Mastering the Find Method in JavaScript
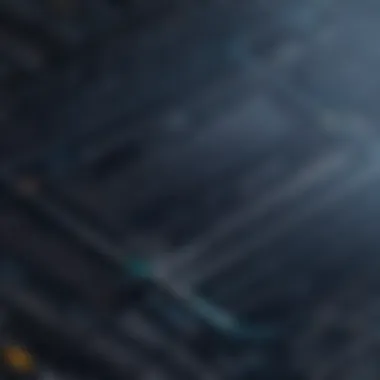
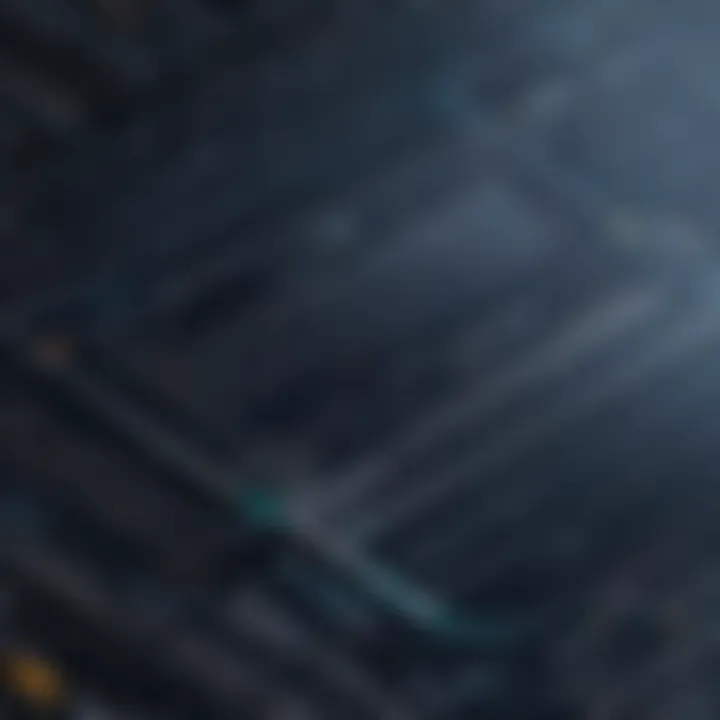
Intro
JavaScript has become a cornerstone of modern web development. Among its many features, the array methods have significantly enhanced how developers interact with data. One such method, known as find, serves a vital role in locating specific elements within an array. Understanding this method is crucial, as it directly influences the efficiency and readability of the code.
The find method operates by iterating through an array and returning the first element that satisfies a provided testing function. This process eliminates the need for more verbose looping techniques, leading to cleaner and more maintainable code.
In this guide, we delve into the intricate workings of the find method. We will explore its functionality, syntax, and practical use cases. Additionally, we will examine how it compares with similar methods like filter and map, as well as discuss performance considerations. This exploration will empower tech enthusiasts and industry professionals to enhance their skills in JavaScript array manipulation.
Intro to the 'find' Method
The 'find' method in JavaScript represents a significant advancement in the way developers can interact with arrays. This method is not merely a feature; it is a powerful tool that enables precise element retrieval based on defined criteria. By understanding 'find', programmers gain a valuable asset that enhances code readability and efficiency. In an era where data manipulation is crucial, the ability to quickly locate specific items in arrays simplifies many programming tasks.
What is the 'find' Method?
The 'find' method is an array function that comes built into JavaScript. It allows users to search for the first element in an array that meets a certain condition. Typically, a callback function is provided as an argument to define this condition. When 'find' executes, it traverses the array and applies the callback to each element until it identifies one that returns . If no elements satisfy the condition, it returns . This capability makes 'find' particularly useful for looking through arrays of objects or data structures.
Here's a basic example of how the method works:
In this case, the method locates the first number greater than 3. The simplicity of this syntax illustrates its ease of use and effectiveness.
Why Use the 'find' Method?
Using 'find' presents multiple advantages for developers looking to streamline their code. It enhances clarity by allowing for more straightforward logic when searching through arrays. Instead of using cumbersome loops or manual checks, 'find' abstracts this complexity into a single line of code.
Some key benefits include:
- Code Cleanliness: Using 'find' results in fewer lines of code, improving readability and maintainability.
- Performance Efficiency: For large datasets, 'find' can optimize search times by stopping at the first match rather than processing all elements.
- Flexibility: The method can handle complex conditions through custom callback functions, making it versatile across various scenarios.
For JavaScript developers seeking clean and effective solutions in their work, mastering the 'find' method is essential.
In summary, understanding the 'find' method is crucial for modern JavaScript development. It not only simplifies array handling but also enhances the overall quality of coding practices.
Understanding Array Methods in JavaScript
Array methods in JavaScript play a central role in handling data collections. As an essential part of the language, they allow developers to manipulate arrays with ease and precision. Understanding these methods lays the groundwork for utilizing the 'find' method effectively and efficiently.
The 'find' method is just one in a rich ecosystem of array methods, and grasping the larger context of these tools can enhance a developer's approach to problem-solving. With numerous built-in methods available, it is important to know their characteristics and how they can improve productivity. Beyond individual functions, the synergy created when combining these methods often yields elegant solutions to complex challenges.
Overview of Array Methods
Array methods in JavaScript include a wide variety of operations to process and manipulate array data. Functions such as , , , , and are notable for their ability to simplify tasks. Each method serves a distinct purpose, whether that be adding elements, removing elements, or transforming data.
For instance, the method is utilized for transforming each element of an array based on a specified function. This method returns a new array containing the results, leaving the original untouched, while condenses an array into a single value based on an accumulator function.
Understanding each method’s behavior, return values, and performance implications is key for selecting the appropriate tool for a given task. Detailed comprehension will aid in optimizing code.
Key Array Manipulation Methods
- forEach: Executes a provided function once for each array element. It offers a straightforward syntax but does not return a new array or modify the original.
- filter: Produces a new array containing all elements that pass the provided test. This method is excellent for use cases where data needs to be filtered based on specific criteria.
- find: Returns the first element in an array that satisfies the provided testing function. If no values satisfy the testing function, is returned. This method is crucial for locating specific data items in larger collections.
- some: Tests whether at least one element in the array passes the provided function. It is useful for checks where only a boolean result is needed.
- slice: Creates a shallow copy of a portion of an array into a new array object selected from the original array. This method is useful when needing to extract parts of an array without altering the original.
By mastering these key methods, developers can take full advantage of JavaScript’s capabilities, enhancing both the efficiency and clarity of their code. Each method encapsulates certain logic, allowing for cleaner and more maintainable scripts.
Syntax of the 'find' Method

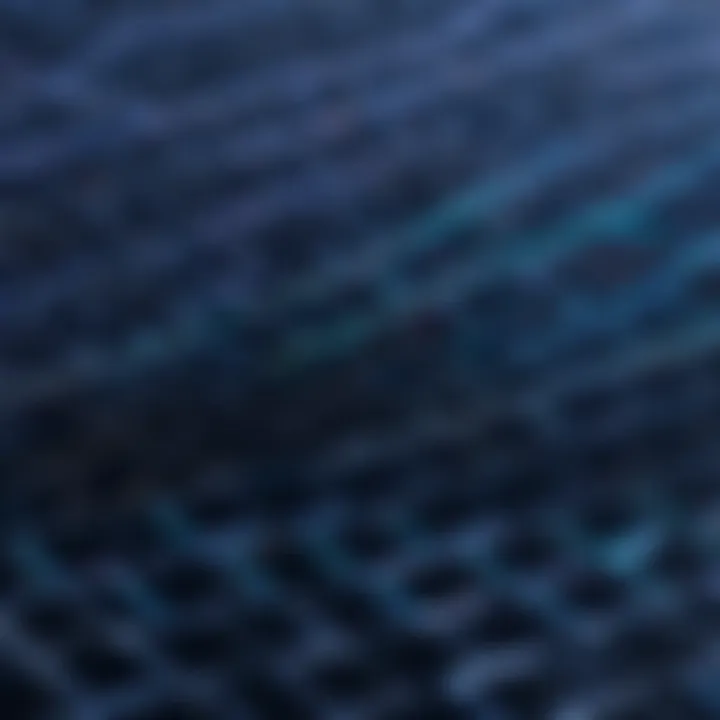
The syntax of the 'find' method in JavaScript is essential for understanding how to effectively utilize it in various programming contexts. This core aspect provides not just the structure but also the necessary parameters that define its operational capacity. Knowing the syntax allows developers to grasp its practical applications and integrate it seamlessly into their code. Moreover, a solid understanding of syntax promotes the writing of clean, efficient, and maintainable code, which is vital in any development project.
Basic Syntax Structure
The basic syntax of the 'find' method can be expressed as follows:
Here, the key components include:
- array: The array on which the method is called.
- callback: A function that is executed for each element in the array. This function takes up to three arguments:
- thisArg (optional): A value to use as when executing the callback.
- element: The current element being processed.
- index (optional): The index of the current element.
- array (optional): The array find was called upon.
The output will return the value of the first element in the array that satisfies the provided testing function, or if no elements pass the test.
Parameters Explained
Understanding the parameters of the 'find' method is crucial for leveraging its full potential. The callback function plays a pivotal role because it dictates what element will be found.
- callback: This is where the developer specifies the condition needed to find an element. For example, if you are looking for a user with a specific ID:
- thisArg: This optional parameter can be helpful when the callback uses to reference a specific context. For example:
The 'find' method is case-sensitive and only retrieves the first matching element, not all matches.
By understanding both the basic syntax and the parameters, developers can implement the 'find' method effectively in their applications, making it a powerful tool for various use cases.
How to Use the 'find' Method
Understanding how to effectively use the 'find' method is essential for anyone working with arrays in JavaScript. This method allows developers to search through arrays to locate an element that meets specific criteria. Its efficiency in finding elements makes it a preferred choice in various coding tasks.
Using 'find' simplifies the code and enhances readability. For instance, instead of looping through an array and using an if statement to check for a match, 'find' provides a clear one-liner that specifies the search condition directly.
Basic Example
To illustrate the use of the 'find' method, consider this simple example:
In this code snippet, we create an array of numbers. The 'find' method is used to locate the first number that is greater than 3. The callback function passed to 'find' checks each number in the array, returning 4 as it is the first element that satisfies the condition.
This example clearly shows how 'find' streamlines the search process, allowing the developer to focus on defining the condition rather than the looping mechanics.
Advanced Usage Scenarios
As developers grow familiar with the 'find' method, they can explore more advanced use cases. Here is a more complex scenario:
Imagine you have an array of objects representing users. Each object contains properties like 'id', 'name', and 'age'. If you want to find a user by their ID, you can do so with ease:
In this example, we look for a user whose ID is 2. Here, the 'find' method effectively scans the array of user objects and returns the entire object that meets the condition. This feature proves its utility in real-world applications where data is often composed of structured objects.
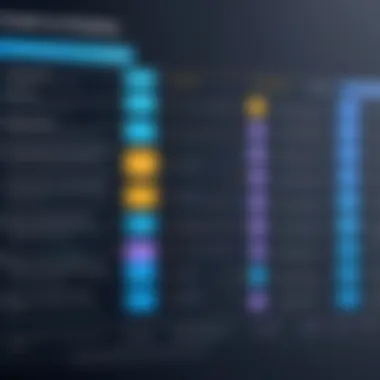
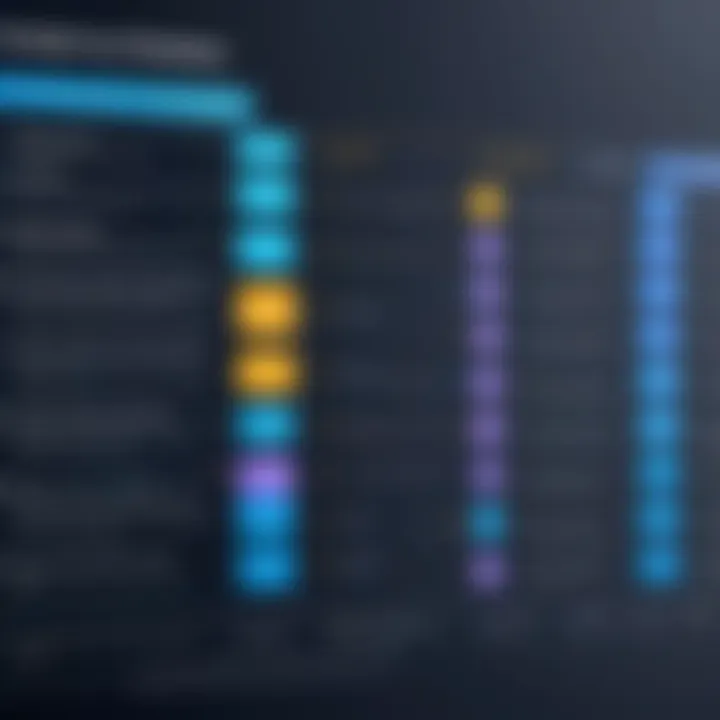
The 'find' method is best believed for clarity and shortness in code rather than performance.
Additionally, the 'find' method's combined ability to integrate with conditional logic opens up possibilities for user input validation. You can swiftly check if a specific input exists within an array and take further action based on the result. Overall, mastering the 'find' method equips developers with a powerful tool for simplifying array searches.
Common Use Cases for 'find'
The 'find' method in JavaScript is beneficial for many practical scenarios. Understanding the common use cases for this method enhances its usability in various coding situations. It directly aligns with tasks where locating specific elements within an array is necessary. This section will explore two primary scenarios: searching objects in arrays and validating user input. Each example will highlight how the 'find' method streamlines the process, offering notable efficiency and clarity.
Searching Objects in Arrays
When working with arrays consisting of objects, the 'find' method proves invaluable. In such cases, developers often need to extract specific information based on object properties. For example, if you have an array of user objects and need to find a user by their ID, the 'find' method can efficiently locate that object. Here is an example:
This snippet demonstrates how 'find' allows for direct access to the object without manually iterating over the array. This increases code clarity and reduces potential errors. Such simplicity is a significant benefit for developers who often juggle multiple data structures.
Validating User Input
Another important use case for the 'find' method is in validating user input. When accepting data, it is common to ensure the value entered meets specific criteria. For instance, if a form requires unique usernames, the 'find' method can check if a username is already taken. Consider this scenario:
Here, the code uses 'find' to determine if the username exists. If found, it indicates the username is taken. Otherwise, it confirms availability. This approach not only simplifies the validation process but also enhances user experience by providing immediate feedback.
The 'find' method is essential for efficiently managing arrays and validating user data. Its versatility makes it a core component of modern JavaScript programming.
In summary, the common use cases for the 'find' method illustrate its practical applications. By exploring searching objects in arrays and validating user input, we gain insight into the simplicity and efficiency this method contributes to coding practices in JavaScript.
Comparison of 'find' with Other Array Methods
The comprehension of the 'find' method is further enhanced through comparison with other array methods in JavaScript. Understanding how 'find' differs from those methods gives insights into its unique strengths and weaknesses. This section highlights the distinctions between 'find', 'filter', and 'some', illustrating when to use each method depending on the context of your application.
'filter' vs 'find'
Both 'filter' and 'find' serve the purpose of searching through arrays, yet they accomplish it in different ways. The primary distinction is that while 'filter' returns all elements that meet the specified condition, 'find' only retrieves the first element that satisfies the condition. This difference alone can impact performance and functionality.
For instance, if you want to find a specific user within an array of user objects where all users have an age property, using 'find' is efficient:
In contrast, 'filter' might be used when you want to get a list of all users above a certain age:
Consequently, the choice between 'filter' and 'find' is predominantly about the need for either a single element or a collection of elements. If performance is a concern, especially with large arrays, using 'find' may offer better efficiency when you only need one result.
'some' vs 'find'
The comparison between 'some' and 'find' is also significant to understand. While 'find' retrieves a specific element, 'some' only checks if at least one element in the array meets the condition; it returns a boolean value rather than the element itself. This can be useful for verification purposes.
For example:
In this case, 'some' will stop checking once it finds the first even number, making it efficient for such checks. On the other hand, if you need to locate that specific even number, you would use 'find'. This distinction highlights the utility of each method based on the requirements of your code.
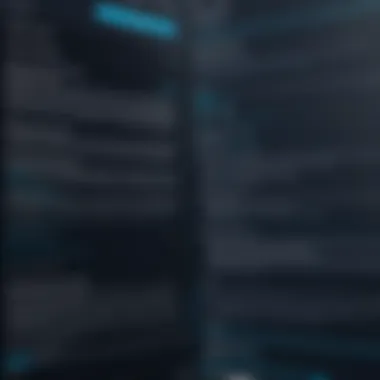
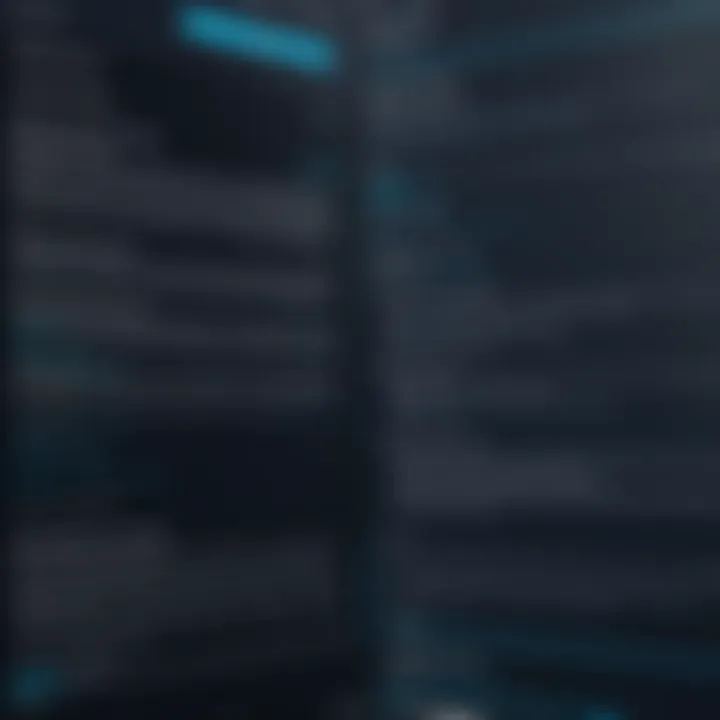
In summary, understanding the limitations and applications of 'find', 'filter', and 'some' can greatly enhance how you manage arrays in your JavaScript projects. Each method offers distinct benefits, making it crucial to select the right one based on your needs.
Performance Considerations when Using 'find'
When working with the 'find' method in JavaScript, performance considerations become essential. Understanding how 'find' operates can greatly impact the efficiency of your code, especially in larger applications with extensive data sets. Efficient code can save both time and resources, making it crucial for developers to grasp the nuances of the 'find' method's execution speed and its overall performance.
Time Complexity Analysis
The 'find' method operates with a time complexity of O(n), where n is the number of elements in the array. This linear time complexity arises because 'find' must iterate through the array until it finds the first matching element or reaches the end.
This means that in the worst-case scenario, where the desired element is at the last index or not present at all, the method checks each element, leading to possible performance concerns in large arrays. For instance, using 'find' on an array with millions of elements might take noticeably longer than on a smaller collection.
In practical scenarios, if you frequently need to retrieve elements from massive datasets, consider using a data structure with better lookup performance, such as a Set or a Map. This can reduce the need to repeatedly scan through large arrays, avoiding potential bottlenecks.
Best Practices for Optimization
To maximize the performance of the 'find' method, consider implementing the following strategies:
- Limit Array Size: Ensure that the array you operate on is as small as necessary. Unused elements yield performance loss, so filter data before executing 'find'.
- Utilize Efficient Predicates: The predicate function you pass to 'find' should be optimized for quicker evaluations. Return false as soon as possible to stop unnecessary processing.
- Cache Results: If the same search is repeated multiple times, cache the results instead of re-executing 'find'. This can greatly reduce computational time.
- Profile Your Code: Regularly use performance profiling tools to analyze execution times and identify potential slow spots in code. This data is crucial for optimizing your approach effectively.
By being mindful of how you apply the 'find' method, you can improve the efficiency of your applications and enhance the overall user experience.
Practical Examples and Applications
The find method is a powerful tool within JavaScript's array manipulation suite. Its efficacy lies in its ability to succinctly locate elements based on specified conditions. Practical examples and applications illustrate the method's significance, providing a bridge between theoretical understanding and real-world implementation. Exploring these practical scenarios enables developers to recognize opportunities where the find method can streamline their code.
Real-World Coding Scenarios
In numerous applications, the need to search for specific data within arrays arises. Consider a typical e-commerce platform where products are stored in an array of objects. Each product may have characteristics like id, name, and price. Using the find method allows a developer to quickly retrieve an item based on its id or any unique characteristic. Here’s a practical example:
The code snippet above effectively retrieves the smartphone object, demonstrating how the find method simplifies the search process. Moreover, this example reveals how neatly organized data can enhance code readability and efficiency. In more complex applications involving user experiences, such as a dashboard displaying user information, the use of find can make the task less burdensome when locating specific user records.
Integrating 'find' with Modern Frameworks
Modern JavaScript frameworks, like React and Vue, often handle complex data sets. Integrating the find method within these frameworks can improve overall application performance and user experience. In a React application, for instance, if you have a list of tasks being rendered, and you want to find a specific task by its id, the following example could be very handy:
Here, the task with the specified id is easily found, allowing further actions like displaying task details or performing updates.
The find method doesn't just improve efficiency; it allows for cleaner and more maintainable code, especially in larger applications where data retrieval becomes cumbersome.
Frameworks like Vue also show great synergy with the find method, particularly in managing state. For example, when implementing Vuex for state management, locating a particular item within the store can be seamlessly accomplished. In these settings, the find method complements the reactive nature of frameworks, making it easier to manage real-time updates without tedious code.
Finale
The conclusion serves as a vital part of this article, synthesizing key insights about the 'find' method in JavaScript. This method has proved to be an essential array manipulation tool that simplifies searching for specific elements in arrays. Through a detailed exploration, we have seen how its syntax, parameters, and practical applications work in harmony to meet programming needs.
Recap of Key Points
At the core, the 'find' method operates on arrays, returning the value of the first matching element based on a specified condition. Here are the significant takeaways:
- Functionality: The 'find' method enables efficient searching in arrays without the need for complex loops or manual iterations.
- Syntax: Understanding the syntax is crucial. The method accepts a callback function that defines the condition for finding elements that match specific criteria.
- Use Cases: This method is particularly useful for locating objects within arrays and validating user inputs, making it invaluable in real-world applications.
- Comparison: Differentiating 'find' from other array methods such as 'filter' and 'some' uncovers its unique advantages, especially in scenarios requiring only the first match.
- Performance Considerations: Being aware of the time complexity and best practices can further optimize the use of 'find', particularly in larger datasets.
Future of Array Methods in JavaScript
As JavaScript evolves, so does its array manipulation capabilities. Developers can expect enhancements in performance and functionality. The future may bring new array methods that build upon the existing framework, extending capabilities further.
Furthermore, combining the 'find' method with modern frameworks is likely to become increasingly seamless. Expect better integration with asynchronous operations, improving performance when dealing with data streams or API calls. The community's focus on efficiency and usability will continue driving innovations in array handling.
In essence, as JavaScript grows, methods like 'find' will remain pivotal, adapting to the needs of developers and the challenges of modern coding environments.