Mastering Inheritance in Python: A Detailed Guide
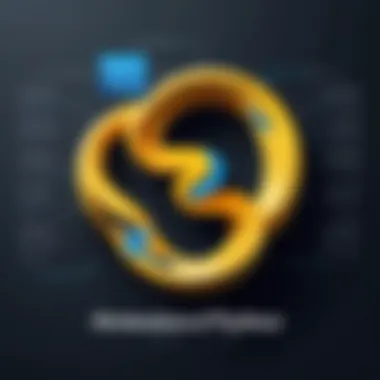
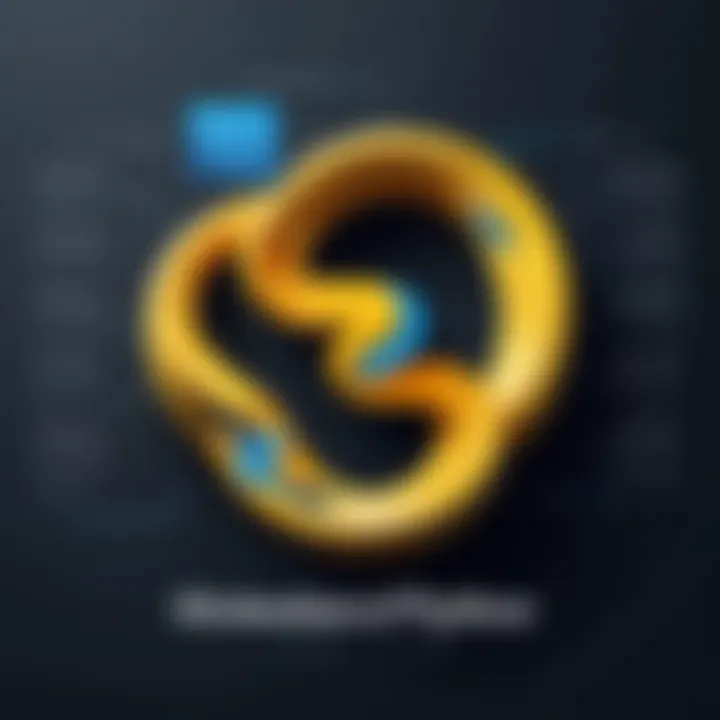
Intro
Inheritance in Python can be viewed as one of the cornerstones of object-oriented programming. It allows developers to create new classes based on existing ones, promoting code reusability and enhancing the structure of their applications. By building on established behavior, inheritance allows programmers to save time while creating more understandable code that can be easily maintained.
In this guide, we will take a thorough look at the different types of inheritance available in Python, specifically single, multiple, multilevel, and hierarchical inheritance. Each serves its own purpose and comes with a set of benefits that can drastically improve the way a programmer approaches a project. From newcomers trying to find their footing to seasoned veterans looking to refine their craft, this guide aims to offer something for everyone.
Understanding inheritance is not just seeing how to use it; it is comprehending why it matters. With the right grasp, one can streamline complex operations and make the code not just functional, but elegant as well.
This article will also include practical examples that translate the concepts into real-world applications. We'll examine the advantages that come with using inheritance, coupled with the potential pitfalls that developers should remain wary of. By the end, readers should feel confident in their ability to utilize inheritance to build scalable and efficient code. While we wonât just skim the surface, weâll dive into meaningful details that lay the groundwork for a solid understanding of inheritance in Python.
Preface to Inheritance in Python
Inheritance is a powerful concept that lies at the very heart of object-oriented programming in Python. It allows developers to create a new class based on an existing class, inheriting its attributes and behaviors while introducing new functionalities. This capability not only streamlines coding practices but also promotes cleaner, more efficient, and organized code.
In this part of the article, we'll delve into the definition of inheritance and its significance in the world of programming. Understanding inheritance is essential for anyone looking to optimize their code structure and leverage the full power of Python.
Definition of Inheritance
At its core, inheritance is a mechanism in Python that enables one class, referred to as the derived or child class, to inherit properties and methods from another class, known as the base or parent class. This relationship fosters a hierarchical organization of classes, simplifying the management of shared code.
For example, consider a base class called `Animal`. This class has attributes like `species` and methods like `make_sound()`. A derived class, `Dog`, can inherit these properties from `Animal` but also have specific attributes like `breed` and methods such as `fetch()`. Hereâs a brief code snippet that illustrates this concept:
This example highlights how `Dog` inherits from `Animal`, allowing reuse of code and adding its unique features. The core idea here is that inheritance fosters a natural extension of functionality.
Importance of Inheritance
The significance of inheritance extends beyond mere code reuse. It fundamentally shapes how developers approach problem-solving in programming. Here are some key reasons why inheritance is important:
- Code Reusability: By reusing existing code, inheritance reduces redundancy. Instead of rewriting similar functionalities for different classes, developers can simply extend existing ones.
- Organized Structure: Inheritance helps in organizing related classes and their functionalities logically. This makes the code easier to understand and maintain, akin to a family tree where each member shares traits.
- Ease of Maintenance: When a base class is modified, all derived classes gain these updates automatically. This means less effort is needed to manage changes in the codebase.
- Polymorphism: Inheritance supports polymorphism. This principle allows methods in derived classes to override those in base classes, providing flexible and dynamic method calling based on the object type.
- Enhanced Collaboration in Development: It allows teams to work on different classes concurrently without stepping on each otherâs toes, as long as they follow the established base class protocols.
Inheritance is not just a technique; it's a design philosophy that empowers programmers to think creatively and systematically.
In summary, grasping the definition and importance of inheritance is foundational to mastering Pythonâs object-oriented capabilities. As we proceed through this article, weâll explore various types and implementations of inheritance, equipping you with the tools needed to effectively utilize this powerful feature.
Fundamental Concepts of Object-Oriented Programming
Understanding the fundamental concepts of object-oriented programming (OOP) is crucial for grasping inheritance in Python. OOP serves as a blueprint for building complex software in a more maintainable and scalable way. Think of it like assembling a jigsaw puzzle; each piece represents a class or object, and how you put these pieces together determines the final image of your application. This section focuses on two core elements: classes and objects, as well as the paradigms of encapsulation, polymorphism, and abstraction.
Classes and Objects
Classes and objects are the bedrock of OOP. A class can be likened to a blueprint; it defines the properties and behaviors that its objects will have. For instance, if you consider a class named , it might have attributes like , , and , and methods like and .
An object, on the other hand, is an instance of a class. If is the blueprint, then a with a specific color like is an object derived from that blueprint. This relationship allows for a clear structure in your code:
- Encapsulation: By wrapping properties and methods within classes, you limit access to specific functionalities and data. Itâs like putting your valuables in a safe; only authorized individuals can access them.
- Reusability: Once a class is created, you can produce multiple objects from it without redefining the same properties. Itâs an efficient way to keep your code DRY (Donât Repeat Yourself), letting you focus on building out further functionality instead of redundant code.
In Python, defining a class is straightforward:
In this example, is a class with the constructor , which initializes its attributes. You can create an object from this class like so:
Encapsulation, Polymorphism, and Abstraction
Encapsulation, polymorphism, and abstraction are pillars that support the OOP paradigm, enhancing how we interact with programs through greater flexibility and simplicity.
- Encapsulation: As mentioned earlier, this concept bundles data and methods within one unit or class, limiting external access to them. In Python, private variables can be created using underscore prefixes (e.g., ). Think of it as a wrapper around a gift; you canât see what's inside until the wrapper is removed, ensuring that unintended interactions are minimized. For instance:
class BankAccount: def init(self, balance): self._balance = balance
def deposit(self, amount): self._balance += amount
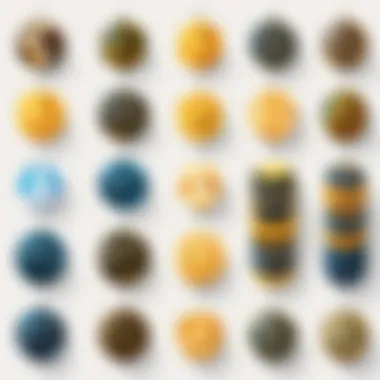
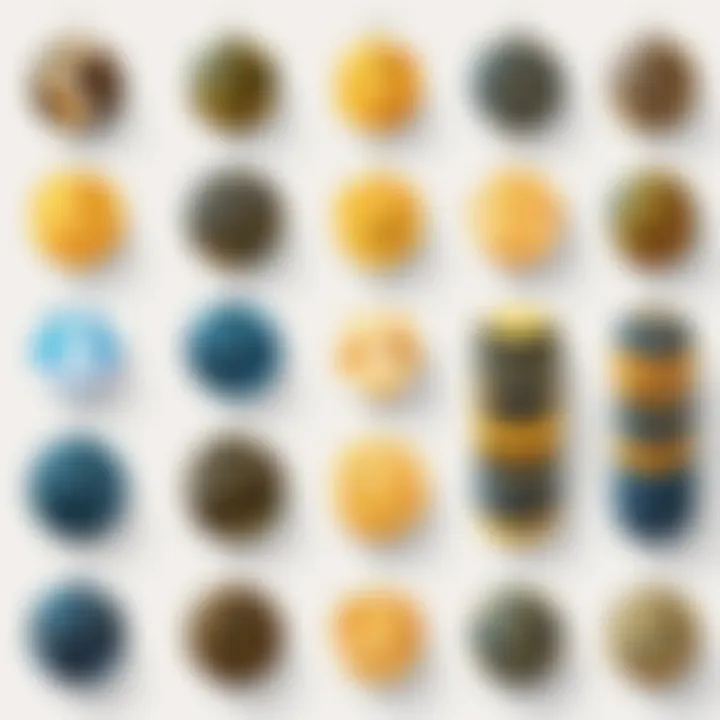
- Abstraction: This principle helps simplify complex reality; it hides unnecessary details and exposes only the relevant parts. Just like when you drive a car, you donât need to know how the engine works under the hood. In Python, this can be achieved through abstract base classes (ABCs) which provide a skeletal structure to define methods that must be created within any child classes. Use them when you want to enforce certain methods on derived classes:
from abc import ABC, abstractmethod
class Animal(ABC): @abstractmethod def sound(self): pass
class Dog(Animal): def sound(self): return 'Bark'
Here, the class inherits from the class. This pattern offers a clear way to build upon existing functionalities while keeping the structure organized.
Multiple Inheritance
In Python, multiple inheritance allows a class to inherit from more than one parent class. This can increase the expressiveness of your code, but itâs not without its challenges.
Consider this example:
Here, the class is able to utilize methods from both and . However, caution is needed to avoid method resolution order (MRO) issues, particularly in complex structures.
Multilevel Inheritance
This form of inheritance involves a hierarchy of classes where a derived class can inherit from another derived class.
For example:
inherits from , which in turn inherits from . This brings about a hierarchy where each level can add its functionalities, fostering a clearer code organization.
Hierarchical Inheritance
In this type, multiple subclasses inherit from a single base class, which can enhance code maintainability.
Take a look at:
Both and inherit from , showcasing how this method can efficiently organize related classes while minimizing repetitive code.
Hybrid Inheritance
Hybrid inheritance combines two or more types of inheritance methods. Itâs particularly handy for creating complex relationships among classes, but it also demands careful implementation to sidestep confusion in inheritance chains.
For illustration:
Here, class inherits from both and , which in turn come from . This wide-reaching relationship could introduce complications with method overriding and resolve preferences if not carefully managed.
Understanding the various types of inheritance in Python is vital for any programmer keen on enhancing their coding chops. It shapes how software is structured, and knowing when and how to use each type can lead to more efficient and organized code.
Implementing Inheritance in Python
Implementing inheritance in Python is a cornerstone of effective object-oriented programming. This feature not only facilitates code reusability, but also enables a structured approach to coding that minimizes redundancy. By allowing developers to define base classes that encapsulate common behavior and attributes, it becomes easier to build more specialized classes without starting from scratch. Furthermore, inheritance promotes better organization of code, making it simpler to understand and maintain.
Defining Base and Derived Classes
Base classes provide the foundation, while derived classes extend that foundation with added complexity and functionality. For instance, consider a base class that defines general properties common to all animals, such as and . A derived class, say , can then inherit these properties and add its own specific attributes like or .
In this code snippet, is a derived class that extends the base class. It inherits the and properties. The function ensures that the base class's method is called, allowing streamlined initialization. This way, developers can avoid unnecessary code duplication, conserving time and reducing errors.
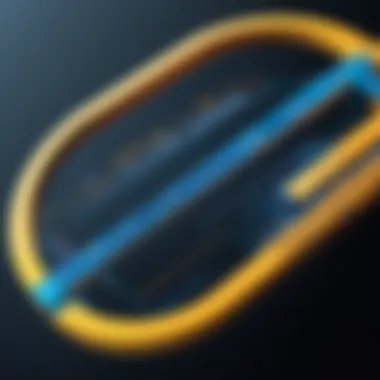
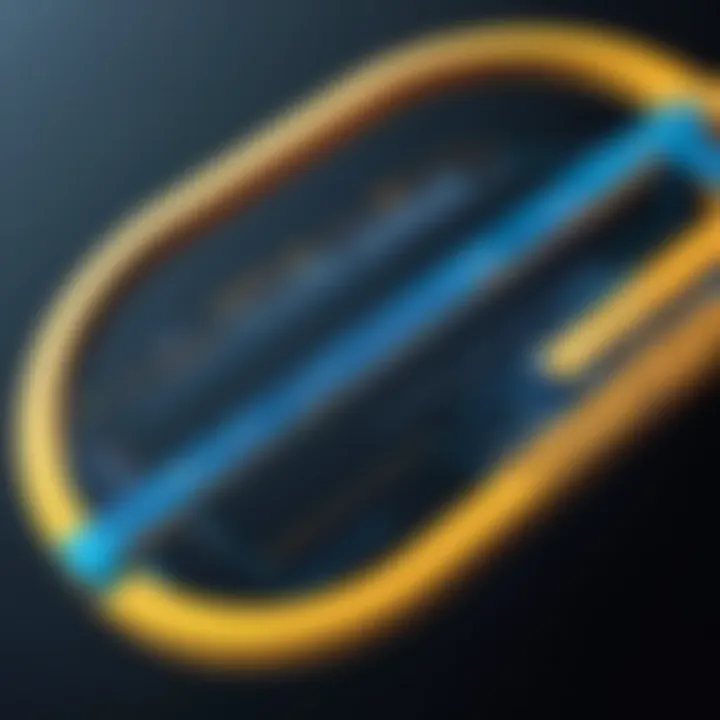
Inheritance is not just a feature; it's a guiding philosophy in object-oriented programming that promotes efficient code.
Using the Super() Function
The function is crucial in the inheritance model. Its primary role is to give access to methods and properties of a base class from within a derived class. This function smoothens the process of calling base class methods, especially when there are multiple inheritance layers. When used, it makes your code cleaner and more maintainable.
Consider the following example:
In this case, inherits from . The function calls the method of , which allows to initialize its and attributes without repeating code. This function not only enhances code clarity but also supports collaboration between multiple classes and layers.
Implementing inheritance effectively can significantly enhance both the scalability and readability of your code. As such, understanding how to define base and derived classes alongside utilizing functions like is essential for any Python programmer.
Advantages of Using Inheritance
Inheritance in Python is more than just a fancy programming feature; it's the mortar that holds the bricks of structured code together. This principle allows developers to write code that stands the test of time. Let's explore how inheriting properties and methods from parent classes can be a game-changer for thoses pursuing efficiency and clarity in their Python projects.
Code Reusability and Maintenance
One of the standout benefits of using inheritance is undoubtedly code reusability. Instead of rewriting code for similar classes, you can create a base classâoften termed a parent or superclassâand then derive other classes from it, making adjustments as needed. This approach not only reduces redundancy but also fosters a cleaner codebase.
For example, if youâre building a software application that handles different types of user accounts, you might have a class that stores shared attributes like username and password. Then, classes such as and can inherit from , and you only need to write the common code once.
Additionally, maintaining code becomes easier due to centralization. If a bug emerges or enhancement is needed, you can fix it in one placeâthe base classâand the changes propagate automatically to all derived classes. This drastically cuts down the risk of overlooking some aspect of the codeâan outcome that can often lead to a cascade of errors. In summary:
- Reduced code duplication
- Easier updates and maintenance
- Enhanced clarity of program structure
Logical Grouping of Classes
Another advantage of inheritance is its ability to promote logical grouping of classes. Through this hierarchical structure, developers can organize classes in a way that mirrors real-world relationships, making the code more intuitive. For instance, consider an application for a zoo management system. You can have a base class called , with specific derived classes like , , or .
This logical classification not only improves readability but also showcases how certain features can be generalized, while still allowing for specialized behavior.
"A well-structured codebase is a joy to work with, saving time and confusion down the line."
When structuring classes in this way, Pythonâs inheritance capabilities shine through; they allow for clear expectations regarding shared behavior. Developers can easily understand that any , for instance, will have certain attributes like or behavior like , while will inherit traits such as and .
In essence, this clear categorization leads to:
- Enhanced code organization
- Easier collaboration among developers
- Increased understanding of the software architecture
Common Challenges with Inheritance
Inheritance, while a robust aspect of Python's object-oriented programming, isn't without its pitfalls. As programmers delve deeper into utilizing inheritance, they may encounter various challenges that can complicate designs and lead to unforeseen consequences. Understanding these challenges can help in crafting more effective class hierarchies, ensuring a smoother coding experience.
Among the several issues, two notable ones often arise: the diamond problem in multiple inheritance and the overriding of methods. Tackling these issues is vital, as they can influence the overall maintainability and functionality of your code. Having a strong grasp of these challenges allows developers to use inheritance effectively and avoid common misconceptions.
Diamond Problem in Multiple Inheritance
The diamond problem, at its core, presents a unique scenario where a class inherits from two classes that share a common base class. Let's consider this with a simple analogy. Imagine a family tree where a child inherits traits from both parents, but those parents inherit from the same grandparent. This overlapping can lead to confusion about which inherited features should take precedence.
In Python, when dealing with multiple inheritance, the method resolution order (MRO) comes into play. Python uses a specific algorithm, known as C3 Linearization, to determine how classes are organized regarding method resolution. So, if you have:
In this example, inherits from both and , which in turn both derive from . This brings to light that when is called on an object of , Python gives preference to 's method due to its position in the MRO. One must be educated about these inheritance rules, as they might impact the predictability of code.
Understanding the diamond problem and MRO is crucial to navigate the complexities of inheritance in Python.
Overriding Methods
Overriding methods might seem straightforward at first glance, but it can conjure some sticky situations, particularly when not done carefully. In the context of inheritance, overriding is when a derived class provides its own implementation of a method that is already defined in its base class. This can be completely beneficial but also risky if not managed attentively.
When you override a method, itâs important to remember the principle of least surpriseâyour inherited methods should act as expected unless there's a compelling reason for behavior to shift. A common mishap involves calling the base class method from the derived class after overriding, which can occasionally lead to unexpected results or errors if not handled correctly.
For instance:
Here, the keyword ensures that the base class's method is called before the derived class's implementation. This practice can be beneficial for applying shared behaviors while still adding enhancements exclusive to the subclass. However, a misalignment in expectations can confuse both the developer and future maintainers of the code.
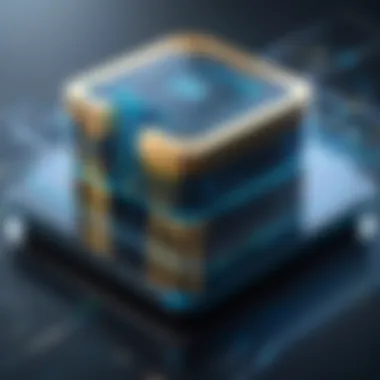
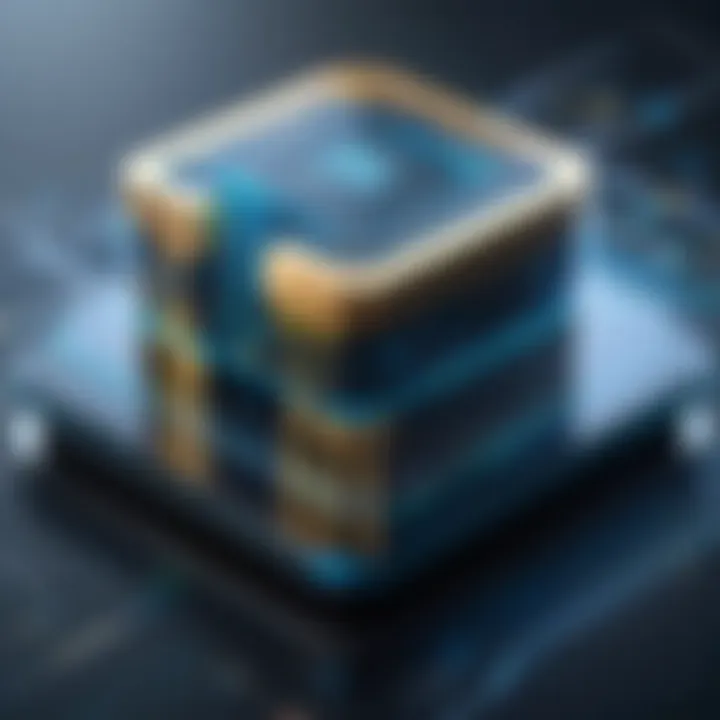
In summary, while inheritance can enhance code reusability and maintainability, it is crucial to be aware of these common challenges. By understanding the nuances of the diamond problem and the implications of method overriding, developers can navigate these issues with increased confidence and skill.
Practical Examples of Inheritance in Python
Inheritance in Python isn't just a theoretical topic; it's a pragmatic tool that allows developers to create efficient and organized code. Practical examples help cement understanding by demonstrating real-world applications of inheritance principles. These examples serve to illustrate not only the mechanics of inheritance but also its advantages in improving code maintainability and scalability. Through concrete scenarios, we can better grasp how to effectively harness inheritance in our projects, thus making our code more intuitive and streamlined.
Case Study: Animal Class Hierarchy
The Animal class hierarchy provides an excellent case study to understand how inheritance can be structured in Python. Consider an application aimed at creating a simulation of various animal species. Here, a base class named can encapsulate shared attributes and methods, such as , , and . This foundational class can then be extended into derived classes such as , , and , each showcasing their unique behaviors while inheriting properties from .
Here's how it might look in code:
In this setup, the and classes inherit the properties of the class but also override the method to provide their specific implementations. This demonstrates code reusability while allowing for flexibility in behaviors. It's a win-win situation that saves time and keeps the codebase clean.
Case Study: Vehicle Class Structure
Another insightful example of inheritance is the structuring of a vehicle class. In this scenario, a base class can be designed to encapsulate general properties like , , and . The derived classes, such as , , and , can then introduce additional characteristics pertinent to those specific types of vehicles.
Consider the following implementation:
This approach not only encapsulates vehicle details but also allows for class-specific data to be added without altering the base class system. Extending functionality becomes straightforward, enabling easier future modifications. Each class can have methods and properties that reflect their own characteristics while still benefiting from the general vehicle properties.
Inheritance streamlines code structures and enhances readability, pushing developers towards more elegant solutions than ever before.
Best Practices for Inheritance
Inheritance, while a powerful feature of Python, comes with its own set of guidelines that can make or break your code's flexibility and maintainability. Embracing best practices not only ensures smoother development but also enhances code clarity and reduces the likelihood of bugs.
When to Use Inheritance
Knowing when to utilize inheritance is as critical as the act itself. Here are some indicators that suggest inheritance might be the right choice:
- Related Classes: If two or more classes share a significant amount of behavior or attributes, consider creating a base class. For instance, an class can serve as a parent for both and , allowing shared methods for pay calculations.
- Code Reuse: Inheritance shines when it comes to reducing code duplication. If you find yourself writing the same methods across multiple classes, it's time to rethink your design. Using inheritance can help streamline common functionality, making your codebase easier to manage.
- Extensibility: If you anticipate adding new similar classes in the future, starting with a base class can set a solid foundation for extending features. This way, youâll keep your system open for future growth without rewriting existing code.
However, tread carefully. Not every relationship warrants inheritance. Instead, sometimes compositionâa design principle where one class contains instances of anotherâmay serve better. Always keep the object-oriented principle of âis-aâ versus âhas-aâ in mind.
Avoiding Over-Complexity
Complexity can creep into your design like weeds in a garden. To prevent your class hierarchy from becoming a tangled mess, consider the following practices:
- Limit the Depth of Inheritance: Deep inheritance trees can lead to tightly coupled code that is challenging to understand and maintain. Ideally, a chain of three to four levels should suffice. The deeper it goes, the harder it gets to track the flow of data and functionality.
- Be Cautious with Multiple Inheritance: While powerful, multiple inheritance should be used judiciously. The famous âDiamond Problem,â where a class inherits from two classes that share a common ancestor, can lead to ambiguity in method resolution. Evaluate whether the benefits of using multiple inheritance outweigh the potential issues.
- Favor Composition Over Inheritance: Often, mixing classes together through composition can yield clearer structures. Instead of making one class inherit from another, consider creating objects that collaborate. For example, a might have an instead of extending it.
âSimplicity is the ultimate sophistication.â - Leonardo da Vinci
By keeping a close eye on complexity, you not only improve readability but also pave the way for easier troubleshooting and enhancements.
Finale
In wrapping up our discussion on inheritance in Python, itâs crucial to recognize its importance within the wider framework of programming. Inheritance not only simplifies code development but also establishes a logical structure that makes managing complex systems more feasible. Without inheritance, programmers would face a daunting task, constantly reinventing the wheel for every new feature or module.
Employing inheritance offers significant benefits:
- Code Reusability: It allows programmers to leverage existing code, reducing redundancy and streamlining development.
- Organization: By structuring related classes through inheritance, it encourages logical grouping and easier understanding of functionality.
- Maintainability: Updates to base classes automatically propagate to derived classes, saving time and reducing the risk of errors.
However, programmers should exercise caution. While inheritance promotes efficient coding practices, overuse can lead to convoluted hierarchies that are difficult to manage. Itâs essential to weigh the benefits against the potential for increased complexity.
"With great power comes great responsibility." This saying holds water in the realm of inheritance. It is a powerful tool, but mismanagement can lead to a tangled mess of interdependencies and behaviors that can confound even seasoned developers.
Summary of Key Points
To summarize, we have delved into the following key aspects throughout this article:
- Definition and Importance: Inheritance is a foundational principle in object-oriented programming that enhances code efficiency and structure.
- Types of Inheritance: Each type, from single to hybrid inheritance, serves unique purposes and offers different mechanisms for extending functionality.
- Implementation: Knowing how to define base and derived classes is critical, and utilizing the function efficiently can improve access to parent class methods.
- Challenges: Specific complexities, like the diamond problem and method overriding, need careful consideration in implementation.
- Best Practices: Understanding when to use inheritance is key to avoiding unnecessary complications.
This overview underscores the multiple dimensions of inheritance in Python, reinforcing its significance in software development.
Future of Inheritance in Python Development
As we look toward future Python development, inheritance will undoubtedly continue to play a pivotal role. Given the rapid evolution of software architecture, the necessity for modular, maintainable code will only increase. We can expect the following trends to shape how inheritance is utilized:
- Integration with Other Paradigms: As programming paradigms develop, inheritance will need to interact seamlessly with concepts like functional programming, which may lead to hybrid approaches in designing classes and modules.
- Shift Toward Composition: While inheritance is powerful, thereâs a growing trend toward using composition over inheritance. Developers might prefer combining simpler classes instead of relying on deep inheritance hierarchies, a shift that recognizes the limitations of inheritance.
- Tooling and Frameworks: The emergence of robust frameworks could also redefine inheritance use. Tools that encourage best practices and mitigate complications will likely enhance how developers interact with inheritance in their projects.