think ahead about scalability and maintainability. The importance of documentation, for instance, is often underestimated. A well-documented process can save time and effort in future projects.
Summary of Key Takeaways
- Workflow Efficiency: An organized development environment accelerates workflow, minimizing bottlenecks and frustrations.
- Tools and Libraries: Familiarity with essential tools like Git, npm, and React Developer Tools cannot be stressed enough - they can save significant time.
- Troubleshooting Awareness: Understanding common challenges and possessing the knowledge to address them swiftly enhances overall productivity.
- Scalability: Keep in mind that a well-set environment should adapt to growth and shifts in project requirements.
"An ounce of prevention is worth a pound of cure." This old adage rings true in the realm of software development, where preparation can substantially reduce the hours spent debugging later on.
Continuous Learning and Improvement
To keep pace with the dynamic landscape of ReactJS and web development in general, adopting a mindset of continuous learning is paramount. The technology stacks change, and new features are released frequently. Therefore:
- Follow Official Documentation: Ensure your resources are up-to-date by regularly consulting official documentation to stay abreast of the latest changes and recommendations.
- Engage with the Community: Platforms like Reddit offer rich exchanges of ideas and troubleshooting advice. Don’t hesitate to participate.
- Experiment: Set aside time for side projects or exploration of new libraries and tools. Practical hands-on experience fosters understanding beyond the theoretical.
- Feedback Loops: Seek feedback from peers about your practices and setups. What seems ideal for you might not work for someone else; adapt and refine based on collective insights.
In summary, the end of one project merely marks the beginning of your next education phase. By actively integrating these practices and maintaining a focus on improvement, you not only enhance your skill set but also contribute positively to your projects and teams.