Mastering Conditional Logic with Python's If Statement
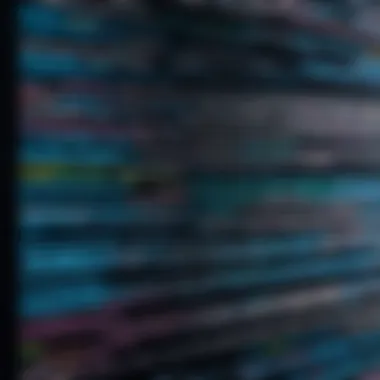
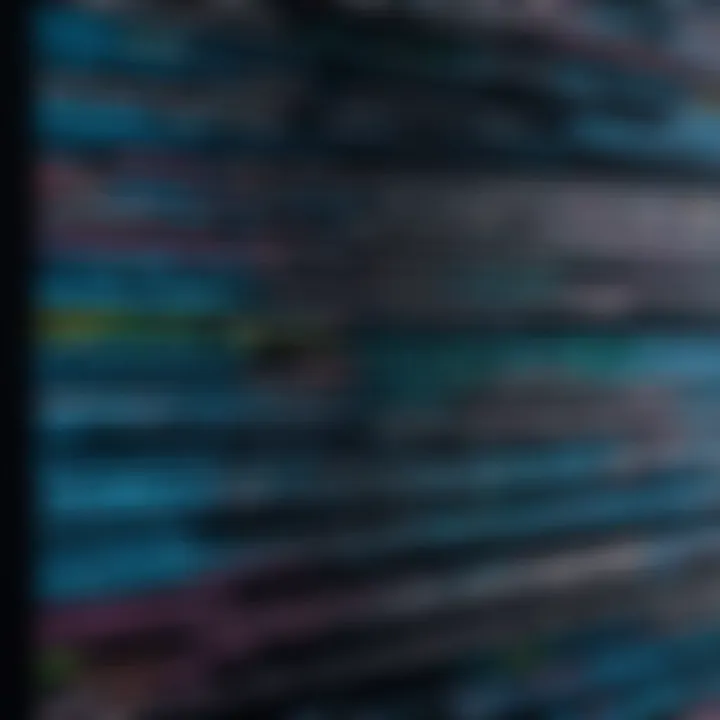
Intro
In the realm of programming, the ability to make decisions is a cornerstone skill. At the heart of this decision-making process in Python lies the if statement. Understanding this fundamental concept not only equips developers with the tools to write effective code but also opens doors to more advanced programming techniques. Whether you are crafting a simple script or diving into complex algorithms, mastering the if statement is essential.
As we explore this topic, we will unpack the syntax and practical applications of the if statement. This will include everything from interpreting the basic structure to understanding intricacies that seasoned programmers use for sophisticated logic flows. The intent is to provide a clear and approachable guide, helping both novices and veterans alike to refine their skills and enhance their programming prowess.
Conditional statements are the bedrock of logical operations in programming. They allow your code to react dynamically based on different conditions. This ability to dictate the code's flow based on input or states makes it an invaluable asset in a programmer's toolkit.
In this article, we aim to demystify the if statement, shedding light on its syntax, variations, and practical uses. By the end, youāll not only be comfortable with the basics but also ready to implement more nuanced applications of conditional logic.
Letās dive into the intricate world of the if statement, unlocking its potential in your Python programming journey.
Prolusion to Conditional Statements
In the realm of programming, conditional statements play a crucial role, serving as the backbone of decision-making processes. The if statement, in particular, stands out as one of the most fundamental elements of control flow in Python. It allows programmers to dictate how their code responds to various conditions or inputs, enhancing its functionality and adaptability. Without this capability, software would operate without regard to user input or logic, rendering it rigid and unresponsive.
By understanding conditional statements, developers gain the power to create dynamic programs that analyze data, respond to user behavior, and ultimately deliver a more tailored experience. This flexibility is particularly significant in todayās programming landscape, where speed, efficiency, and user engagement are paramount.
Moreover, knowing how to effectively implement these statements opens the door to more complex programming concepts. As we delve into the specifics of the if statement, you'll discover how to harness its potential to facilitate everything from basic error-checking to sophisticated decision-making algorithms. This knowledge not only simplifies the logical flow of your applications but also cultivates a robust foundation for mastering Python.
Conditional statements are the tools that allow developers to think dynamically and create software that truly interacts with users.
In the following sections, we will break down the if statement, explore its syntax, uncover its execution, and study various applications and common pitfalls. This comprehensive approach will empower you to integrate conditional logic into your Python projects seamlessly.
Overview of the If Statement
When it comes to programming, the ability to make decisions is paramount. The if statement stands at the core of this capability in Python. Understanding this foundational concept is not just beneficial; it's essential. The if statement allows a program to execute certain actions based on whether a condition holds true. This simple yet powerful tool is what transforms static code into dynamic, responsive applications.
Importance of the If Statement
The if statement facilitates flexibility within your code, enabling programs to react differently depending on the circumstances. For instance, imagine a simple shopping cart application that offers discounts during a sale season. An if statement checks if the current date falls within a given range before applying a discount. Without it, the program would lack the ability to adapt to changing conditions, essentially operating in a one-size-fits-all manner.
Additionally, learning about the if statement equips you with essential problem-solving skills. Every time you encounter a conditional situation, you're honing your ability to think critically and logically. By understanding how to effectively implement if statements, you prepare yourself for more complex programming constructs later on, paving the way for mastering advanced algorithms and data manipulation techniques.
"Programming isn't just about writing code; it's about solving problems. The if statement is a fundamental tool in that toolkit."
As we delve deeper into the if statement, weāll discover its syntax, how to execute it, and the nuances that come with it. Grasping these concepts will enhance not only your programming skills but also your overall ability to think like a developer.
Basic Syntax of an If Statement
When working with the if statement in Python, the syntax is straightforward, resembling everyday language. Hereās how it basically looks:
This syntax lays the groundwork for decision-making in your code. The can be any logical expression that evaluates to either True or False. For instance, if you wanted to check whether a user is eligible for a certain feature, you'd write:
This simple illustration emphasizes that the code block- indented under the if statement- only executes when the condition is satisfied.
Executing an If Statement
Executing an if statement hinges on understanding how Python evaluates the condition. It's crucial to comprehend that Python performs a boolean evaluation. When your program hits an if statement, it checks the condition. If itās true, the following block of code runs. If itās false, the program bypasses that section and may continue with other instructions.
Consider this example:
In this case, the output "It's a warm day!" will only print if the temperature exceeds 25. If you change the variable to 20, nothing will be displayed. Itās as simple as that.
To summarize, the if statement in Python is not just a mere tool; it's the backbone of control flows in programming. Knowing how to wield it effectively can hugely impact the functionality and responsiveness of your applications.
Components of an If Statement
Understanding the components of an if statement is essential for mastering conditional logic in Python. It lays the groundwork for how decisions are made in your code. Grasping these elements makes coding more efficient and also helps in avoiding common mistakes. In programming, communicating intent through clear and concise logic is crucial. It's not just about getting the right answer but also ensuring others can easily read and understand what the code is doing. Let's dive deeper into two critical components: logical expressions and indentation.
Logical Expressions in If Statements
Logical expressions form the backbone of any if statement in Python. These are the conditions that decide whether a certain block of code should run or not. When you see an if statement, what follows is a logical expression. It's like the gatekeeper, checking whether the conditions are satisfied before moving on.
Here are a few key types of logical expressions:
- Comparison Operators: Used to compare two values. Common ones include , , ``, , , and . For example, evaluating if is greater than uses .
- Logical Operators: These are used to combine one or more conditions. The common logical operators include , , and . For example, checks if a person is an adult but not senior.
- Membership and Identity Operators: These determine if a value is part of a collection or if two variables refer to the same object. Operators like and can be quite handy. For instance, checks if 'apple' is among the items in .
When structured correctly, logical expressions can enhance the decision-making process of your program, leading to more intuitive and functional code.
Indentation in Python
Now, letās shift gears to indentation, which is often an overlooked but critical component in Python coding. Unlike many other programming languages that use braces to define blocks of code, Python relies heavily on indentation. This is not just a matter of style; itās a matter of functionality. In Python, indentation determines the scope of the if statement, defining which code belongs to that particular conditional branch.
A common pitfall with indentation involves misalignments, leading to syntax errors or, worse, logical errorsāwhere the code runs but produces unexpected results. Here are some important considerations:
- Consistency is Key: Always use the same number of spaces for indentation. Whether it's four spaces or a tab, just be consistent throughout your code. Mixing them can lead to confusion.
- Use Spaces over Tabs: Although both are acceptable, it is generally best practice to avoid tabs. Most coding environments default to spaces. Plus, spaces display uniformly across different platforms, whereas tabs can vary.
- Indent for Clarity: Itās not just about meeting requirements. Properly indented code increases readability, making it easier for others (or even yourself at a later time) to understand the logic behind your code.
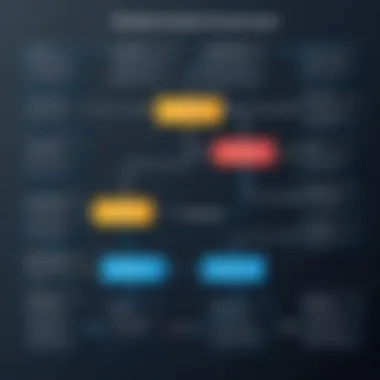
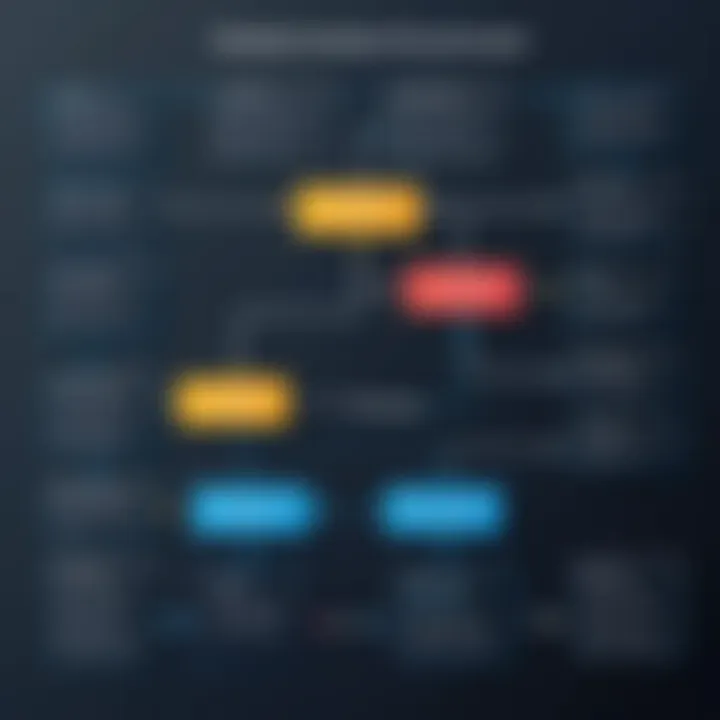
Important Note: In Python, failing to indent correctly can turn a functioning program into a maze of errors. Always check your alignment!
In summary, both logical expressions and indentation are essential parts of crafting effective if statements. Mastering these components not only makes your code run correctly but also contributes to developing clear and understandable programs. This clarity can save time and frustration in debugging and maintenance efforts down the line.
Variations of If Statements
In programming, versatility is a precious asset. The variations of the if statement in Python allow developers to create more complex decision-making processes in an elegant manner. Understanding these variations is crucial for any programmer, as they serve not only to simplify code but to enhance readability. The essence of programming is taking conditions that are often straightforward and wrapping them in logic that processes information swiftly, making usersā lives easier.
The Else Clause
The else clause offers a way to define an alternative action when the if condition is not met. It effectively creates a binary decision path, simplifying the logic behind conditions. Hereās how it works: after an if statement evaluates a condition, Python looks for an else clause. If the condition holds true, the block of code under the if executes; if not, it swiftly jumps to the else clause.
Consider the scenario of checking a userās age:
In this example, is is clear cut. If the age is 18 or more, the output reflects adulthood; otherwise, the output speaks for youth. This simple conditional logic augments decision-making in a user-friendly way. The beauty lies in far more than binary outcomes, though. You can think of else as a safety net, ensuring that your code always has a defined behavior, regardless of the input. That said, itās important not to misuse it. Adding unnecessary else clauses can introduce clutter, so keeping the code clear is paramount.
The Elif Clause
As conditions grow, so do the needs for more selective paths than a simple binary choice can provide. Thatās where the elif clause comes into play. Short for "else if," this clause allows for multiple checks in a single conditional structure. A programmer can chain together as many conditions as necessary, refining the decision-making process with clarity and ease.
Hereās a classic example to illustrate how elif can be particularly useful:
In this instance, each grade threshold is evaluated stepwise. The if statement checks for an A, Ų„Ų°Ų§ it fails to meet that, elif checks for B, and so forth. This layered approach is not just more manageable; it provides clarity when navigating complex decision trees. When using elif, it is essential to keep in mind the order of checks, as Python will execute the first condition it encounters as true; subsequent conditions will be ignored, which could lead to unexpected results if not well thought out.
In summary, variations of the if statement, particularly with the incorporation of else and elif, allow programmers to craft articulate and precise paths through their code. As you harness these tools, strive to maintain clarity. Remember, and the clearer the code, the easier it is to maintain and update, a principle that will always serve you well in programming.
Practical Applications of If Statements
When it comes to programming in Python, understanding the practical applications of if statements is key to building robust and responsive code. This section emphasizes how if statements serve as the backbone of decision-making in various scenarios, from interactive user interfaces to data manipulation tasks. By harnessing conditional logic effectively, developers can ensure their applications respond aptly to a plethora of situations, enhancing both functionality and user experience.
Using If Statements in User Input Scenarios
In every application where user interaction is involved, if statements reign supreme. For instance, consider the various responses an application can provide based on user input. If a user is asked to input their age, the program can determine which services or content to present based on whether they are above a certain age or not. Hereās an example demonstrating that:
In this snippet, depending on the age the user inputs, the message displayed will change. This not only guides the user properly but also ensures that the application can dynamically adjust to different situations. This is a prime example of how if statements shape the interface, granting it responsiveness.
Conditional Logic in Data Processing
Data processing is another realm where if statements shine. As data sets can vary widely, applying conditional logic allows for tailored analysis and reporting. If you're processing sales data, for instance, your program might need to apply different calculations based on the sales thresholds.
Imagine you are analyzing whether sales figures hit a particular target. A simple if statement can decide whether to apply a bonus to employees:
In this scenario, if the sales exceed $10,000, it triggers a calculation for a bonus. If not, the program communicates back the lack of a bonus clearly. This showcases how conditional statements can influence operations based on specific criteria, making data-driven decisions smooth and effective.
Practical applications of if statements are pivotal in both user interactions and data analysis, showcasing the essence of conditional logic in modern programming.
Common Mistakes to Avoid
Understanding common pitfalls when using the if statement in Python is crucial for any programmer. Avoiding these mistakes not only leads to clearer code but also enhances the debugging process and improves overall efficiency. The insights drawn from identifying these errors help prevent wasted time and effort when developing complex applications. Remember, commonly encountered issues can make even the simplest tasks feel like navigating a minefield. Here are some common mistakes that Python coders should steer clear from.
Incorrect Indentation Issues
Indentation is a cornerstone of Pythonās syntax and yet also a frequent trouble spot for many. In Python, indentation isnāt just about aesthetics; it defines the blocks of code. If you mix spaces and tabs, or if the indentation levels are inconsistent, Python will throw an "IndentationError" faster than you can say "bug fix!"
Hereās an example that clearly illustrates this:
In the above snippet, the print function on the second line has an incorrect indentation level which will disrupt the flow and lead to confusion.
Therefore, to avoid such pitfalls, keep your indentation uniform throughout your code. Here are a few tips for maintaining proper indentation:
- Stick to either tabs or spaces: Choose one method and remain consistent.
- Use an IDE: Many integrated development environments highlight indentation levels and can auto-format code accordingly.
- Check settings: Verify your code editor's formatting settings, especially if you're collaborating with others.
Logical Errors in Conditions
Logical errors can be a programmer's worst nightmare. Unlike syntax errors, which are easily identified by Python, logical errors can silently wreak havoc in your code. You might write a condition that seems perfectly valid but behaves unexpectedly due to a minor oversight.
Consider this example:
This snippet will print "You are an adult" as expected given the age of 20. But imagine a situation where age is determined by user input:
In this case, the program will fail to evaluate correctly because the input function returns a string. To fix it, you would need to convert the input to an integer first:
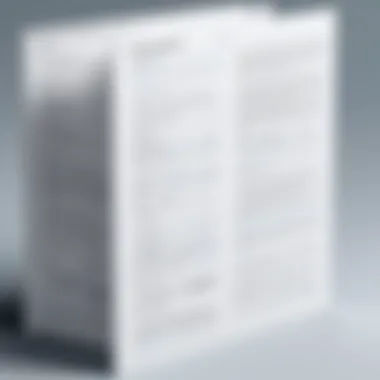
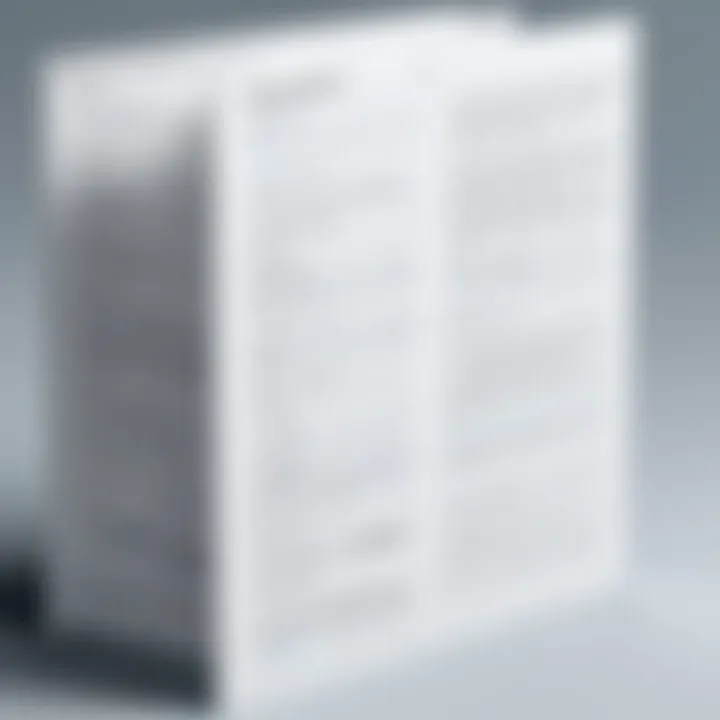
Hereās a few ways to dodge logical errors:
- Be clear with data types: Always ensure that the values being compared are of the same type.
- Print diagnostic information: Use print statements to track variable values and understand the flow of logic.
- Utilize assertions: Assert statements can help identify logic flaws during development.
"An ounce of prevention is worth a pound of cure." Better understanding and prevention of these mistakes can save you countless hours of debugging in the long run.
Advanced Conditional Statements
In the world of Python programming, mastering advanced conditional statements is not just about knowing the basics. Itās about taking that foundational knowledge and extending it to leverage complex decision-making. This part of the article focuses on two significant aspects: nested if statements and the use of multiple conditions. Understanding these advanced techniques is vital as they allow for more nuanced control over code execution.
Utilizing advanced conditional statements can lead to more efficient code. If your program can process various scenarios with fewer lines, thatās a win for readability and performance. Moreover, as projects become more intricate, incorporating these advanced techniques can significantly reduce the likelihood of mistakes that arise from oversimplified logic.
Nested If Statements
Nested if statements enable you to check additional conditions within an if statement. This structure creates a hierarchy, where one condition can lead to further evaluations. Itās like peeling back layers of an onion to find the core. For example, imagine you have a situation where you want to verify a userās status based on multiple levels of security clearance.
Hereās how you might represent that in code:
In this example, the code evaluates the user clearance step by step. If the user is not an admin, it goes deeper to check if they're a moderator. If neither, it defaults to basic resources. This layered approach helps manage complex logic flows and can be quite powerful. Yet, keep in mind that readability might suffer if the nesting gets too deep. This could confuse anyone reading the code later.
Using Multiple Conditions
When it comes to decision-making in programs, sometimes one condition isnāt enough. Python allows you to evaluate multiple conditions using logical operatorsā, , and . This flexibility lets you craft intricate conditions with ease.
For instance, if youāre building an online store, you may want to check both the availability of a product and whether a user is a premium member. Consider the following code snippet:
This example illustrates how using multiple conditions can help in making more informed selections in your code. By clearly outlining the logic related to both and , we handle different scenarios seamlessly. Moreover, using and provides a strong toolset for programming logic, letting developers express themselves crisply and succinctly.
Key Takeaway: Advanced conditional statements, through nested statements and multiple conditions, allow for robust decision-making capabilities in programming, opening doors to sophisticated logic flows that better cater to varied scenarios.
Best Practices for Writing If Statements
When it comes to writing if statements in Python, it's not just about getting the code to run; itās about making sure it runs well and is easy to read. Best practices in writing if statements can significantly improve code quality, making it more maintainable and understandable. Effective if statements set the tone for the program, enabling developers to handle decisions succinctly and clearly. Here, we dive into the fundamental aspects of writing effective if statements that every programmer should keep in mind.
Keeping Conditions Simple and Clear
One of the paramount rules in programming is to keep it simple. Complex conditions can lead to confusion and, in the worst case, bugs that may take hours to track down. The goal should be to write conditions that are both comprehensible and concise. Hereās a few things to consider:
- Limit the Length: If a conditional statement becomes too long, consider breaking it into smaller parts. Instead of trying to cram everything into one statement, you can derive a boolean variable that represents part of your condition.
- Use Descriptive Variable Names: Naming matters! If a variable clearly indicates its purpose (like ), it becomes easier to understand the intent of the condition, leading to code that reads like a story.
- Avoid Negation: Writing complex negations can lead to misunderstandings. For instance, instead of writing something like , you might write . This keeps it clearer and may save your readers from mental gymnastics.
"Simplicity is the ultimate sophistication." - Leonardo da Vinci
By following these simple guidelines, programmers can prevent misinterpretations and make their code more intuitive, ultimately making life easier both for themselves and their colleagues.
Commenting on Complex Logic
Even with the best practices, there are times when you canāt escape complex logic. In such cases, itās crucial to document your thought process through comments. Poorly-commented code is like a book without a table of contents: frustrating. Hereās how to effectively comment on complex logic:
- Purpose Over Explanation: Comments should explain why you chose a particular logic flow rather than how it works. For example, instead of writing a line-by-line description of your code, focus on the underlying rationale behind it. This helps future developers who might need to modify your code understand your thinking.
- Use Block Comments: When a chunk of code warrants it, consider using block comments to outline the sections of complex logic. This breaks down large conditions into easily digestible parts for someone who might be skimming through.
- Tag the Known Issues: If your logic has known edge cases or potential pitfalls, clearly state them in the comments. For instance, you might say: "# TODO: Handle case when user has no profile picture."
Remember, comments are there to help, not drown out the code. Well-placed comments serve as signposts, guiding onlookers through the labyrinth of your code. By implementing these best practices, programmers can write if statements that are not only functional but also maintainable and easily understandable.
Real-World Examples
Real-world examples bring abstract concepts to life, helping us to grasp the practical applications of what we learn. In programming, especially when it comes to conditional statements like the if statement in Python, seeing concrete use cases can illuminate their importance and functionality. This section will dive into two specific areas where if statements shine: game development and web applications. Understanding these applications will not only reinforce the theoretical aspects but also enhance your coding repertoire.
If Statements in Game Development
In the realm of game development, if statements are the backbone of interactive gameplay. They allow programmers to dictate the flow of actions based on player choices and game states. For example, consider a simple scenario in a role-playing game: if a playerās character has enough health points, they can attack; if not, they must heal. Hereās how that might look in code:
This block checks the player's health and executes different functions depending on the condition. Itās a basic but effective demonstration of how if statements control game logic.
Moreover, if statements can be incorporated in more complex game mechanics, such as AI decision-making. A non-playable character (NPC) could use an if statement to determine how it reacts to the player:
- If the player is close, the NPC might attack.
- If the player is far away, the NPC may choose to retreat or seek cover.
Such conditional logic can greatly enhance the gaming experience, making gameplay seem more dynamic and responsive.
Conditional Logic in Web Applications
Web applications utilize if statements to manage user interactions and responses effectively. They can tailor content based on user input or system state, making for a highly customizable experience. For instance, when a user submits a form on a website, if statements help ensure that the information is valid before proceeding to the next step.
Imagine a contact form where the user must input an email address. The code might look something like this:
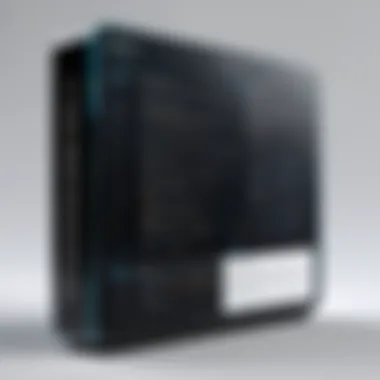
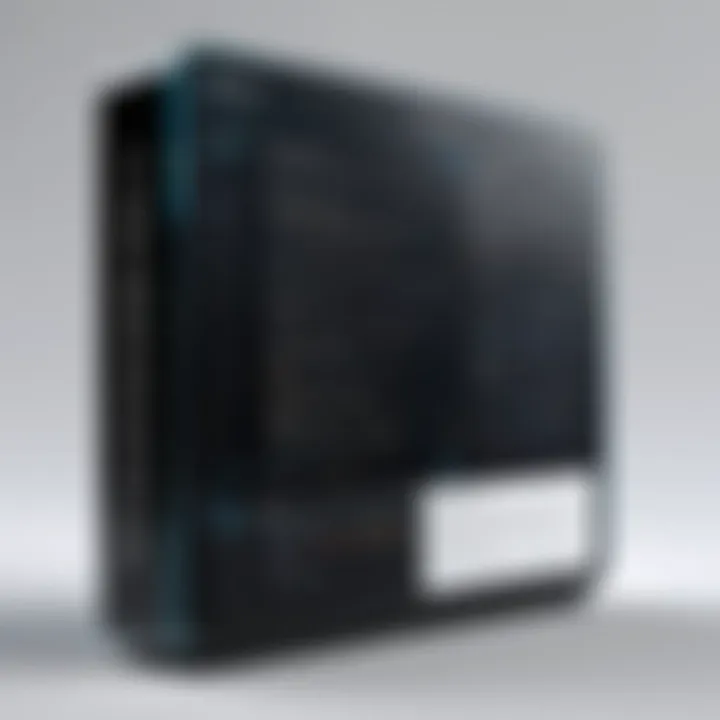
In this scenario, the program validates the email and either saves the information or displays an error message based on the outcome of that validation. This responsiveness is essential for user experience and can prevent potential errors before they affect system performance.
Additionally, if statements can dictate the visibility of certain features based on user roles. For example, an admin user might see more settings than a regular user. This conditional visibility enhances security and usability, guiding users based on their permissions.
"In programming, understanding the nuances of conditional statements like if statements not only bolsters your coding skills but also empowers your creativity."
Using real-world examples like game development and web applications illustrates the vast and practical uses of if statements. They are not just tools, but integral components that drive engagement and functionality in everyday programming tasks.
Testing Conditional Statements
Testing conditional statements is not just a routine step in programming; it lays the foundation for a robust application. Without proper testing, even minor logical errors can spiral into significant pitfalls. This is especially true in complex applications where a simple statement can determine the behavior of entire segments of code. Thus, understanding the best practices for testing these statements is crucial for any developer aiming for high-quality code.
One of the fundamental benefits of testing conditional statements is the early identification of bugs. When you write tests alongside your code, it allows you to confirm that each condition leads to the desired outcome. This proactive approach can save time and resources later in the development process. By frequently testing your code, you can catch those elusive bugs before they make a grand entrance into your working environment.
Unit Testing If Statements
Unit testing plays a pivotal role in ensuring each piece of your codebase is doing its job. For statements, unit tests evaluate the conditions and outcomes defined in your code snippet. A well-written unit test for an statement can appear quite straightforward:
Here, we define a simple function , and our tests verify if our conditions return the correct values. The code checks if numbers given are correctly identified as even or odd, reflecting the logical flow built-in the statement. If the tests fail, you catch errors before promoting to production, which often lowers the risk of unrecognized issues lurking in the code.
Itās also recommended to evaluate the edge cases while writing your tests. For instance, the input type could be other than integers, like strings or lists, and testing how your statements handle these will highlight any shortcomings in your logic early on.
Debugging Techniques for If Statements
Debugging is an inevitable part of programming. Even the best of us trip over the odd logical misstep or an unexpected behavior in our code. The key is to know how to address these hiccups. A variety of techniques exist to help diagnose problems in your statements.
- Print Statements: Sometimes itās as easy as adding a statement to show what your variables are holding at that moment. This method can unveil if the expected logic is following through or being bypassed altogether.
- Using Debuggers: Tools such as , the Python debugger, allow you to step through your code line by line. This helps visualize what part of your statement is being executed and where the logic might be going astray.
- Test-Driven Development: Writing your tests before implementing the function can conflict less with the final logic. If your tests fail, itās a clear indication that your statements need to be reevaluated. This proactive methodology often leads to fewer debugging sessions later on.
- Logical Flow Check: Drawing a flowchart may also serve as a crystal-clear visual aid to see how your logical statements link up. Placing checks and balances helps clarify where the discrepancies stem from, as well.
Debugging isn't just about fixing problems but understanding your code's behavior in a dynamic context. Each approach can yield a variety of insights, enabling you to refine your logic over time, therefore enhancing the overall structure of your applications.
Testing and debugging when it comes to conditional statements are skills that sharpen with experience. Immersing oneself in best practices can drastically bolster not only the quality of your code but also your efficiency as a programmer.
Exploring Alternatives to If Statements
Conditional statements, particularly the if statement, are a cornerstone of programming logic. However, there are times when exploring alternatives to these statements can be beneficial. This isnāt just about looking for a shortcut; itās about enhancing readability, simplifying code, and even optimizing performance in certain scenarios. By delving into alternatives, programmers can broaden their toolset, learn to think differently, and often find more elegant solutions to problems.
Using Ternary Operators
Ternary operators provide a compact way to evaluate conditions and return values based on those evaluations. Unlike traditional if statements, which require multiple lines, a ternary operator condenses this into a single expression. The general syntax in Python looks like this:
For example, letās say you want to assign a value based on a user's age:
This one-liner checks the age and assigns "Adult" if the condition is true, or "Minor" otherwise. Itās concise and can make your code cleaner, especially in cases where you need to return a value based on a condition and donāt require a full if-else block.
Benefits of Ternary Operators:
- Conciseness: Reduces lines of code, making it easier to read at a glance.
- Inline Evaluation: Keeps related logic closely tied together, providing more context.
- Enhanced Readability: For simple conditions, it can make the intent clearer.
Despite these advantages, it's crucial to note that overusing ternary operators or utilizing them for complex conditions can lead to confusion. As with all tools, know when it is appropriate to use them, and ensure the simplicity remains in execution.
Switch Statements in Other Languages
While Python does not natively support a switch statement like C or Java, understanding how these work in other programming languages can provide insight into alternative logical structures.
A switch statement offers a way to dispatch execution based on the value of an expression, making it potentially more efficient than a long series of if-elif statements. The syntax typically resembles this example in C:
This structure can improve performance in scenarios where you are checking against known values, and it often improves the readability of the code, especially when dealing with multiple conditions.
Although you can achieve similar functionality using if statements in Python, like this:
The switch statementās approach may lead to cleaner, more organized code, especially in cases requiring extensive conditional checks. Languages like JavaScript now support similar constructs with , which some developers find useful when implementing state machines or routing logic.
Closure
The conclusion of this article serves as a pivotal point to synthesize the insights gleaned from our exploration of the if statement in Python. Understanding if statements, along with their variations and implications, emboldens programmers to build more dynamic and responsive applications. This functionality is foundational in programming; it creates a framework in which decisions can be made based on specific criteria.
Recap of Key Points
To wrap up, letās revisit some core ideas we discussed throughout the article:
- Basic Syntax: We delved into the straightforward structure, showcasing how even beginners can leverage simple syntax to initiate logical flows.
- Logical Expressions: Highlighting the significance of expressions within if statements helped clarify how conditions control program execution.
- Common Pitfalls: We addressed frequent mistakes, particularly around indentation and logic errors, enlightening developers on what to avoid.
- Advanced Concepts: The nuances of nested if statements and combining multiple conditions opened the door for more complex decision-making in code.
The reinforcement of these points not only clarifies the importance of the if statement but also lays a groundwork for further learning.
Future Outlook on Conditional Programming
Looking forward, the landscape of programming will continue to evolve. Conditional programming is likely to take on new forms as machine learning and artificial intelligence become increasingly prominent. These fields often integrate if statements and their variants in unique ways, adapting to vast data sets and user interactions.
Moreover, as developers seek to simplify code and improve efficiency, alternative structures might arise. Understanding standard practices now will serve as a foundation for embracing these future paradigms. With the rise of new programming styles and frameworks, programmers who master the if statement will find themselves well-equipped to navigate and adopt upcoming innovations.
In essence, mastering if statements is not just about coding; itās about developing a mindset for problem solving that will serve any programmer throughout their career.
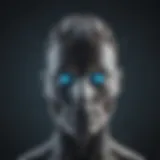
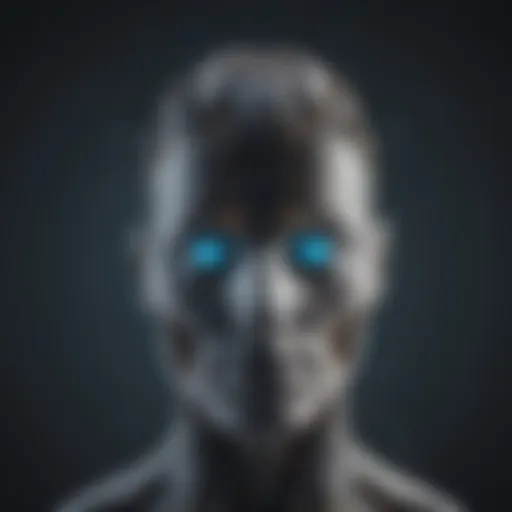