Mastering Exit Strategies in Python For Loops
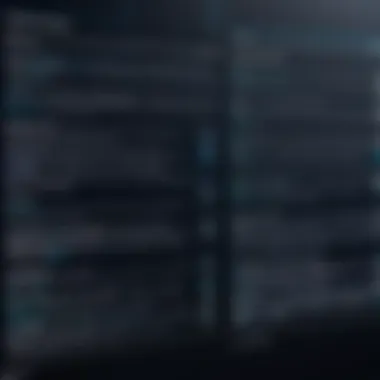
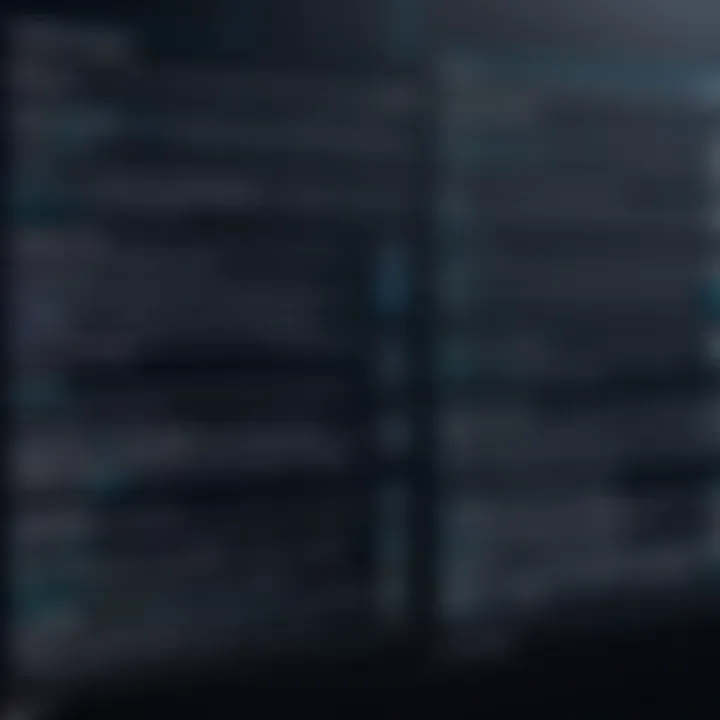
Intro
In programming, understanding how control structures like loops function is essential. For loops in Python are designed for iterating over complex data structures. However, there are times when one may need to exit these loops prematurely. This involves utilizing built-in statements such as and , or exploring more sophisticated practices with exceptions and generator functions. This guide is meant to anchor tech enthusiasts and industry professionals in the knowledge necessary to navigate and employ these techniques effectively.
Tech Trend Analysis
Overview of the current trend
The use of loops in programming is gaining increased attention, especially as data handling becomes more complex. Many developers are focusing on optimizing their loops to improve performance and readability. As such, mastery of exiting for loops has become a crucial skill among computer programmers and software engineers.
Implications for consumers
When developers use loops effectively, applications can run faster and with less CPU consumption. This increased potential results in smoother user experiences, more responsive designs, and reduced wait times for consumers, leading to enhanced satisfaction.
Future predictions and possibilities
As technology evolves, programming languages like Python will likely introduce more advanced mechanisms for managing loops. Future developments may include enhanced inline documentation for and , potentially simplifying learning curve for beginners. Furthermore, as artificial intelligence grows, loop efficiency will become increasingly crucial in optimizing data processing.
Fundamental Loop Control Mechanisms
Break Statement
The statement provides a straightforward method to exit a loop entirely when a certain condition is satisfied. The fundamental syntax looks like this:
When the statement executes, the loop is terminated immediately, and the control flow is transferred to the statement following the loop.
Continue Statement
In contrast, allows for skipping the current iteration and proceeding with the next. Using this can be useful for filtering elements without needing to revise entire structure:
This statement makes the code cleaner and more efficient, ensuring unnecessary iterations do not consume resources.
Advanced Techniques for Exiting Loops
In more complex scenarios, you might need to rely on exceptions to exit loops. Using exceptions introduces an elegant way to manage flow under unexpected circumstances.
Using Exceptions
You can define an exception and raise it when a specific condition occurs in the loop:
This method allows quitting loops cleanly and managing situations without cluttering the main logic with numerous break statements.
Leveraging Generator Functions
Python’s generator functions allow for code that “yields” values rather than returning them all at once. It's possible to exit such loops naturally based on yielding conditions:
This results in less memory consumption and improved performance over standard loops when dealing with extensive datasets.
Mastery of exiting for loops is not just benefitcial for cleaner code but also fosters resource optimization.
Ending
Exiting for loops effectively is a critical programming skill that allows for greater control over code execution. With a deep understanding of the fundamentals and advanced techniques covered in this guide, readers are well-equipped to enhance their coding practices in Python. Staying informed about innovations in loop management will continue to be valuable as programming technologies evolve.
Intro to For Loops in Python
For loops are fundamental constructs in Python programming. They enable the repettion of code for elements within a sequence, such as lists, tuples, or even strings. This capability is essential for many programming tasks, allowing for efficient and scalable code structure. The simplicity of for loops makes them accessible, but the true mastery comes with understanding their nuances.
The exploration into exiting for loops provides invaluable insights for developers. Working with loops often entails determining how and when to exit them properly. Proper management of loops directly affects performance and readability of the code. This section sets the ground for further discussion on the mechanics of controlling loop behavior.
Understanding Loop Constructs
In Python, loops are classified into various constructs. The most commonly used varieties are the for and while loops. Each type serves its own purposes but understanding their foundational behaviors serves also to achieve optimal use.
The for loop in particular operates by iterating over iterables, functionally accessing each element in a given sequence. This method is trademarked by its clarity and the reduction of code complexity. However, developers must also be cautious about the complexities introduced when trying to exit loops mid-execution.
Syntax of For Loops
The syntax of a for loop in Python allows flexibility for different operations on sequence elements.
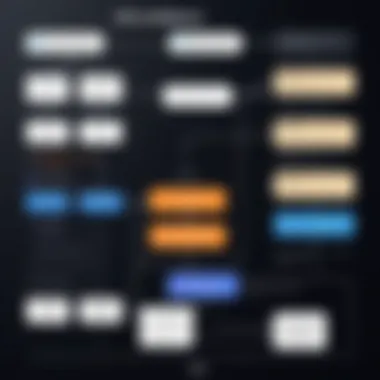
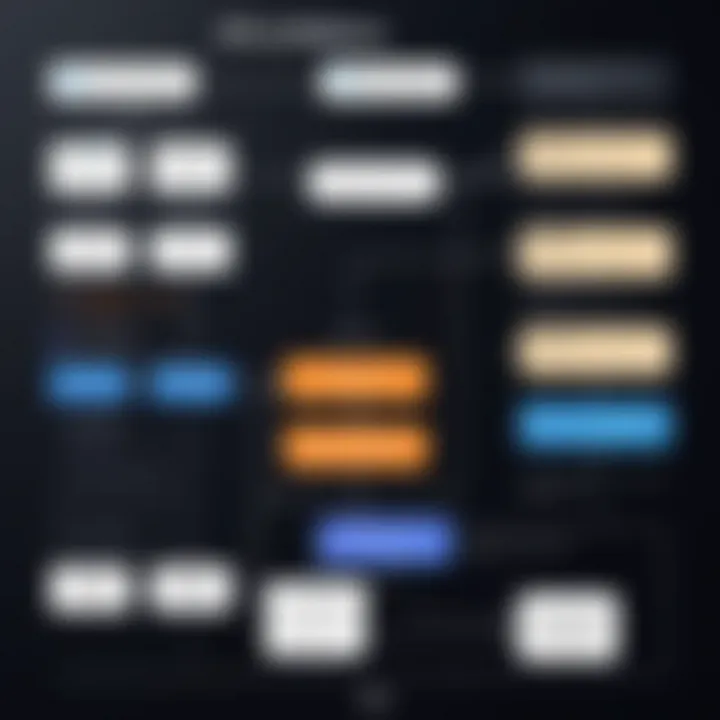
Here, the denotes any Python iterable—such as a list or string—and as iterations occur, assumes the value of each component in that iterable. The indentation under the for statement is vital; it determines the block of code associated with the loop. Exiting from this loop correctly can transform otherwise intricate code into neat and comprehensible lines. Knowing how to implement control statements to prematurely exit a loop becomes a necessity as projects grow in complexity and scope.
Basic Loop Control Statements
In the realm of Python programming, understanding loop control statements is essential for writing efficient code. These statements serve as tools that enable precise control over the execution flow of loops. For enthusiasts and professionals alike, mastering these elements ensures that code runs as intended without unnecessary iterations or stoppages.
The most notable of these loop control statements in Python are the break and continue statements. Each serves distinct purposes but both are pivotal in managing how and when loops terminate or proceed.
The Break Statement
The break statement deliberately halts a loop's execution. It serves as a useful mechanism during search operations or any situation where a particular condition signifies the need to exit the loop. For example, if searching for a specific value in a data set, employing a break condition not only conserves computing resources but clears up complexity in larger structures.
Example of Break Statement:
This code will print numbers 1 to 4, and stop when it reaches 5. The known condition allows for early exits, optimizing runtime.
Benefits of Using Break:
- Resource Efficiency: By stopping unnecessary processing, it can significantly reduce resource consumption, especially in lengthy loops.
- Improved Clarity: Developers can express clearer intent, making code easier to understand.
The use of the break statement should be considered carefully. Over-using it might lead to premature termination of loops. Thus, a balance is crucial.
The Continue Statement
In contrast with break, the continue statement allows a loop to skip the current iteration but continue running. It instructs the code to bypass certain levels of logic under predefined conditions, letting the remaining iterations process as normal. This is especially handy in data filtering scenarios where certain criteria must be ignored but others executed.
Example of Continue Statement:
This snippet will output numbers 1, 2, 4, and 5. Number 3 is excluded, whether it is causing issues in earlier computations or simply is not desired in outputs.
Benefits of Using Continue:
- Flexibility: It allows for fine-grained control, focusing only on iterations that meet determined conditions.
- Usage Simplification: Some loops can become complex without it, making code cleaner and intuitive.
While the continue statement can be beneficial in avoiding unwanted processes, excessive use can result in obfuscation of capabilities and lead to confusion in understanding loop behavior.
Advanced Techniques for Exiting Loops
In this section, we take an in-depth look at the advanced techniques available for exiting loops in Python. Understanding these techniques is crucial for writing efficient and responsive code. While the basic control statements like break and continue serve their purpose, we must examine scenarios where more intricate motives are required. Exceptions and generator functions provide distinct pathways for exiting loops, leading to clearer code and achieving specific logic requirements. Examining how and when to use these techniques enhances not only performance but also maintainability.
Using Exceptions to Exit Loops
Leveraging exceptions to exit loops brings significant benefits. Often, normal control flow can be disrupted unexpectedly. This is where exceptions prove useful. By intending to handle outlier cases gracefully, one can keep the main logic clean and focused. Exceptions do not only terminate loops but can also be utilized to convey precise information about what went wrong.
Example of Using Exceptions
A common situation could be found in reading files. When processing data line-by-line, if a line has an unexpected not been formatted correctly, raising an error simply allows the loop to exit while maintaining awareness about the error’s context.
Studying this example shows that while loops designed for iterations process successfully, clarity in the contextual exit makes the internal flow much easier. Importantly, one can catch these tailored exceptions elsewhere in cast by using an orderly error management fresh worth considering while in broader constructs.
Leveraging Generator Functions
Another technique involves using generator functions to manage iterations more effectively. Generator functions maintain their state, which allows loop exit customizations. They yield values incrementally instead of generating them all at once. It is clever when handling larger datasets or complex calculations, saving memory and resources efficiently. Yielding control allows breaking out of loops lighthearted, making it easier, adaptable.
How Generators Can Help
When a generator function generates items in a sequence, if a specific condition is flagged, the loop can be exited safely. For instance, using a generator for a sequence to find prime numbers can allow exiting without processing unnecessary calculations after achieving the first required amount.
This example demonstrates how to use a generator function to produce prime numbers, only terminating once specified limits have been met. It improves efficiency, and encapsulates the prime number logic better available without prematurely embodying disparate items before requirements defined.
Ultimately, employing advanced techniques such as exceptions and generator functions for exiting loops enables effective control over computer behavior. When correctly used, they lead to cleaner code, better resource management, and consequently, proffer overall performance with increased robustness that* underpins substantial technical solutions.
Performance Considerations
Performance considerations are crucial when writing code, especially in programming languages like Python where efficiency can impact the overall performance of applications. Understanding these elements is essential to write optimal code and make the best choices with loop control. Choosing the right way to exit a loop not only affects readability but also alters execution time and resource consumption.
Efficiency of Break and Continue
The and statements significantly influence the efficiency of loops in Python. Utilizing to exit a loop can save time in scenarios where continuing is futile. For instance, if a search operation finds the desired element, using immediately terminates the loop, preventing further unnecessary iterations. This enhances performance, particularly with larger datasets, where looping through each element can cause slowdowns.
When to Use Break
- Use when an exit condition is met sooner than the loop completing.
- It minimizes time complexity, especially with nested loops.
- Helps maintain code clarity when exiting loops on certain conditions.
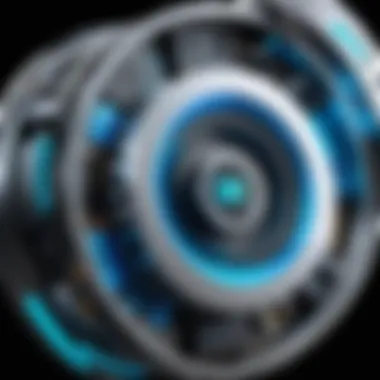
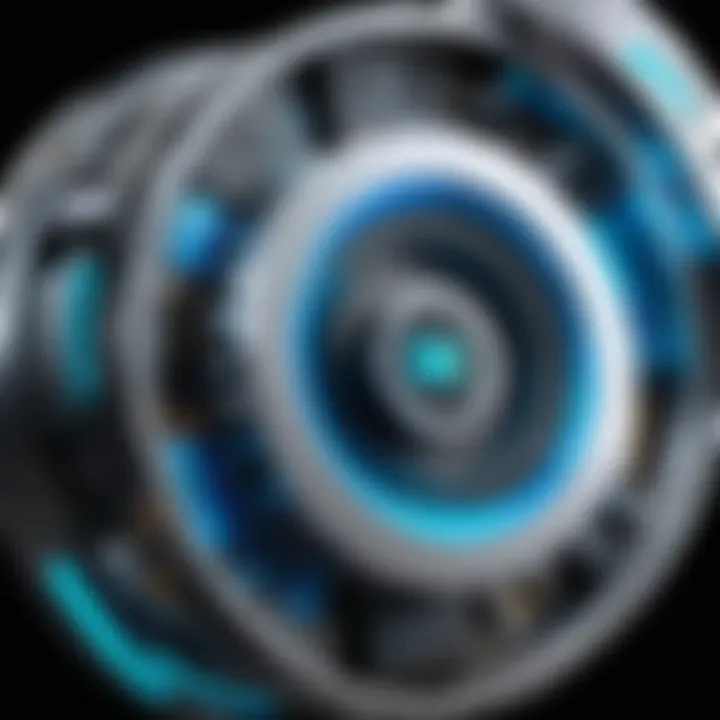
The statement alters the loop's flow by skipping to the next iteration. This skill can be useful in filtering data. However, careless use may degrade performance. Multiple calls to in a long loop can expand computational time if not implemented pragmatically. Always witness the balance between loop logic and efficiency, ensuring that is genuinely optimizing performance.
Impact of Exceptions on Performance
Using exceptions to exit a loop can be helpful but also brings considerations of its impact on performance. Exception handling in Python can be resource-intensive and should be utilized sapiently. Each raised exception can result in a stack trace that consumes memory. Therefore, while strategies such as utilizing could be useful for exiting loops upon error conditions, overusing them may introduce noticeably larger overhead.
Key Points to Note:
- Use exceptions for exceptional scenarios and not for regular control flow.
- Evaluate the trade-offs of exception handling against more straightforward logic like or
- Pay attention to profiling your code to identify bottlenecks effectively.
In sum, understanding performance considerations with exiting for loops can optimize programs, reduce execution time, and ultimately lead to improved user experiences. The efficient use of control statements enhances code behavioral richness, making applications responsive and quick.
Practical Examples and Use Cases
Understanding practical examples and use cases for exiting for loops in Python is essential for making effective decisions while coding. These examples illustrate how to apply theoretical concepts in real-world scenarios. Specifically, they showcase the utility of and statements in managing loop execution in different contexts.
The importance lies in the ability to develop efficient algorithms and avoid errors related to unexpected behavior. Real-world programming often entails dealing with large datasets or continuous streams of input, situations where a program might not need to process every element. Thus, effectively controlling loop behavior enhances both performance and clarity.
Consideration of these practical items allows programmers to better streamline their code.
Using Break in Search Algorithms
The statement is particularly useful in search algorithms when one wants to terminate a loop as soon as a condition is met. For example, finding a specific element in a list can be performed more efficiently by using . When you find the target item, there is no need to continue checking subsequent elements.
Imagine a simple implementation where you search for a particular number in a list:
In this case, once the number 30 is found, there is no benefit in continuing the loop. It stops immediately, saving unnecessary processing time. The construct thus creates more readable code and facilitates a prompt exit from the loop once the goal is achieved.
Real-world applications include scenarios where performance is critical, such as game development, database searching, or even data analysis tasks where specific conditions can halt progress for simplicity and speed.
Utilizing Continue in Data Filtering
The statement provides another control flow mechanism. It allows the loop to skip certain iterations without breaking out entirely. This is particularly useful in data filtering scenarios where some data entries do not meet criteria but you want to process others.
For example, if you want to run validations on user inputs but skip any entries that are invalid:
In this snippet, any empty or None entry will be ignored by the loop. Skipping to the next iteration allows the processing of valid inputs only. Programs that process large datasets, like logs or user data, benefit significantly from this structure.
Ultimately, applying and statements correctly supports cleaner and more efficient code. Implementing these techniques not only maintains clarity but also enhances the performance of Python applications.
Common Mistakes and Their Solutions
Understanding the common mistakes related to exiting for loops is crucial for anyone working with Python. These common errors often lead to inefficient code or unexpected behavior during execution. By recognizing and addressing these mistakes, developers can streamline their coding process and avoid frustrating debugging sessions. This section will detail two significant pitfalls: accidental infinite loops and the misuse of loop control statements. Unpacking these errors provides insights into improving coding practices while empowering programmers to write more efficient and predictable code.
Accidental Infinite Loops
Infinite loops occur when the conditions for terminating a loop are never met. This situation arises mostly due to oversight in the logic of the loop's construction. For instance, if the condition controlling a for loop depends on variables that never update or change within the loop's body, you end up running the loop forever.
Here is an example that demonstrates an accidental infinite loop:
In this instance, the code will run as designed, but similar constructs with improper conditions can lead potentially anywhere from unexpected behavior to program hang-ups. Activities like forgetting to increment counters or modifying the wrong variable are frequently responsible. Using proper logic before deploying loop constructs prevents this frustrating situation.
To recognize trailing loops:
- Always check loop conditions before
- Verify control logic’s flow of execution
- Review how values change during each iteration
When caught in an infinite loop, employing Ctrl+C can cease execution, though it's better to avoid needing that. Addressing probable pitfalls in loop conditions preemptively leads to more efficient coding practices.
Misuse of Loop Control Statements
Using control statements like break and continue correctly is essential to effective loop management. Beginners often face challenges when just starting with Python. Over-reliance on these control statements can lead to logic errors or less readable code.
Choices made concerning whether to break from a loop too hastily can cause premature termination, disrupting expected outcomes entirely. Conversely, misusing continue may skip necessary iterations, thus affecting code results adversely.
Examples of misuse include:
- Break Statement Misuse: Breaking a loop too soon might be likened to ceasing travel between destinations midway—once while searching for a value.
- Continue Statement Misuse: Utilizing continue with hampers that might not check values as expected, affecting the loop's integrity.
Operating properly by approaching these keywords judiciously is critical. When scripting:
- Ensure break or continue affects structures meaningfully.
- Monitor how often they appear in your segments.
- Reflect considerations to either apply an if clause or embed less such controls altogether.
Acknowledging these misuse examples is fundamental in developing sound practices, ensuring code reliability while enhancing outcomes.
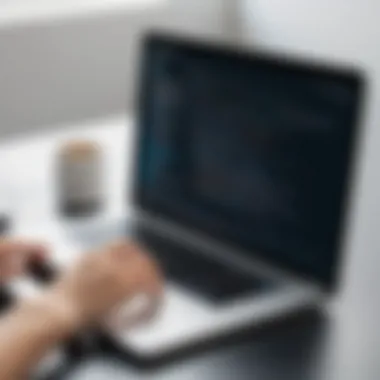
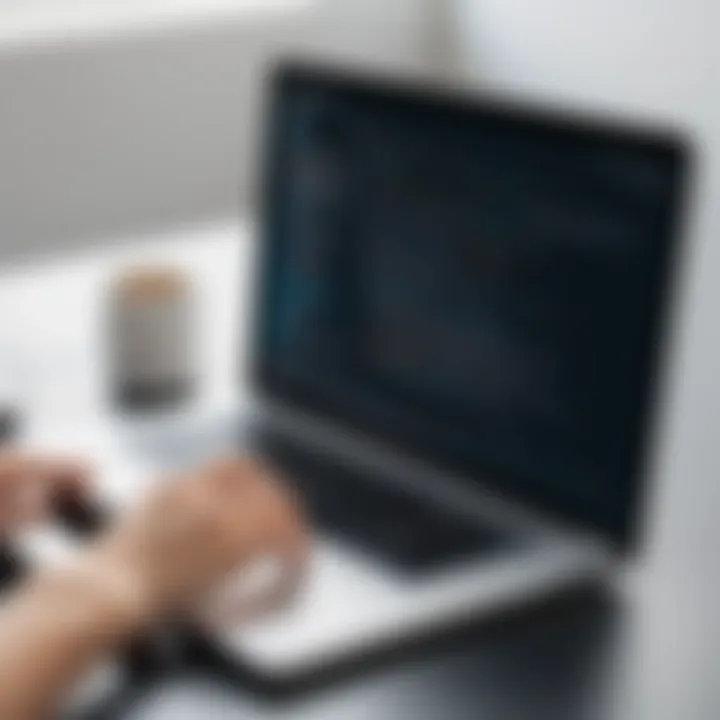
“Crafting code with precision, notably when forming loops, can prevent a majority of common errors relating to control statements.”
By mitigating mistakes such as infinite loops and control statement misuse, programmers fortify not just their scripting endeavors but also arrive at cleaner, maintainable, and overall optimized code design. Investigating, adapting best practices churns potential obstacles into learning moments, further improving coding aptitude.
Best Practices for Writing Loop Statements
Writing loop statements in Python efficiently is foundational for good programming practice. With every for loop, there are underlying principles that can help maintain readability, enhance performance, and simplify debugging processes. In this section, we will explore the significance of adhering to best practices when writing loop statements.
Firstly, best practices help to avoid common pitfalls. Inefficient loops can decrease performance and create complications in larger applications. When loops are readable, it allows for easier maintenance and modifications to the code. Employing sound looping principles can prevent mistakes that might lead to infinite loops or convoluted logic. By focusing on clarity, one fosters collaboration and ensures that other programmers can engage with the code without significant barriers.
Keeping Code Readable
Readable code enhances ease of understanding. Writing for loops that express their intent clearly is critical. Here are a few practical tips to maintain code clarity:
- Descriptive variable names: Choose variable names that reflect their purpose. Instead of , using something like clearly informs the reader.
- Limit loop nesting: Avoid creating multiple loops within loops where possible. It makes the logic complex. Structure the code to maintain a flat hierarchy.
- Consistent formatting: Maintain uniformity in your indentation style and spacing, allowing the loops to blend seamlessly. A well-formatted block visually directs readers quickly.
Examples of readable code:
In this case, the loop clearly iterates through a collection of and prints their names, making the code readily understandable.
Emphasize that readability should never be sacrificed for brevity.
Optimizing Loop Conditions
Loop conditions are integral to the efficiency of a loop's execution. Optimizing how loops are structured can drastically improve a program's performance.
Consider the following aspects to optimize loop conditions:
- Use when requiring indices: If you need the index of items in an iterable, use the function. This eliminates the need for manually maintaining an index integer—even minor savings in processor cycles amounts, especially in large datasets.
- Pre-compute loop bounds: When possible, calculate bounds outside the loop. This reduces repeated calculations and keeps checks minimal during execution.
- Short-circuit conditions: In conditional statements within loops, structure them sequentially from most likely to least likely scenarios. This practice reduces execution time by taking advantage of the way boolean evaluations work in Python.
Properly optimized conditions yield not only a decrease in computational time but facilitate a lighter load when interpreting instruction paths, therefore keeping workflows optimized.
By observing these best practices, programmers can effectively enhance their loops’ readability and performance, which supports clearer and more efficient code bases. Remember that clean, well-structured code will benefit everyone who engages with it, including future you.
Comparative Analysis with Other Languages
Understanding how for loops function across various programming languages enriches one's knowledge and broadens their perspective on loop control mechanisms. This section highlights comparison between Python's approach with that of JavaScript and C++. Such analysis illuminates strengths and weaknesses, providing insights that are mainly applicable when selecting the right tool for specific use cases.
For Loop Constructs in JavaScript
JavaScript’s for loop demonstrates a more classic structure. The syntax is simple yet powerful.
Several critical elements exist here. Initialization often involves declaring a loop variable. The condition propels the loop, while the increment focuses on updating the loop variable. It's essential to note that if the condition evaluates to , the loop halts, similar to Python's concept of a termination condition. Programs often use , and for controlling flow within these loops.
Advanced JavaScript concepts, such as callbacks and promises, enable asynchronous operations within loops but often complicate behavior. Hence, a contrary impact is notable when comparing these loops to Python's more straightforward syntax regarding asynchronous processing, which is less common in the Python for loop paradigm. Thus, it benefits developers to weigh their approach against their specific needs surrounding simplicity and asynchronous execution.
For Loop Mechanisms in ++
C++ uses a familiar structure although slight differences set it apart. The for loop in C++ looks like this:
C++ draws attention to type declarations. In this instance, the type of loop variable must be explicitly stated. Loop performance here can also significantly vary through fine-tuning compiler settings and language features. Unlike Python, which relies heavily on dynamic typing, C++ demands stronger control over types and memory, making it appealing for performance-critical applications.
A feature of loops in C++ that stands out is the use of break and continue statements, paralleling Python’s constructs. Moreover, C++ incorporated range-based for loops since C++11. This addition simplifies iteration over collections effectively, providing readability closer to Python options, especially showcasing iterators:
>
Understanding these frameworks allows developers to sharpen their coding skills and tailor solutions based on programming language behavior.
Overall, analyzing loops across different programming languages reveals not only syntactic differences but also styles that suit varying intercultural programming paradigms. The transition between other languages can be made smoother by internalizing these comparisons, and adopting the most pertinent methodologies will ultimately yield better results.
The End
The conclusion holds significant weight in this article. It serves not only as a summary but also as a crucial element to distill the essential knowledge gained regarding exiting for loops in Python. Each programmer, regardless of their proficiency, needs to recognize when and how to effectively control loop behavior. A sound understanding of break and continue statements, along with how to employ exceptions and generators, allows for greater code efficiency and readability.
Recap of Key Points
To reinforce the insights covered, let’s recap the key points touched upon across this article:
- For Loop Construction: The basic syntax and structural elements offer a foundational base for readers.
- Loop Control Statements: Understanding the purposes of break and continue is vital for managing execution flow.
- Advanced Techniques: Exceptional control methods provide alternative ways to exit loops, enhancing overall flexibility in coding practices.
- Performance Considerations: Analyzing the trade-offs linked with break, continue statements, and exceptions are essential for improving efficiency.
- Practical Examples: Offering real-world scenarios gives context to the functionality of loop control statements in Python.
- Common Mistakes: Identifying frequent pitfalls helps programmers avoid common errors that could lead to unexpected behavior.
- Best Practices: Establishing clear writing and optimal conditions aids in maintaining quality code.
- Comparative Analysis: Understanding differences in for loop constructs across other languages broadens a developer’s perspective.
Future Considerations for Loop Control
As programming continues to evolve, future considerations for loop control in Python could focus on potential enhancements in language constructs and tools. Optimizing how loops interact with asynchronous programming and data handling can yield significant improvements in performance. Also, integrating modern practices such as parallel processing may reshape the traditional use of loops entirely.
Maintaining awareness of Python enhancement proposals (PEPs) will be crucial. Keeping abreast of community discussions will aid developers in adapting their methodologies successfully. In an ever-changing landscape, continuous improvement, learning, and adaptation are key health indications of successful coding practices.
Programming is not just writing code; it evolvs over time through practices and understanding deeper functionality.
By embracing these future trends and challenges, programmers can ensure that their skills remain sharp, relevant, and valuable in the competitive tech industry.